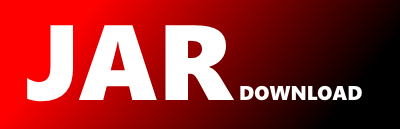
src.main.java.com.vincomobile.fw.basic.tools.ZipManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vincofw-basic Show documentation
Show all versions of vincofw-basic Show documentation
Vincomobile framework (Basic components)
package com.vincomobile.fw.basic.tools;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipManager {
private static Log log = LogFactory.getLog(ZipManager.class);
private String fileNameCompressed;
private OutputStream fileOS;
private ZipOutputStream zipOS;
byte[] buffer;
int rBytes;
public ZipManager(String fileName) {
try {
fileNameCompressed = fileName;
fileOS = new FileOutputStream(fileName);
zipOS = new ZipOutputStream(fileOS);
buffer = new byte[Constants.MAX_LENGTH_READ];
rBytes = 0;
} catch (Exception e) {
log.error("Error creating ZIP:" + e.getMessage());
}
}
public ZipManager(OutputStream fileOS) {
this.fileOS = fileOS;
zipOS = new ZipOutputStream(fileOS);
buffer = new byte[Constants.MAX_LENGTH_READ];
rBytes = 0;
}
public ZipManager(File file) {
try {
fileNameCompressed = file.getPath();
fileOS = new FileOutputStream(file);
zipOS = new ZipOutputStream(fileOS);
buffer = new byte[Constants.MAX_LENGTH_READ];
rBytes = 0;
} catch (Exception e) {
log.error("Error creating ZIP:" + e.getMessage());
}
}
/**
* Get full path for Zip
*
* @return File name
*/
public String getZipFile() {
return fileNameCompressed;
}
/**
* Add directory content to .ZIP
*
* @param fileDirName Directory name to compress
* @throws Exception
*/
public void addDirContentToZipFile(String fileDirName) throws Exception {
File fileDir = new File(fileDirName);
if (fileDir.exists() && fileDir.isDirectory()) {
addDirContentToZipFile(fileDir);
}
}
/**
* Add directory content to .ZIP
*
* @param fileDir Directory to compress
* @throws Exception
*/
public void addDirContentToZipFile(File fileDir) throws Exception {
if ( (fileDir.exists()) && (fileDir.isDirectory()) ) {
File[] files = fileDir.listFiles();
for (File file : files) {
if ((file.isFile()) && (!file.isHidden())) {
addFileToZipFile(file);
}
}
}
}
/**
* Add a file to .ZIP
*
* @param fileName File name to compress
* @throws Exception
*/
public void addFileToZipFile(String fileName) throws Exception {
File file = new File(fileName);
if (file.exists() && file.isFile()) {
addFileToZipFile(file);
}
}
/**
* Add a file to .ZIP
*
* @param file File to compress
* @param entry Zip entry name
* @throws Exception
*/
public void addFileToZipFile(File file, String entry) throws Exception {
if (fileNameCompressed == null || !file.getAbsolutePath().toLowerCase().equals(fileNameCompressed.toLowerCase())) {
FileInputStream fileIS = new FileInputStream(file);
ZipEntry zipEntry = new ZipEntry(entry);
zipOS.putNextEntry(zipEntry);
while ((rBytes = fileIS.read(buffer)) != -1)
zipOS.write(buffer, 0, rBytes);
zipOS.closeEntry();
fileIS.close();
}
}
public void addFileToZipFile(File file) throws Exception {
addFileToZipFile(file, file.getName());
}
public ZipEntry addEntry(String entry) throws IOException {
ZipEntry zipEntry = new ZipEntry(entry);
zipOS.putNextEntry(zipEntry);
return zipEntry;
}
/**
* Add data to .ZIP
*
* @param buffer Bytes to add
* @throws IOException
*/
public void writeToOS(byte[] buffer) throws IOException {
zipOS.write(buffer);
}
public void closeEntry() throws IOException {
zipOS.closeEntry();
}
/**
* Close zip file
*
* @throws Exception
*/
public void closeZip() throws Exception {
zipOS.close();
fileOS.close();
}
public ZipOutputStream getZipOS() {
return zipOS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy