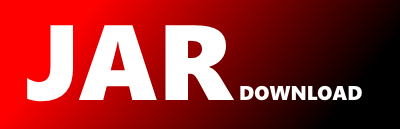
src.main.java.com.vincomobile.fw.basic.tools.Converter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.tools;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.i18n.phonenumbers.NumberParseException;
import com.google.i18n.phonenumbers.PhoneNumberUtil;
import com.google.i18n.phonenumbers.Phonenumber;
import org.apache.commons.codec.binary.Base64;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESKeySpec;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Time;
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Date: 22-may-2005
* Time: 14:32:05
*/
public class Converter {
private static Logger logger = LoggerFactory.getLogger(Converter.class);
private static Random randomGenerator = new Random();
public static final String HASH_MD5 = "MD5";
public static final String HASH_SHA1 = "SHA-1";
public static final String HASH_SHA2 = "SHA-2";
static DecimalFormatSymbols dfs = new DecimalFormatSymbols();
static DecimalFormat df = new DecimalFormat();
/**
* Check float value
*
* @param value Value to check
* @return If valid float
*/
private static boolean checkF(String value) {
try {
Float.parseFloat(value);
return true;
}
catch (Exception e) {
return false;
}
}
public static boolean validFloat(String value, boolean nullOk) {
return (nullOk && isEmpty(value)) || (!isEmpty(value) && (checkF(value.replace(',', '.')) || checkF(value.replace('.', ','))));
}
/*
* Convierte una cadena a real
*/
/**
* Convert string to float
*
* @param value Value to convert
* @return Float value
*/
public static double getFloat(String value) {
return getFloat(value, 0.0);
}
public static double getF(String value, Double defVal) {
try {
return Float.parseFloat(value);
}
catch (Exception e) {
return defVal;
}
}
public static double getF(String value) {
try {
return Float.parseFloat(value);
}
catch (Exception e) {
return Float.NaN;
}
}
public static double getFloat(String value, Double defVal) {
if (value == null)
return defVal;
double res = getF(value.replace(',', '.'));
if (Double.isNaN(res))
res = getF(value.replace('.', ','));
return Double.isNaN(res) ? defVal : res;
}
public static double getFloatPrec(String value, int prec) {
return getFloatPrec(getFloat(value, 0.0), prec);
}
public static double getFloatPrec(double value, int prec) {
double mult = Math.pow(10, prec);
value = value * mult;
double tmp = Math.round(value);
return tmp / mult;
}
/**
* Format a double to string
*
* @param value Float value
* @return Formatted number
*/
public static String formatFloat(double value) {
return formatFloat(value, 2);
}
public static String formatFloat(double value, int digit) {
dfs.setDecimalSeparator(',');
df.setGroupingUsed(false);
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(digit);
df.setMinimumFractionDigits(digit);
return df.format(value);
}
public static String formatFloat(double value, int digit, boolean grouping) {
dfs.setDecimalSeparator(',');
df.setGroupingUsed(grouping);
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(digit);
df.setMinimumFractionDigits(digit);
return df.format(value);
}
public static String formatFloat(double value, int digit, boolean grouping, char separator) {
dfs.setDecimalSeparator(separator);
df.setGroupingUsed(grouping);
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(digit);
df.setMinimumFractionDigits(digit);
return df.format(value);
}
public static float formatFloatToReport(float num){
return Math.round(num*100)/100f;
}
public static double formatDoubleToReport(double num){
return Math.round(num*100)/100d;
}
/**
* Convert float value to SQL
*
* @param value Float value
* @return Float SQL formatted
*/
public static String getSQLFloat(double value) {
dfs.setDecimalSeparator('.');
df.setGroupingUsed(false);
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(2);
df.setMinimumFractionDigits(6);
return df.format(value);
}
public static String getSQLFloat(double value, int min, int max) {
dfs.setDecimalSeparator('.');
df.setGroupingUsed(false);
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(min);
df.setMinimumFractionDigits(max);
return df.format(value);
}
public static String getFloat(double value, int dec) {
df.setDecimalFormatSymbols(dfs);
df.setMaximumFractionDigits(dec);
df.setMinimumFractionDigits(dec);
return df.format(value);
}
public static String getSQLDouble(Double value) {
return value == null ? "null" : getSQLFloat(value);
}
/**
* Validate integer value
*
* @param value Value
* @param nullOk If null is valid
* @return If is valid integer
*/
public static boolean validInt(String value, boolean nullOk) {
if (nullOk && isEmpty(value))
return true;
try {
Integer.parseInt(value);
return true;
}
catch (Exception e) {
return false;
}
}
/**
* Convert string to integer
*
* @param value Value
* @return Integer
*/
public static int getInt(String value) {
return getInt(value, 0);
}
public static int getInt(Integer value) {
if (value == null)
return 0;
else
return value;
}
public static int getInt(String value, Integer defVal) {
try {
return Integer.parseInt(value);
}
catch (Exception e) {
return defVal;
}
}
/**
* Convert string to long
*
* @param value Value
* @return Long
*/
public static long getLong(String value) {
return getLong(value, 0l);
}
public static long getLong(Long value) {
if (value == null)
return 0;
else
return value;
}
public static long getLong(String value, Long defVal) {
try {
return Long.parseLong(value);
}
catch (Exception e) {
return defVal;
}
}
/**
* Convert string to boolean
*
* @param value String (1, S, Y, true, Si, Sí, Yes, On)
* @param defVal Default value
* @return Boolean
*/
public static boolean getBoolean(String value, boolean defVal) {
if (value == null)
return defVal;
else {
value = value.toUpperCase();
return "Y".equals(value) || "1".equals(value) || "1.0".equals(value) || "S".equals(value) || "TRUE".equals(value) || "SI".equals(value) || "SÍ".equals(value) || "YES".equals(value) || "ON".equals(value);
}
}
public static boolean getBoolean(String value) {
return getBoolean(value, false);
}
public static String getBooleanStr(boolean value) {
return value ? "S" : "N";
}
public static boolean getBoolean(Boolean value, boolean defVal) {
return value == null ? defVal : value;
}
/**
* Check time format
*
* @param time Time value
* @return If is valid
*/
public static boolean validTime(String time) {
if (!time.equals("")) {
SimpleDateFormat dFormat = new SimpleDateFormat("HH:mm");
ParsePosition pos = new ParsePosition(0);
return dFormat.parse(time, pos) != null;
}
return true;
}
/**
* Format time
*
* @param time Time value
* @return Formatted time
*/
public static String getTimeStr(Date time) {
if (time != null) {
SimpleDateFormat dFormat = new SimpleDateFormat("HH:mm");
return dFormat.format(time);
}
return null;
}
/**
* Check valid date
*
* @param date Date value
* @return If valid date
*/
public static boolean validDate(String date) {
return validDate(date, "dd/MM/yyyy");
}
public static boolean validDate(String date, String format) {
if (!date.equals("")) {
SimpleDateFormat dFormat = new SimpleDateFormat(format);
ParsePosition pos = new ParsePosition(0);
return dFormat.parse(date, pos) != null;
}
return true;
}
/**
* Convert a Strig in Date
*
* @param date Date string
* @param format Format of string
* @return Date
*/
public static Date getDate(String date, String format) {
if (date != null && !date.equals("")) {
SimpleDateFormat dFormat = new SimpleDateFormat(format, Locale.ENGLISH);
ParsePosition pos = new ParsePosition(0);
return dFormat.parse(date, pos);
}
return null;
}
public static Date getDate(String date) {
Date result = getDate(date, "dd/MM/yyyy");
if (result == null)
result = getDate(date, "dd/MM/yyyy HH:mm:ss");
if (result == null)
result = getDate(date, "yyyy-MM-dd HH:mm:ss");
if (result == null)
result = getDate(date, "yyyy-MM-dd'T'HH:mm:ss");
if (result == null)
result = getDate(date, "dd/MM/yyyy'T'HH:mm:ss");
return result;
}
/**
* Convert a String to Time
*
* @param time Time string
* @param format Format
* @return Time
*/
public static Time getTime(String time, String format) {
if (time != null && !time.equals("")) {
SimpleDateFormat dFormat = new SimpleDateFormat(format);
ParsePosition pos = new ParsePosition(0);
return new Time(dFormat.parse(time, pos).getTime());
}
return null;
}
public static Time getTime(String time) {
return getTime(time, "HH:mm");
}
/**
* Convert a Date in SQL String
*
* @param date Date to convert
* @param format Format
* @param setSep Set or not a "'"
* @return SQL Date
*/
public static String getSQLDate(Date date, String format, boolean setSep) {
if (date == null)
return "null";
SimpleDateFormat dFormat = new SimpleDateFormat(format);
if (setSep)
return "'"+dFormat.format(date)+"'";
else
return dFormat.format(date);
}
public static String getSQLDate(Date date) {
return getSQLDate(date, true);
}
public static String getSQLDate(Date date, boolean setSep) {
return getSQLDate(date, Constants.SQL_DATE_OUTPUT_FORMAT, setSep);
}
/**
* Convert Date to SQL timestamp
*
* @param date Date
* @param format Format
* @param setSep Set or not a "'"
* @return SQL Timestamp
*/
public static String getSQLDateTime(Date date, String format, boolean setSep) {
if (date == null)
return "null";
SimpleDateFormat dFormat = new SimpleDateFormat(format);
if (setSep)
return "'"+dFormat.format(date)+"'";
else
return dFormat.format(date);
}
public static String getSQLDateTime(Date date, boolean setSep) {
return getSQLDateTime(date, Constants.SQL_DATETIME_OUTPUT_FORMAT, setSep);
}
/**
* Convert a Date to String
*
* @param date Date
* @param format Format
* @return String
*/
public static String formatDate(Date date, String format) {
if (date == null)
return "";
SimpleDateFormat dFormat = new SimpleDateFormat(format);
return dFormat.format(date);
}
public static String formatDate(Date date) {
return formatDate(date, "dd/MM/yyyy");
}
/**
* Get a SQL to avoid code injection
*
* @param str String
* @param sep Include sql separator ('str')
* @return Secure SQL value
*/
public static String getSQLString(String str, boolean sep) {
if (str == null)
return "null";
else {
str = str.replace("'", "''");
str = str.replace("\\", "\\\\");
if (sep)
return "'"+str+"'";
else
return str;
}
}
public static String getSQLString(String str) {
return getSQLString(str, true);
}
/**
* Convert a boolean to SQL bit
*
* @param bool Value
* @return SQL value
*/
public static String getSQLBoolean(boolean bool) {
return bool ? "1" : "0";
}
/**
* Convert a long value to SQL
*
* @param value Long value
* @return SQL value
*/
public static String getSQLLong(Long value) {
return value == null ? "null" : value.toString();
}
public static String getSQLLong(String value) {
return value == null ? "null" : Long.toString(getLong(value));
}
public static String getString(String str) {
return getString(str, "");
}
public static String getString(String str, String def) {
if (str == null)
return def;
else
return str;
}
/**
* Verify is object is empty
*
* @param value Value to check
* @return Is empty or not
*/
public static boolean isEmpty(String value) {
return value == null || value.equals("");
}
public static boolean isEmpty(Long value) {
return value == null || value == 0;
}
public static boolean isEmpty(Integer value) {
return value == null || value == 0;
}
public static boolean isEmpty(Date value) {
return value == null;
}
public static boolean isEmpty(Double value) {
return value == null || value == 0;
}
public static boolean isEmpty(List value) {
return value == null || value.isEmpty();
}
/**
* Get a value from ResultSet
*
* @param rs ResultSet
* @param name Field name
* @return Value
* @throws SQLException
*/
public static String getStringFromResultSet(ResultSet rs, String name) throws SQLException {
String value = rs.getString(name);
if (value != null)
value = value.trim();
return value;
}
/**
* Convert to string the StackTrace
*
* @param aThrowable Excepcion
* @return StackTrace string
*/
public static String getStackTrace(Throwable aThrowable) {
final Writer result = new StringWriter();
final PrintWriter printWriter = new PrintWriter(result);
aThrowable.printStackTrace(printWriter);
return result.toString();
}
/**
* Verify if IP is valid
*
* @param ip IP Address (xxx.xxx.xxx.xxx)
* @return Valid or not
*/
public static boolean validIP(String ip) {
String octetos[] = ip.trim().split("\\.");
try {
int uno = Integer.parseInt(octetos[0]);
int dos = Integer.parseInt(octetos[1]);
int tres = Integer.parseInt(octetos[2]);
int cuatro = Integer.parseInt(octetos[3]);
return uno >= 0 && uno < 256 && dos >= 0 && dos < 256 && tres >= 0 && tres < 256 && cuatro >= 0 && cuatro < 256;
}
catch (Exception e) {
return false;
}
}
/**
* Return int value of IP addresss
*
* @param addr IP Address (xxx.xxx.xxx.xxx)
* @return Int value
*/
public static long ipToInt(String addr) {
try {
String[] addrArray = addr.split("\\.");
long num = 0;
for (int i=0;i> 24) & 0xFF) + "." +
((ip >> 16) & 0xFF) + "." +
((ip >> 8) & 0xFF) + "." +
( ip & 0xFF);
}
/**
* Check is valid Java identifier
*
* @param value Value to be check
* @return Valid or not
*/
public static boolean isValidIdentifier(String value) {
// An empty or null string cannot be a valid identifier
if (value == null || value.length() == 0) {
return false;
}
char[] c = value.toCharArray();
if (!Character.isJavaIdentifierStart(c[0])) {
return false;
}
for (int i = 1; i < c.length; i++) {
if (!Character.isJavaIdentifierPart(c[i])) {
return false;
}
}
return true;
}
/**
* Fill string from left
*
* @param val String to complete
* @param fill Character to fill
* @param len Total length of generate string
* @return Filled string
*/
public static String leftFill(String val, char fill, int len) {
int i = val.length();
StringBuffer result = new StringBuffer();
while (i < len) {
result.append(fill);
i++;
}
result.append(val);
return result.toString();
}
public static String leftFill(int val, char fill, int len) {
return leftFill(Integer.toString(val), fill, len);
}
public static String leftFill(long val, char fill, int len) {
return leftFill(Long.toString(val), fill, len);
}
/**
* Fill string from right
*
* @param val String to complete
* @param fill Character to fill
* @param len Total length of generate string
* @return Filled string
*/
public static String rightFill(String val, char fill, int len) {
int i = val.length();
StringBuffer result = new StringBuffer();
result.append(val);
while (i < len) {
result.append(fill);
i++;
}
return result.toString();
}
public static String rightFill(int val, char fill, int len) {
return rightFill(Integer.toString(val), fill, len);
}
public static String rightFill(long val, char fill, int len) {
return rightFill(Long.toString(val), fill, len);
}
/**
* Capitalize a string
*
* @param name Value
* @return Capitalize value
*/
public static String capitalize(String name) {
return name != null ? name.toUpperCase().substring(0, 1) + name.toLowerCase().substring(1) : null;
}
public static String capitalize2(String name) {
return name != null ? name.toUpperCase().substring(0, 1) + name.substring(1) : null;
}
public static String uncapitalize(String name) {
return name != null ? name.toLowerCase().substring(0, 1) + name.substring(1) : null;
}
public static String capitalizePhrase(String phrase) {
String[] words = phrase.split(" ");
String result = "";
for (String word : words) {
word = word.trim();
if (!word.equals("")) {
result += capitalize(word) + " ";
}
}
return result.trim();
}
/**
* Replace not allowed characters in XML
*
* @param val Value
* @return XML Value
*/
public static String getXMLString(String val) {
val = val.replaceAll("&", "&");
val = val.replaceAll("<", "<");
val = val.replaceAll(">", ">");
return val;
}
public static String toUpperCase(String value) {
return value != null ? value.toUpperCase() : null;
}
/**
* Remove accents
*
* @param val Value
* @return Value without accents
*/
public static String replaceTilde(String val) {
if (val == null)
return null;
// á é í ó ú
val = val.replaceAll("á", "a").replaceAll("é", "e").replaceAll("í", "i").replaceAll("ó", "o").replaceAll("ú", "u");
// Á É Í Ó Ú
val = val.replaceAll("Á", "A").replaceAll("É", "E").replaceAll("Í", "I").replaceAll("Ó", "O").replaceAll("Ú", "U");
// ñ Ñ ç Ç
val = val.replaceAll("ñ", "n").replaceAll("Ñ", "Ñ").replaceAll("ç", "Ç").replaceAll("Ç", "Ç");
// à è ì ò ù
val = val.replaceAll("à", "a").replaceAll("è", "e").replaceAll("ì", "i").replaceAll("ò", "o").replaceAll("ù", "u");
// À È Ì Ò Ù
val = val.replaceAll("À", "A").replaceAll("È", "E").replaceAll("Ì", "I").replaceAll("Ò", "O").replaceAll("Ù", "U");
// ä ë ï ö ü
val = val.replaceAll("ä", "a").replaceAll("ë", "e").replaceAll("ï", "i").replaceAll("ö", "o").replaceAll("ü", "u");
// Ä Ë Ï Ö Ü
val = val.replaceAll("Ä", "A").replaceAll("Ë", "E").replaceAll("Ï", "I").replaceAll("Ö", "O").replaceAll("Ü", "U");
return val;
}
/**
* Clear string for non-asii characters (accept only A-Z, a-z, 0-9, space, . , # -
*
* @param val String to clear
* @return Clean string (Character not allow are replaced by spaces)
*/
public static String clearString(String val) {
if (val == null)
return null;
String result = "";
for (int i = 0; i < val.length(); i++) {
char c = val.charAt(i);
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z') || (c >= '0' && c <= '9') || c == ' ' || c == '.' || c == ',' || c == '#' || c == '-')
result += c;
else
result += ' ';
}
return result;
}
/**
* Convert string to HTML
*
* @param val Value
* @return HTML
*/
public static String getHTMLString(String val) {
if (val != null) {
// < > "
val = val.replaceAll("<", "<").replaceAll(">", ">").replaceAll("&", "&").replaceAll("\"", """);
return getHTMLTilde(val);
} else
return " ";
}
public static String getHTMLTilde(String val, boolean replaceCR) {
if (val == null)
return " ";
// ¡ ¿ º ª €
val = val.replaceAll("¡", "¡").replaceAll("¿", "¿").replaceAll("º", "º").replaceAll("ª", "ª").replaceAll("€", "€");
// “ ” – © • °
val = val.replaceAll("“", "“").replaceAll("”", "”").replaceAll("–", "–").replaceAll("©", "©").replaceAll("•", "•").replaceAll("°", "°");
// « » ‘ ’
val = val.replaceAll("«", "«").replaceAll("»", "»").replaceAll("‘", "‘").replaceAll("’", "’");
// á é í ó ú
val = val.replaceAll("á", "á").replaceAll("é", "é").replaceAll("í", "í").replaceAll("ó", "ó").replaceAll("ú", "ú");
// Á É Í Ó Ú
val = val.replaceAll("Á", "Á").replaceAll("É", "É").replaceAll("Í", "Í").replaceAll("Ó", "Ó").replaceAll("Ú", "Ú");
// ñ Ñ ç Ç
val = val.replaceAll("ñ", "ñ").replaceAll("Ñ", "Ñ").replaceAll("ç", "Ç").replaceAll("Ç", "Ç");
// à è ì ò ù
val = val.replaceAll("à", "à").replaceAll("è", "è").replaceAll("ì", "ì").replaceAll("ò", "ò").replaceAll("ù", "ù");
// À È Ì Ò Ù
val = val.replaceAll("À", "À").replaceAll("È", "È").replaceAll("Ì", "Ì").replaceAll("Ò", "Ò").replaceAll("Ù", "Ù");
// â ê î ô û
val = val.replaceAll("â", "â").replaceAll("ê", "ê").replaceAll("î", "î").replaceAll("ô", "ô").replaceAll("û", "û");
// Â Ê Î Ô Û
val = val.replaceAll("Â", "Â").replaceAll("Ê", "Ê").replaceAll("Î", "Î").replaceAll("Ô", "Ô").replaceAll("Û", "Û");
// ä ë ï ö ü
val = val.replaceAll("ä", "ä").replaceAll("ë", "ë").replaceAll("ï", "ï").replaceAll("ö", "ö").replaceAll("ü", "ü");
// Ä Ë Ï Ö Ü
val = val.replaceAll("Ä", "Ä").replaceAll("Ë", "Ë").replaceAll("Ï", "Ï").replaceAll("Ö", "Ö").replaceAll("Ü", "Ü");
if (replaceCR)
val = val.replaceAll("\n", "
").replaceAll("\r", " ");
return val;
}
public static String getHTMLTilde(String val) {
return getHTMLTilde(val, true);
}
public static String getRemoveTilde(String val) {
// á é í ó ú
val = val.replaceAll("á", "a").replaceAll("é", "e").replaceAll("í", "i").replaceAll("ó", "o").replaceAll("ú", "u");
// Á É Í Ó Ú
val = val.replaceAll("Á", "A").replaceAll("É", "E").replaceAll("Í", "I").replaceAll("Ó", "O").replaceAll("Ú", "U");
// ñ Ñ ç Ç
val = val.replaceAll("ñ", "n").replaceAll("Ñ", "N").replaceAll("ç", "c").replaceAll("Ç", "C");
// à è ì ò ù
val = val.replaceAll("à", "a").replaceAll("è", "e").replaceAll("ì", "i").replaceAll("ò", "o").replaceAll("ù", "u");
// À È Ì Ò Ù
val = val.replaceAll("À", "A").replaceAll("È", "E").replaceAll("Ì", "I").replaceAll("Ò", "O").replaceAll("Ù", "U");
// ä ë ï ö ü
val = val.replaceAll("ä", "a").replaceAll("ë", "e").replaceAll("ï", "i").replaceAll("ö", "o").replaceAll("ü", "u");
// Ä Ë Ï Ö Ü
val = val.replaceAll("Ä", "A").replaceAll("Ë", "E").replaceAll("Ï", "I").replaceAll("Ö", "O").replaceAll("Ü", "U");
return val;
}
private static final String PATTERN_EMAIL = "[_a-zA-Z0-9\\-]+(\\.[_a-z0-9\\-]+)*(\\.*)\\@[_a-z0-9\\-]+(\\.[a-z]{1,4})+";
private static final String PATTERN_NAME = "[\\\"\\'>\\<]+";
private static final String EMAIL_PATTERN = "^[_A-Za-z0-9-\\+]+(\\.[_A-Za-z0-9-]+)*@[A-Za-z0-9-]+(\\.[A-Za-z0-9]+)*(\\.[A-Za-z]{2,})$";
/**
* From email address return the email
*
* @param email Email address: "First Name"
* @return Email: [email protected]
*/
public static String getEmailAddress(String email) {
Pattern pattern = Pattern.compile(PATTERN_EMAIL);
email = email.toLowerCase();
Matcher matcher = pattern.matcher(email);
matcher.find();
return email.substring(matcher.start(), matcher.end());
}
/**
* From email address return the name
*
* @param email Email address: "First Name"
* @return Name: First Name
*/
public static String getEmailName(String email) {
return email.replaceFirst(PATTERN_EMAIL, "").replaceAll(PATTERN_NAME, "");
}
/**
* Obtiene una direccion de email a partir de un nombre y una dirección
*
* @param name Nombre
* @param email Direccion
* @return name
*/
public static String getEmail(String name, String email) {
return name != null ? name + "<" + email +">": email;
}
/**
* Verifica si una direccion de Email es correcta
*
* @param email Direccion de email
* @return Indica si es correcta
*/
public static boolean validEmail(String email) {
if (isEmpty(email))
return false;
Pattern pattern = Pattern.compile(EMAIL_PATTERN);
Matcher m = pattern.matcher(email);
return m.matches();
}
/**
* Valida un NIF, CIF o NIE
* @param value Valor a verificar
* @return 1 = NIF ok, 2 = CIF ok, 3 = NIE ok, -1 = NIF error, -2 = CIF error, -3 = NIE error, 0 = ??? error
*/
public static int check_Nif_Cif_Nie(String value) {
String temp = value.toUpperCase();
String cadenadni="TRWAGMYFPDXBNJZSQVHLCKE";
if (!"".equals(temp)) {
//si no tiene un formato valido devuelve error
Pattern patternLetraNumerosAlgo = Pattern.compile("^[A-Z]{1}[0-9]{7}[A-Z0-9]{1}$");
Pattern patternT = Pattern.compile("^[T]{1}[A-Z0-9]{8}$");
Pattern patternNif = Pattern.compile("^[0-9]{8}[A-Z]{1}$");
if (patternLetraNumerosAlgo.matcher(temp).matches() || patternT.matcher(temp).matches() || patternNif.matcher(temp).matches()) {
//comprobacion de NIFs estandar
if (patternNif.matcher(temp).matches()) {
int posicion = Integer.parseInt(value.substring(0,8)) % 23;
char letra = cadenadni.charAt(posicion);
char letradni=temp.charAt(8);
return letra == letradni ? 1 : -1;
}
//algoritmo para comprobacion de codigos tipo CIF
int sumaPares = Integer.parseInt(value.substring(2, 3)) + Integer.parseInt(value.substring(4, 5)) + Integer.parseInt(value.substring(6, 7));
int sumaImpares = 0;
for (int i = 1; i < 8; i += 2) {
int digitoImpar = Integer.parseInt(value.substring(i, i+1));
int digitox2 = 2 * digitoImpar;
int sumaDigitos = digitox2 % 10;
if (digitox2 >= 10) {
sumaDigitos++;
}
sumaImpares += sumaDigitos;
}
int sumaTotal = sumaPares + sumaImpares;
int restoTotal = sumaTotal % 10;
int n = 10 - restoTotal; //en teoría si es cero no hay que restar
//comprobacion de NIFs especiales (se calculan como CIFs)
Pattern patternNifEsp = Pattern.compile("^[KLM]{1}.*");
if (patternNifEsp.matcher(temp).matches()) {
char cosa = (char)(64+n);
return (value.charAt(8) == cosa) ? 1 : -1;
}
//comprobacion de CIFs
Pattern patternCif = Pattern.compile("^[ABCDEFGHJNPQRSUVW]{1}.*");
if (patternCif.matcher(temp).matches()) {
char numeroFinalCorrecto = (n + "").charAt(0);
char letraFinalCorrecta = (char)(64+n);
char caracterAValidar = value.charAt(8);
return ((caracterAValidar == letraFinalCorrecta) || (caracterAValidar == numeroFinalCorrecto)) ? 2 : -2;
}
//comprobacion de NIEs
//T
Pattern patternNie = Pattern.compile("^[T]{1}.*");
if (patternNie.matcher(temp).matches()) {
Pattern patternRaro = Pattern.compile("^[T]{1}[A-Z0-9]{8}$");
boolean b = patternRaro.matcher(temp).matches();
char cosa = (b? (char)1: (char)0);
return (value.charAt(8) == cosa) ? 3 : -3;
}
//XYZ
Pattern patternXyz = Pattern.compile("^[XYZ]{1}.*");
if (patternXyz.matcher(temp).matches()) {
temp = temp.replace('X', '0').replace('Y', '1').replace('Z', '2');
String cosa = temp.substring(0, 8);
int pos = Integer.parseInt(cosa) % 23;
return (value.charAt(8) == cadenadni.charAt(pos)) ? 3 : -3;
}
}
return 0;
}
return 0;
}
/**
* Trunc a string
*
* @param text Text to trunc
* @param size Max length
* @param wordWrap When is true, word are not truncated
* @return Trunc text
*/
public static String truncText(String text, int size, boolean wordWrap) {
String result = text;
if (text != null && text.length() > size) {
if (wordWrap) {
int idx = size - 3;
while (result.charAt(idx--) != ' ' && idx > 0) {
}
if (idx > 0)
result = text.substring(0, idx+1) + " ...";
else
result = text.substring(0, size - 3) + " ...";
} else {
result = text.substring(0, size - 3) + "...";
}
}
return result;
}
public static String truncText(String text, int size) {
return truncText(text, size, false);
}
/**
* Convert a byte array to hexdecimal
*
* @param bytes bytes
* @return Hexadecimal representation
*/
public static String getHexString(byte[] bytes) {
StringBuffer hexString = new StringBuffer();
for (byte aByte : bytes) {
String hex = Integer.toHexString(0xff & aByte);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
/**
* Returns the integer value of a numeric object
*
* @param value Value to convert
* @return Integer value (Long)
*/
public static Long getLongValue(Object value) {
if (value == null)
return null;
if (value instanceof BigInteger)
return ((BigInteger) value).longValue();
if (value instanceof BigDecimal)
return ((BigDecimal) value).longValue();
if (value instanceof Integer)
return ((Integer) value).longValue();
if (value instanceof Long)
return ((Long) value);
return null;
}
public static Integer getIntegerValue(Object value) {
if (value == null)
return null;
if (value instanceof BigInteger)
return ((BigInteger) value).intValue();
if (value instanceof BigDecimal)
return ((BigDecimal) value).intValue();
if (value instanceof Integer)
return (Integer) value;
if (value instanceof Long)
return ((Long) value).intValue();
return null;
}
/**
* Eliminates underscores and converts Java conventions
*
* @param input Value to convert (id_item_product)
* @return Java-convention name (idItemProduct)
*/
public static String removeUnderscores(String input) {
if (input == null)
return null;
StringBuilder result = new StringBuilder();
String[] tmp = input.split("_");
for (int i = 0; i < tmp.length; i++) {
String fragment;
if (i == 0) {
fragment = tmp[i];
} else {
fragment = tmp[i].substring(0, 1).toUpperCase() + tmp[i].substring(1);
}
result.append(fragment);
}
return result.toString();
}
/**
* Resets underscore a string CamelCase
*
* @param input CamelCase String (idItemProduct)
* @return Urderscore String (id_Item_Product)
*/
public static String restoreUnderscores(String input) {
String regex = "([a-z])([A-Z])";
String replacement = "$1_$2";
return input.replaceAll(regex, replacement);
}
/**
* Get crytografic hash value
*
* @param method Method (MD4, MD5, SHA, SHA256)
* @param msg Message
* @return Hash vale for message
* @throws UnsupportedEncodingException
* @throws NoSuchAlgorithmException
*/
public static String getHashValue(String method, String msg) throws UnsupportedEncodingException, NoSuchAlgorithmException {
byte[] bytesOfMessage = msg.getBytes("UTF-8");
MessageDigest md = MessageDigest.getInstance(method);
byte[] thedigest = md.digest(bytesOfMessage);
return getHexString(thedigest);
}
public static String getHashValue2(String method, String msg) {
try {
return getHashValue(method, msg);
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
/**
* Encrypt plain text
*
* @param secret Secret phrase
* @param plainText Plain text
* @return Encryted text
* @throws Exception
*/
public static String encrypt(String secret, String plainText) throws Exception {
DESKeySpec keySpec = new DESKeySpec(secret.getBytes("UTF8"));
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey key = keyFactory.generateSecret(keySpec);
byte[] cleartext = plainText.getBytes("UTF8");
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] enc = cipher.doFinal(cleartext);
return new String(Base64.encodeBase64(enc));
}
/**
* Decrypt a text
*
* @param secret Secret phrase
* @param encryptedText Encryted text
* @return Plane text
* @throws Exception
*/
public static String decrypt(String secret, String encryptedText) throws Exception {
DESKeySpec keySpec = new DESKeySpec(secret.getBytes("UTF8"));
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey key = keyFactory.generateSecret(keySpec);
byte[] encrypedPwdBytes = Base64.decodeBase64(encryptedText.getBytes());
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] plainTextPwdBytes = (cipher.doFinal(encrypedPwdBytes));
return new String(plainTextPwdBytes, "UTF8");
}
/**
* Decrypt a password encrypted by Web Client
*
* @param password Encrypted password
* @param passPhrase Password phrase
* @return Plain password
*/
public static String decryptPassword(String password, String passPhrase) {
String result = password;
if (!Converter.isEmpty(result)) {
AesUtil aesUtil = new AesUtil(128, 1000);
String decryptedPassword = new String(java.util.Base64.getDecoder().decode(password));
String[] tokens = decryptedPassword != null ? decryptedPassword.split("::") : new String[0];
if (tokens.length == 3) {
result = aesUtil.decrypt(tokens[1], tokens[0], passPhrase, tokens[2]);
}
} else {
result = null;
}
return result;
}
/**
* Clear file name (removing spaces, especial characters, etc)
*
* @param filename Nombre del fichero
* @return Valor limpio
*/
public static String getCleanFileName(String filename) {
return getRemoveTilde(filename).replaceAll(" ", "_");
}
/**
* Generate unique identifier
*
* @return UUID
*/
public static String getUUID() {
UUID uuid = UUID.randomUUID();
String[] tokens = uuid.toString().toUpperCase().split("-");
String result = "";
for (String token : tokens) {
result += token;
}
return result.toLowerCase();
}
/**
* Get a value if have characters or null
*
* @param value Value to check
* @return value or null
*/
public static String getNullOrValue(String value) {
return isEmpty(value) ? null : value;
}
/**
* Get a value if have characters or empty string
*
* @param value Value to check
* @return value or empty string
*/
public static String getEmptyOrValue(String value) {
return isEmpty(value) ? "" : value;
}
/**
* Build a list from integer range
*
* @param min Minimum value
* @param max Maximum value
* @return List
*/
public static List buildList(Integer min, Integer max) {
List result = new ArrayList<>();
if (min != null && max != null) {
for (long i = min; i <= max; i++) {
result.add(i);
}
}
return result;
}
/**
* Build a list from year range
*
* @param dbResult DB result set (min, max)
* @return List
*/
public static List buildYears(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy