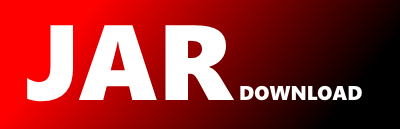
src.main.java.com.vincomobile.fw.basic.tools.Mailer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.tools;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.JavaMailSenderImpl;
import javax.mail.internet.MimeMessage;
import java.util.Map;
public interface Mailer {
/**
* Obtiene el From configurado
*
* @return From
*/
String getFromUser();
/**
* Send a text mail
*
* @param from From address
* @param to Address list (separate by , or ;)
* @param subject Message subject
* @param text Message text
* @return Success or not
*/
boolean sendTextEmail(String from, String to, String subject, String text);
/**
* Send a email (MIME)
*
* @param from From address
* @param to Address list (separate by , or ;)
* @param subject Message subject
* @param body Message text
* @param resources Inline resources
* @param attachments File attachments ([file name] -> [full path to file])
* @param customSender User account to send email
* @param bcc BCC field
* @param mimeMessage Mime message
* @return Success or not
*/
boolean sendMimeEmail(String from, String to, String subject, String body, Map resources, Map attachments, JavaMailSender customSender, String bcc, MimeMessage mimeMessage);
boolean sendMimeEmail(String from, String to, String subject, String body, Map resources, Map attachments, JavaMailSender customSender, String bcc);
boolean sendMimeEmail(String from, String to, String subject, String body, Map resources, Map attachments, JavaMailSender customSender);
boolean sendMimeEmail(String from, String to, String subject, String body, Map resources, Map attachments);
/**
* Send a email (MIME) defined in a template
*
* @param from From address
* @param to Address list (separate by , or ;)
* @param subject Message subject
* @param template Template for Apache Velocity
* @param model Hashmap with model for template
* @param resources Inline resources
* @param attachments File attachments ([file name] -> [full path to file])
* @param customSender User account to send email
* @return Success or not
*/
boolean sendTemplateEmail(String from, String to, String subject, String template, Map model, Map resources, Map attachments, JavaMailSender customSender);
boolean sendTemplateEmail(String from, String to, String subject, String template, Map model, Map resources, Map attachments);
boolean sendTemplateEmail(String from, String to, String subject, String template, Map model, Map resources);
boolean sendTemplateEmail(String from, String to, String subject, String template, Map model);
/**
* Busca la cantidad de registro MX para el email
*
* @param email Nombre del host smtp
* @return Cantidad de host con MX
*/
int doLookupMX(String email);
/**
* Get sender configuration
*
* @param sender Sender implementation
* @return Current configuration
*/
MailerSenderConfig getSenderConfig(JavaMailSenderImpl sender);
/**
* Set sender configuration
*
* @param sender Sender implementation
* @param config Configuration
*/
void setSenderConfig(JavaMailSenderImpl sender, MailerSenderConfig config);
/**
* Set sender configuration to use SSL
*
* @param sender Sender implementation
* @param ssl Use or not SSL
*/
void setSenderConfigSSL(JavaMailSenderImpl sender, boolean ssl);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy