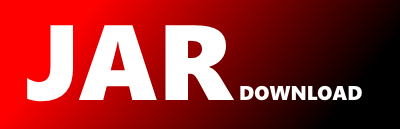
src.main.java.com.vincomobile.fw.basic.hooks.AdPreferenceValidate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.hooks;
import com.vincomobile.fw.basic.FWConfig;
import com.vincomobile.fw.basic.business.SecurityLevel;
import com.vincomobile.fw.basic.business.ValidationError;
import com.vincomobile.fw.basic.persistence.model.AdPreference;
import com.vincomobile.fw.basic.persistence.services.AdMessageService;
import com.vincomobile.fw.basic.persistence.services.AdPreferenceService;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
import java.util.List;
@Component("AdPreferenceValidateFW")
@Qualifier("hookBase")
public class AdPreferenceValidate extends Hook {
private final AdPreferenceService preferenceService;
private final AdMessageService messageService;
private static final SecurityLevel[] levels = new SecurityLevel[] {
new SecurityLevel(4, 10, 0, 0),
new SecurityLevel(6, 15, 0, 0),
new SecurityLevel(8, 20, 3, 180),
new SecurityLevel(8, 30, 5, 90),
};
public AdPreferenceValidate(AdPreferenceService preferenceService, AdMessageService messageService) {
this.preferenceService = preferenceService;
this.messageService = messageService;
name = FWConfig.HOOK_PREFERENCE_VALIDATE;
packageName = "[VincoFW]";
}
/**
* Execute a hook
*
* @param result Result execution
* @param arguments Arguments (List, AdPreference)
*/
@Override
public void execute(HookResult result, Object... arguments) {
logger.info(packageName + " Execute hook: " + name);
List errors = (List) arguments[0];
AdPreference preference = (AdPreference) arguments[1];
if (AdPreferenceService.GLOBAL_PASSWORD_CHECK_MODE.equals(preference.getProperty())) {
int level = getLevel(preference.getValue());
if (level == -1) {
// Check valid mode
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, "AD_ValidationErrorPasswdMode"));
} else {
// Check min and max size
AdPreference prefSizeMin = getPreference(AdPreferenceService.GLOBAL_PASSWORD_SIZE_MIN, preference);
AdPreference prefSizeMax = getPreference(AdPreferenceService.GLOBAL_PASSWORD_SIZE_MAX, preference);
if (prefSizeMin == null) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, "AD_ValidationErrorNotPasswdSizeMin"));
}
if (prefSizeMax == null) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, "AD_ValidationErrorNotPasswdSizeMax"));
}
if (errors.size() == 0) {
// Validate min and max for the mode
if (prefSizeMin.getLong(0L) < levels[level].getSizeMin()) {
prefSizeMin.setValue("" + levels[level].getSizeMin());
preferenceService.update(prefSizeMin);
}
if (prefSizeMax.getLong(0L) > levels[level].getSizeMax()) {
prefSizeMax.setValue("" + levels[level].getSizeMax());
preferenceService.update(prefSizeMax);
}
if (AdPreferenceService.PASSWORD_CHECK_MODE_HIGH.equals(preference.getValue()) || AdPreferenceService.PASSWORD_CHECK_MODE_SECURE.equals(preference.getValue())) {
// Check store count and valid days
AdPreference prefStoreCount = getPreference(AdPreferenceService.GLOBAL_PASSWORD_STORE_COUNT, preference);
AdPreference prefValidDays = getPreference(AdPreferenceService.GLOBAL_PASSWORD_VALID_DAYS, preference);
if (prefStoreCount == null) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, "AD_ValidationErrorNotPasswdStoreCount"));
}
if (prefValidDays == null) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, "AD_ValidationErrorNotPasswdValidDays"));
}
if (errors.size() == 0) {
// Validate store count and valid days for the mode
if (prefStoreCount.getLong(0L) < levels[level].getStoreCount()) {
prefStoreCount.setValue("" + levels[level].getStoreCount());
preferenceService.update(prefStoreCount);
}
if (prefValidDays.getLong(0L) <= 0 || prefValidDays.getLong(0L) > levels[level].getValidDays()) {
prefValidDays.setValue("" +levels[level].getValidDays());
preferenceService.update(prefValidDays);
}
}
}
}
}
} else if (isPasswordProperty(preference.getProperty())) {
AdPreference prefCheckMode = getPreference(AdPreferenceService.GLOBAL_PASSWORD_CHECK_MODE, preference);
if (prefCheckMode != null) {
// Check value for a mode
int level = getLevel(prefCheckMode.getValue());
if (AdPreferenceService.GLOBAL_PASSWORD_SIZE_MIN.equals(preference.getProperty())) {
if (preference.getLong(0L) < levels[level].getSizeMin()) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_TEXT, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdSizeMin", levels[level].getSizeMin())));
}
AdPreference prefSizeMax = getPreference(AdPreferenceService.GLOBAL_PASSWORD_SIZE_MAX, preference);
if (prefSizeMax != null && prefSizeMax.getLong() <= preference.getLong(0L)) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdSizeMinMax")));
}
} else if (AdPreferenceService.GLOBAL_PASSWORD_SIZE_MAX.equals(preference.getProperty())) {
if (preference.getLong(0L) < levels[level].getSizeMax()) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_TEXT, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdSizeMax", levels[level].getSizeMax())));
}
AdPreference prefSizeMin = getPreference(AdPreferenceService.GLOBAL_PASSWORD_SIZE_MIN, preference);
if (prefSizeMin != null && prefSizeMin.getLong() >= preference.getLong(0L)) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_MESSAGE, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdSizeMinMax")));
}
} else if (AdPreferenceService.GLOBAL_PASSWORD_STORE_COUNT.equals(preference.getProperty())) {
if (preference.getLong(0L) < levels[level].getStoreCount()) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_TEXT, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdStoreCount", levels[level].getStoreCount())));
}
} else if (AdPreferenceService.GLOBAL_PASSWORD_VALID_DAYS.equals(preference.getProperty())) {
if (preference.getLong(0L) < levels[level].getValidDays()) {
errors.add(new ValidationError(ValidationError.TYPE_GLOBAL, "", ValidationError.CODE_TEXT, messageService.getMessage(preference.getIdClient(), null, "AD_ValidationErrorPasswdValidDays", levels[level].getValidDays())));
}
}
}
}
}
/**
* Check if preference is for password
*
* @param property Property name
* @return If is password preference
*/
private boolean isPasswordProperty(String property) {
return AdPreferenceService.GLOBAL_PASSWORD_SIZE_MIN.equals(property) ||
AdPreferenceService.GLOBAL_PASSWORD_SIZE_MAX.equals(property) ||
AdPreferenceService.GLOBAL_PASSWORD_STORE_COUNT.equals(property) ||
AdPreferenceService.GLOBAL_PASSWORD_VALID_DAYS.equals(property);
}
/**
* Get the security level for mode
*
* @param mode Check mode
* @return Level
*/
private int getLevel(String mode) {
return (AdPreferenceService.PASSWORD_CHECK_MODE_BASIC.equals(mode) ? 0 :
(AdPreferenceService.PASSWORD_CHECK_MODE_STANDARD.equals(mode) ? 1 :
(AdPreferenceService.PASSWORD_CHECK_MODE_HIGH.equals(mode) ? 2 :
(AdPreferenceService.PASSWORD_CHECK_MODE_SECURE.equals(mode) ? 3 : -1))));
}
/**
* Get preference (related to validation)
*
* @param property Property name
* @param preference Prefence to valitate
* @return Preference
*/
private AdPreference getPreference(String property, AdPreference preference) {
AdPreference result = preferenceService.getPreference(property, preference.getIdClient(), preference.getVisibleatIdRole(), preference.getVisibleatIdUser(), preference.getVisibleatCustom());
return result == null || result.getIdPreference() == null ? null : result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy