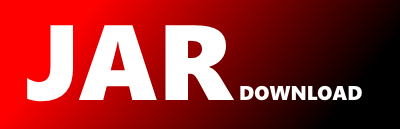
com.vip.saturn.job.console.mybatis.service.impl.CurrentJobConfigServiceImpl Maven / Gradle / Ivy
/**
* Copyright 2016 vip.com.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
**/
package com.vip.saturn.job.console.mybatis.service.impl;
import com.vip.saturn.job.console.exception.SaturnJobConsoleException;
import com.vip.saturn.job.console.mybatis.entity.JobConfig4DB;
import com.vip.saturn.job.console.mybatis.repository.CurrentJobConfigRepository;
import com.vip.saturn.job.console.mybatis.service.CurrentJobConfigService;
import com.vip.saturn.job.console.mybatis.service.HistoryJobConfigService;
import org.apache.commons.io.IOUtils;
import org.apache.ibatis.session.ExecutorType;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import javax.annotation.Resource;
import java.util.Date;
import java.util.List;
import java.util.Map;
@Service
public class CurrentJobConfigServiceImpl implements CurrentJobConfigService {
@Resource
private HistoryJobConfigService historyJobConfigService;
@Autowired
private CurrentJobConfigRepository currentJobConfigRepo;
@Autowired
private SqlSessionFactory sqlSessionFactory;
@Transactional(rollbackFor = Exception.class)
@Override
public int create(JobConfig4DB currentJobConfig) throws SaturnJobConsoleException {
return currentJobConfigRepo.insert(currentJobConfig);
}
@Transactional(rollbackFor = Exception.class)
@Override
public int deleteByPrimaryKey(Long id) throws SaturnJobConsoleException {
return currentJobConfigRepo.deleteByPrimaryKey(id);
}
@Transactional(rollbackFor = Exception.class)
@Override
public int updateByPrimaryKey(JobConfig4DB currentJobConfig) throws SaturnJobConsoleException {
return currentJobConfigRepo.updateByPrimaryKey(currentJobConfig);
}
@Override
public void batchUpdatePreferList(List jobConfigs) throws SaturnJobConsoleException {
SqlSession batchSqlSession = sqlSessionFactory.openSession(ExecutorType.BATCH, false);
try {
for (JobConfig4DB currentJobConfig : jobConfigs) {
batchSqlSession.getMapper(CurrentJobConfigRepository.class).updatePreferList(currentJobConfig);
}
batchSqlSession.commit();
} catch (Exception e) {
batchSqlSession.rollback();
throw new SaturnJobConsoleException("error when batchUpdatePreferList", e);
} finally {
IOUtils.closeQuietly(batchSqlSession);
}
}
@Override
public JobConfig4DB findConfigByNamespaceAndJobName(String namespace, String jobName)
throws SaturnJobConsoleException {
return currentJobConfigRepo.findConfigByNamespaceAndJobName(namespace, jobName);
}
@Transactional(rollbackFor = Exception.class)
@Override
public void updateNewAndSaveOld2History(final JobConfig4DB newJobConfig, final JobConfig4DB oldJobConfig,
final String userName) throws SaturnJobConsoleException {
// 持久化当前配置
if (userName != null) {
newJobConfig.setLastUpdateBy(userName);
}
newJobConfig.setLastUpdateTime(new Date());
updateByPrimaryKey(newJobConfig);
// 持久化历史配置
oldJobConfig.setId(null);
historyJobConfigService.create(oldJobConfig);
}
@Transactional(rollbackFor = Exception.class)
@Override
public void updateStream(JobConfig4DB currentJobConfig) throws SaturnJobConsoleException {
currentJobConfigRepo.updateStream(currentJobConfig);
}
@Override
public List findConfigByQueue(String queue) {
return currentJobConfigRepo.findConfigsByQueue(queue);
}
@Override
public List findConfigsByNamespace(String namespace) throws SaturnJobConsoleException {
return currentJobConfigRepo.findConfigsByNamespace(namespace);
}
@Override
public List findConfigsByNamespaceWithCondition(String namespace, Map condition,
Pageable pageable) throws SaturnJobConsoleException {
return currentJobConfigRepo.findConfigsByNamespaceWithCondition(namespace, condition, pageable);
}
@Override
public int countConfigsByNamespaceWithCondition(String namespace, Map condition)
throws SaturnJobConsoleException {
return currentJobConfigRepo.countConfigsByNamespaceWithCondition(namespace, condition);
}
@Override
public int countEnabledUnSystemJobsByNamespace(String namespace) throws SaturnJobConsoleException {
return currentJobConfigRepo.countEnabledUnSystemJobsByNamespace(namespace, 1);
}
@Override
public List findConfigNamesByNamespace(String namespace) throws SaturnJobConsoleException {
return currentJobConfigRepo.findConfigNamesByNamespace(namespace);
}
@Override
public void batchSetGroups(String namespace, List jobNames, String groupName, String userName) {
currentJobConfigRepo.batchSetGroups(namespace, jobNames, groupName, userName);
}
@Override
public void addToGroups(String namespace, List jobNames, String groupName, String userName) {
currentJobConfigRepo.addToGroups(namespace, jobNames, groupName, userName);
}
@Override
public List findHasValidJobNamespaces(String jobType, int isEnabled) {
return currentJobConfigRepo.findHasValidJobNamespaces(jobType, isEnabled);
}
@Override
public List findValidJobsCronConfig() {
return currentJobConfigRepo.findValidJobsCronConfig();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy