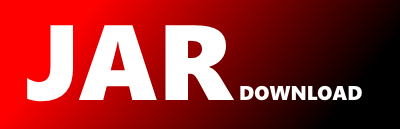
src.main.java.com.vincomobile.fw.basic.tools.IDUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.tools;
import org.apache.commons.lang.RandomStringUtils;
import java.util.Calendar;
import java.util.Date;
import java.util.Random;
public final class IDUtil {
private IDUtil() {
throw new AssertionError();
}
// API
public final static String randomPositiveLongAsString() {
return Long.toString(randomPositiveLong());
}
public final static String randomNegativeLongAsString() {
return Long.toString(randomNegativeLong());
}
public final static long randomPositiveLong() {
long id = new Random().nextLong() * 10000;
id = (id < 0) ? (-1 * id) : id;
return id;
}
public final static long randomNegativeLong() {
long id = new Random().nextLong() * 10000;
id = (id > 0) ? (-1 * id) : id;
return id;
}
public final static String randomPositiveIntAsString() {
return Integer.toString(randomPositiveInt());
}
public final static String randomNegativeIntAsString() {
return Integer.toString(randomNegativeInt());
}
public final static int randomPositiveInt() {
int id = new Random().nextInt() * 10000;
id = (id < 0) ? (-1 * id) : id;
return id;
}
public final static int randomNegativeInt() {
int id = new Random().nextInt() * 10000;
id = (id > 0) ? (-1 * id) : id;
return id;
}
public final static double randomPositiveDouble() {
double id = new Random().nextDouble() * 10000;
id = (id < 0) ? (-1 * id) : id;
return id;
}
public final static double randomNegativeDouble() {
double id = new Random().nextDouble() * 10000;
id = (id > 0) ? (-1 * id) : id;
return id;
}
public final static String randomString(int count) {
return RandomStringUtils.randomAlphanumeric(count);
}
public final static String randomString() {
return RandomStringUtils.randomAlphanumeric(10);
}
public final static boolean randomBoolean() {
return randomPositiveInt() % 2 == 0;
}
public final static Date randomDate() {
int days = randomPositiveInt() % 365;
Calendar cal = Calendar.getInstance();
cal.setTime(new Date());
cal.add(Calendar.DAY_OF_YEAR, days);
return cal.getTime();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy