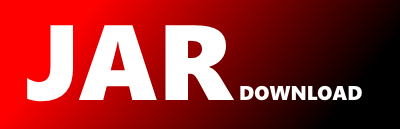
ma.vi.base.reflect.State Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.vikmad.base Show documentation
Show all versions of com.vikmad.base Show documentation
Base algos, data structures and utilities
The newest version!
/*
* Copyright (c) 2018 Vikash Madhow
*/
package ma.vi.base.reflect;
import ma.vi.base.lang.NotFoundException;
import ma.vi.base.tuple.T2;
import java.util.Optional;
/**
* Reflectively read and write an object state.
*
* @author Vikash Madhow ([email protected])
*/
public class State {
public static Object get(Object object, String path) {
T2
© 2015 - 2025 Weber Informatics LLC | Privacy Policy