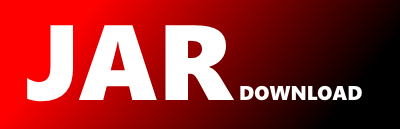
mapper.UserMapper.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.vip.saturn.job.console.mybatis.repository.UserRepository"> <resultMap id="baseResultMap" type="com.vip.saturn.job.console.mybatis.entity.User"> <result column="user_name" property="userName" jdbcType="VARCHAR"/> <result column="password" property="password" jdbcType="VARCHAR"/> <result column="real_name" property="realName" jdbcType="VARCHAR"/> <result column="employee_id" property="employeeId" jdbcType="VARCHAR"/> <result column="email" property="email" jdbcType="VARCHAR"/> <result column="created_by" property="createdBy" jdbcType="VARCHAR"/> <result column="create_time" property="createTime" jdbcType="TIMESTAMP"/> <result column="last_updated_by" property="lastUpdatedBy" jdbcType="VARCHAR"/> <result column="last_update_time" property="lastUpdateTime" jdbcType="TIMESTAMP"/> <result column="is_deleted" property="isDeleted" jdbcType="BOOLEAN"/> </resultMap> <insert id="insert" parameterType="com.vip.saturn.job.console.mybatis.entity.User"> INSERT INTO `user`(`user_name`, `password`, `real_name`, `employee_id`, `email`, `created_by`, `create_time`, `last_updated_by`, `last_update_time`, `is_deleted`) VALUES(#{userName, jdbcType=VARCHAR}, #{password, jdbcType=VARCHAR}, #{realName, jdbcType=VARCHAR}, #{employeeId, jdbcType=VARCHAR}, #{email, jdbcType=VARCHAR}, #{createdBy, jdbcType=VARCHAR}, #{createTime, jdbcType=TIMESTAMP}, #{lastUpdatedBy, jdbcType=VARCHAR}, #{lastUpdateTime, jdbcType=TIMESTAMP}, #{isDeleted, jdbcType=BOOLEAN}) </insert> <update id="update" parameterType="com.vip.saturn.job.console.mybatis.entity.User"> UPDATE `user` SET `password` = #{password, jdbcType=VARCHAR}, `real_name` = #{realName, jdbcType=VARCHAR}, `employee_id` = #{employeeId, jdbcType=VARCHAR}, `email` = #{email, jdbcType=VARCHAR}, `last_updated_by` = #{lastUpdatedBy, jdbcType=VARCHAR}, `is_deleted` = #{isDeleted, jdbcType=BOOLEAN} WHERE `user_name` = #{userName, jdbcType=VARCHAR} </update> <sql id="selectAllSql"> SELECT `user_name`, `password`, `real_name`, `employee_id`, `email`, `created_by`, `create_time`, `last_updated_by`, `last_update_time`, `is_deleted` FROM `user` </sql> <select id="selectAll" resultMap="baseResultMap"> <include refid="selectAllSql"></include> WHERE `is_deleted` = '0' </select> <select id="select" resultMap="baseResultMap"> <include refid="selectAllSql"></include> WHERE `user_name` = #{userName, jdbcType=VARCHAR} AND `is_deleted` = '0' </select> <select id="selectWithNotFilterDeleted" resultMap="baseResultMap"> <include refid="selectAllSql"></include> WHERE `user_name` = #{userName, jdbcType=VARCHAR} </select> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy