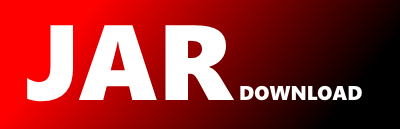
src.main.java.com.vincomobile.fw.basic.tools.MailerUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.tools;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.mail.javamail.MimeMessageHelper;
import javax.mail.MessagingException;
import javax.mail.internet.AddressException;
import javax.mail.internet.InternetAddress;
public class MailerUtils {
private static Logger logger = LoggerFactory.getLogger(MailerUtils.class);
/**
* Split email address
*
* @param items Emails separated by , or ;
* @return Email address
*/
public static String[] getAddress(String items) {
items = items.replace(',', ';');
return items.split(";");
}
/**
* Get a list emails address
*
* @param items Emails separated by , or ;
* @return Email address
* @throws AddressException
*/
public static InternetAddress[] getInternetAddress(String items) {
String[] dir = getAddress(items);
InternetAddress[] address = new InternetAddress[dir.length];
for (int i = 0; i < dir.length; i++) {
try {
address[i] = new InternetAddress(dir[i]);
} catch (Throwable ex) {
logger.error("Invalid address: " + dir[i], ex);
logger.error("Exception: " + ex.getMessage());
return null;
}
}
return address;
}
/**
* Get host part for a email address
*
* @param email Email address ([email protected])
* @return Host part (host.com)
*/
public static String getHostPart(String email) {
String[] tokens = email.split("@");
return tokens[1];
}
/**
* Set BCC emmail
*
* @param helper Message helper
* @param bcc BCC email
* @return Successful if BCC is set
*/
public static boolean setBCC(MimeMessageHelper helper, String bcc) throws MessagingException {
if (bcc != null) {
// Set BCC address
final InternetAddress[] toBcc = MailerUtils.getInternetAddress(bcc);
if (toBcc == null) {
return false;
}
helper.setBcc(toBcc);
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy