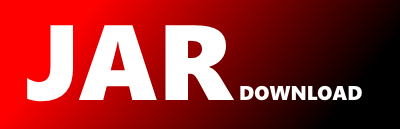
src.main.java.com.vincomobile.fw.basic.business.AdChartResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.business;
import com.vincomobile.fw.basic.persistence.model.AdChart;
import com.vincomobile.fw.basic.tools.Converter;
import java.util.ArrayList;
import java.util.List;
public class AdChartResult {
private String ctype;
private String keyField;
private String nameField;
private String dateMode;
private int year;
private String titleX;
private String titleY;
private Boolean showTitleX;
private Boolean showTitleY;
private String legend1;
private String legend2;
private String legend3;
private String legend4;
private String legend5;
private String legend6;
private String legend7;
private String legend8;
private String legend9;
private String legend10;
private String color1;
private String color2;
private String color3;
private String color4;
private String color5;
private String color6;
private String color7;
private String color8;
private String color9;
private String color10;
private int items = 1;
private List values = new ArrayList<>();
public void addValue(AdChartValue value) {
this.values.add(value);
}
public AdChartValue getValue(String valueId) {
for (AdChartValue value : this.values) {
if (value.getId().equals(valueId))
return value;
}
return null;
}
public void setChart(AdChart chart) {
ctype = chart.getCtype();
titleX = chart.getTitleX();
titleY = chart.getTitleY();
showTitleX = chart.getShowTitleX();
showTitleY = chart.getShowTitleY();
legend1 = chart.getLegend1();
legend2 = chart.getLegend2();
legend3 = chart.getLegend3();
legend4 = chart.getLegend4();
legend5 = chart.getLegend5();
legend6 = chart.getLegend6();
legend7 = chart.getLegend7();
legend8 = chart.getLegend8();
legend9 = chart.getLegend9();
legend10 = chart.getLegend10();
color1 = Converter.getNullOrValue(chart.getColor1());
color2 = Converter.getNullOrValue(chart.getColor2());
color3 = Converter.getNullOrValue(chart.getColor3());
color4 = Converter.getNullOrValue(chart.getColor4());
color5 = Converter.getNullOrValue(chart.getColor5());
color6 = Converter.getNullOrValue(chart.getColor6());
color7 = Converter.getNullOrValue(chart.getColor7());
color8 = Converter.getNullOrValue(chart.getColor8());
color9 = Converter.getNullOrValue(chart.getColor9());
color10 = Converter.getNullOrValue(chart.getColor10());
keyField = chart.getKeyField();
nameField = chart.getNameField();
}
public void setLegend(int indx, String value) {
switch (indx) {
case 1:
legend1 = value;
break;
case 2:
legend2 = value;
break;
case 3:
legend3 = value;
break;
case 4:
legend4 = value;
break;
case 5:
legend5 = value;
break;
case 6:
legend6 = value;
break;
case 7:
legend7 = value;
break;
case 8:
legend8 = value;
break;
case 9:
legend9 = value;
break;
case 10:
legend10 = value;
break;
}
}
public void setColor(int indx, String value) {
switch (indx) {
case 1:
color1 = value;
break;
case 2:
color2 = value;
break;
case 3:
color3 = value;
break;
case 4:
color4 = value;
break;
case 5:
color5 = value;
break;
case 6:
color6 = value;
break;
case 7:
color7 = value;
break;
case 8:
color8 = value;
break;
case 9:
color9 = value;
break;
case 10:
color10 = value;
break;
}
}
public String getCtype() {
return ctype;
}
public void setCtype(String ctype) {
this.ctype = ctype;
}
public String getKeyField() {
return keyField;
}
public void setKeyField(String keyField) {
this.keyField = keyField;
}
public String getNameField() {
return nameField;
}
public void setNameField(String nameField) {
this.nameField = nameField;
}
public String getTitleX() {
return titleX;
}
public void setTitleX(String titleX) {
this.titleX = titleX;
}
public String getTitleY() {
return titleY;
}
public void setTitleY(String titleY) {
this.titleY = titleY;
}
public Boolean getShowTitleX() {
return showTitleX;
}
public void setShowTitleX(Boolean showTitleX) {
this.showTitleX = showTitleX;
}
public Boolean getShowTitleY() {
return showTitleY;
}
public void setShowTitleY(Boolean showTitleY) {
this.showTitleY = showTitleY;
}
public List getValues() {
return values;
}
public void setValues(List values) {
this.values = values;
}
public int getItems() {
return items;
}
public void setItems(int items) {
this.items = items;
}
public String getLegend1() {
return legend1;
}
public void setLegend1(String legend1) {
this.legend1 = legend1;
}
public String getLegend2() {
return legend2;
}
public void setLegend2(String legend2) {
this.legend2 = legend2;
}
public String getLegend3() {
return legend3;
}
public void setLegend3(String legend3) {
this.legend3 = legend3;
}
public String getLegend4() {
return legend4;
}
public void setLegend4(String legend4) {
this.legend4 = legend4;
}
public String getLegend5() {
return legend5;
}
public void setLegend5(String legend5) {
this.legend5 = legend5;
}
public String getLegend6() {
return legend6;
}
public void setLegend6(String legend6) {
this.legend6 = legend6;
}
public String getLegend7() {
return legend7;
}
public void setLegend7(String legend7) {
this.legend7 = legend7;
}
public String getLegend8() {
return legend8;
}
public void setLegend8(String legend8) {
this.legend8 = legend8;
}
public String getLegend9() {
return legend9;
}
public void setLegend9(String legend9) {
this.legend9 = legend9;
}
public String getLegend10() {
return legend10;
}
public void setLegend10(String legend10) {
this.legend10 = legend10;
}
public String getColor1() {
return color1;
}
public void setColor1(String color1) {
this.color1 = color1;
}
public String getColor2() {
return color2;
}
public void setColor2(String color2) {
this.color2 = color2;
}
public String getColor3() {
return color3;
}
public void setColor3(String color3) {
this.color3 = color3;
}
public String getColor4() {
return color4;
}
public void setColor4(String color4) {
this.color4 = color4;
}
public String getColor5() {
return color5;
}
public void setColor5(String color5) {
this.color5 = color5;
}
public String getColor6() {
return color6;
}
public void setColor6(String color6) {
this.color6 = color6;
}
public String getColor7() {
return color7;
}
public void setColor7(String color7) {
this.color7 = color7;
}
public String getColor8() {
return color8;
}
public void setColor8(String color8) {
this.color8 = color8;
}
public String getColor9() {
return color9;
}
public void setColor9(String color9) {
this.color9 = color9;
}
public String getColor10() {
return color10;
}
public void setColor10(String color10) {
this.color10 = color10;
}
public String getDateMode() {
return dateMode;
}
public void setDateMode(String dateMode) {
this.dateMode = dateMode;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy