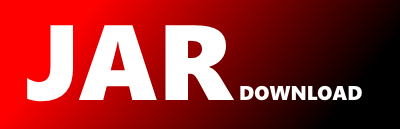
ma.vi.base.tuple.Tuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.vikmad.base Show documentation
Show all versions of com.vikmad.base Show documentation
Base algos, data structures and utilities
The newest version!
/*
* Copyright (c) 2016 Vikash Madhow
*/
package ma.vi.base.tuple;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
/**
* Interface of all tuples.
*
* @author Vikash Madhow ([email protected])
*/
public interface Tuple extends Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy