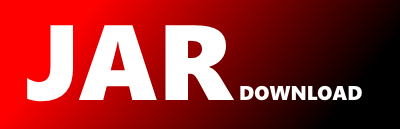
com.vip.saturn.job.console.service.JobService Maven / Gradle / Ivy
/**
* Copyright 1999-2015 dangdang.com.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.vip.saturn.job.console.service;
import com.vip.saturn.job.console.domain.*;
import com.vip.saturn.job.console.exception.SaturnJobConsoleException;
import com.vip.saturn.job.console.mybatis.entity.JobConfig4DB;
import com.vip.saturn.job.console.repository.zookeeper.CuratorRepository.CuratorFrameworkOp;
import com.vip.saturn.job.console.vo.GetJobConfigVo;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.util.List;
import java.util.Map;
public interface JobService {
List getGroups(String namespace) throws SaturnJobConsoleException;
void enableJob(String namespace, String jobName, String updatedBy) throws SaturnJobConsoleException;
void disableJob(String namespace, String jobName, String updatedBy) throws SaturnJobConsoleException;
void removeJob(String namespace, String jobName) throws SaturnJobConsoleException;
/**
* 获取该作业可选择的优先Executor
*/
List getCandidateExecutors(String namespace, String jobName) throws SaturnJobConsoleException;
void setPreferList(String namespace, String jobName, String preferList, String updatedBy)
throws SaturnJobConsoleException;
List getCandidateUpStream(String namespace) throws SaturnJobConsoleException;
List getCandidateDownStream(String namespace) throws SaturnJobConsoleException;
void addJob(String namespace, JobConfig jobConfig, String createdBy) throws SaturnJobConsoleException;
void copyJob(String namespace, JobConfig jobConfig, String copyingJobName, String createdBy)
throws SaturnJobConsoleException;
int getMaxJobNum() throws SaturnJobConsoleException;
boolean jobIncExceeds(String namespace, int maxJobNum, int inc) throws SaturnJobConsoleException;
List getUnSystemJobs(String namespace) throws SaturnJobConsoleException;
List getUnSystemJobsWithCondition(String namespace, Map condition, int page, int size)
throws SaturnJobConsoleException;
int countUnSystemJobsWithCondition(String namespace, Map condition)
throws SaturnJobConsoleException;
int countEnabledUnSystemJobs(String namespace) throws SaturnJobConsoleException;
/**
* @deprecated since 3.1.0,不再支持systemJob
*/
@Deprecated
List getUnSystemJobNames(String namespace) throws SaturnJobConsoleException;
List getJobNames(String namespace) throws SaturnJobConsoleException;
/**
* 持久化作业到指定namespace
*/
void persistJobFromDB(String namespace, JobConfig jobConfig) throws SaturnJobConsoleException;
/**
* 持久化作业到特定zk上面
*/
void persistJobFromDB(JobConfig jobConfig, CuratorFrameworkOp curatorFrameworkOp) throws SaturnJobConsoleException;
List importJobs(String namespace, MultipartFile file, String createdBy)
throws SaturnJobConsoleException;
File exportJobs(String namespace) throws SaturnJobConsoleException;
File exportSelectedJobs(String namespace, List jobList) throws SaturnJobConsoleException;
ArrangeLayout getArrangeLayout(String namespace) throws SaturnJobConsoleException;
JobConfig getJobConfigFromZK(String namespace, String jobName) throws SaturnJobConsoleException;
JobConfig getJobConfig(String namespace, String jobName) throws SaturnJobConsoleException;
JobStatus getJobStatus(String namespace, String jobName) throws SaturnJobConsoleException;
JobStatus getJobStatus(String namespace, JobConfig jobConfig) throws SaturnJobConsoleException;
boolean isJobShardingAllocatedExecutor(String namespace, String jobName) throws SaturnJobConsoleException;
List getJobServerList(String namespace, String jobName) throws SaturnJobConsoleException;
GetJobConfigVo getJobConfigVo(String namespace, String jobName) throws SaturnJobConsoleException;
void updateJobConfig(String namespace, JobConfig jobConfig, String updatedBy) throws SaturnJobConsoleException;
List getAllJobNamesFromZK(String namespace) throws SaturnJobConsoleException;
void updateJobCron(String namespace, String jobName, String cron, Map customContext,
String updatedBy) throws SaturnJobConsoleException;
/**
* 获取作业所分配的executor及先关分配信息。
*/
List getJobServers(String namespace, String jobName) throws SaturnJobConsoleException;
/**
* 获取JobServer状态信息
*/
List getJobServersStatus(String namespace, String jobName) throws SaturnJobConsoleException;
void runAtOnce(String namespace, String jobName) throws SaturnJobConsoleException;
void stopAtOnce(String namespace, String jobName) throws SaturnJobConsoleException;
/**
* 获取作业运行状态
*/
List getExecutionStatus(String namespace, String jobName) throws SaturnJobConsoleException;
/**
* 获取运行日志
*/
String getExecutionLog(String namespace, String jobName, String jobItem) throws SaturnJobConsoleException;
/**
* 通过Queue名获取作业信息
*/
List getJobsByQueue(String queue) throws SaturnJobConsoleException;
/**
* 批量设置作业的分组
* @param namespace
* @param jobNames 待设置分组的作业名集合
* @param oldGroupNames 修改前的分组名集合
* @param newGroupsNames 修改后的分组名集合
* @param userName 操作者
*/
void batchSetGroups(String namespace, List jobNames, List oldGroupNames, List newGroupsNames, String userName) throws SaturnJobConsoleException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy