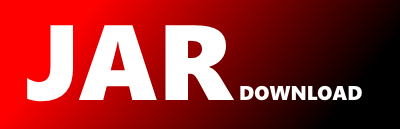
src.main.java.com.vincomobile.fw.basic.business.AdChartValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.business;
import java.util.ArrayList;
import java.util.List;
public class AdChartValue {
private String id;
private String X;
private String fullX;
private Object value;
private Double value1;
private Double value2;
private Double value3;
private Double value4;
private Double value5;
private Double value6;
private Double value7;
private Double value8;
private Double value9;
private Double value10;
private List valueList;
public AdChartValue(String id) {
this.id = id;
this.value1 = 0.0;
this.value2 = 0.0;
this.value3 = 0.0;
this.value4 = 0.0;
this.value5 = 0.0;
this.value6 = 0.0;
this.value7 = 0.0;
this.value8 = 0.0;
this.value9 = 0.0;
this.value10 = 0.0;
this.value = null;
this.valueList = new ArrayList<>();
}
public AdChartValue(String id, String X, String fullX) {
this.id = id;
this.X = X;
this.fullX = fullX;
this.value1 = 0.0;
this.value2 = 0.0;
this.value3 = 0.0;
this.value4 = 0.0;
this.value5 = 0.0;
this.value6 = 0.0;
this.value7 = 0.0;
this.value8 = 0.0;
this.value9 = 0.0;
this.value10 = 0.0;
this.value = null;
this.valueList = new ArrayList<>();
}
public void add(AdChartValue value) {
this.value1 += value.value1;
this.value2 += value.value2;
this.value3 += value.value3;
this.value4 += value.value4;
this.value5 += value.value5;
this.value6 += value.value6;
this.value7 += value.value7;
this.value8 += value.value8;
this.value9 += value.value9;
this.value10 += value.value10;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getX() {
return X;
}
public void setX(String x) {
X = x;
}
public String getFullX() {
return fullX;
}
public void setFullX(String fullX) {
this.fullX = fullX != null ? fullX : X;
}
public void setValue(int indx, Double value) {
switch (indx) {
case 1:
value1 = value;
break;
case 2:
value2 = value;
break;
case 3:
value3 = value;
break;
case 4:
value4 = value;
break;
case 5:
value5 = value;
break;
case 6:
value6 = value;
break;
case 7:
value7 = value;
break;
case 8:
value8 = value;
break;
case 9:
value9 = value;
break;
case 10:
value10 = value;
break;
}
}
public Double getValue1() {
return value1;
}
public void setValue1(Double value1) {
this.value1 = value1;
}
public Double getValue2() {
return value2;
}
public void setValue2(Double value2) {
this.value2 = value2;
}
public Double getValue3() {
return value3;
}
public void setValue3(Double value3) {
this.value3 = value3;
}
public Double getValue4() {
return value4;
}
public void setValue4(Double value4) {
this.value4 = value4;
}
public Double getValue5() {
return value5;
}
public void setValue5(Double value5) {
this.value5 = value5;
}
public Double getValue6() {
return value6;
}
public void setValue6(Double value6) {
this.value6 = value6;
}
public Double getValue7() {
return value7;
}
public void setValue7(Double value7) {
this.value7 = value7;
}
public Double getValue8() {
return value8;
}
public void setValue8(Double value8) {
this.value8 = value8;
}
public Double getValue9() {
return value9;
}
public void setValue9(Double value9) {
this.value9 = value9;
}
public Double getValue10() {
return value10;
}
public void setValue10(Double value10) {
this.value10 = value10;
}
public List getValueList() {
return valueList;
}
public void setValueList(List valueList) {
this.valueList = valueList;
}
public Object getValue() {
return value;
}
public void setValue(Object value) {
this.value = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy