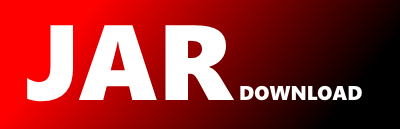
us.codecraft.xsoup.XTokenQueue Maven / Gradle / Ivy
package us.codecraft.xsoup;
import java.util.ArrayList;
import java.util.List;
import org.jsoup.helper.Validate;
import com.virjar.sipsoup.parse.TokenQueue;
/**
* Created by virjar on 17/6/19.
*/
public class XTokenQueue extends TokenQueue {
private static final String[] quotes = { "\"", "'" };
private static final char singleQuote = '\'';
private static final char doubleQuote = '"';
/**
* Create a new TokenQueue.
*
* @param data string of data to back queue.
*/
public XTokenQueue(String data) {
super(data);
}
public String consumeToUnescaped(String str) {
String s = consumeToAny(str);
if (s.length() > 0 && s.charAt(s.length() - 1) == '\\') {
s += consume();
s += consumeToUnescaped(str);
}
Validate.isTrue(pos < queue.length(), "Unclosed quotes! " + queue);
return s;
}
public String consumeAny(String... seq) {
for (String s : seq) {
if (matches(s)) {
pos += s.length();
return s;
}
}
return "";
}
public String chompBalancedQuotes() {
String quote = consumeAny(quotes);
if (quote.length() == 0) {
return "";
}
StringBuilder accum = new StringBuilder(quote);
accum.append(consumeToUnescaped(quote));
accum.append(consume());
return accum.toString();
}
public String chompBalancedNotInQuotes(char open, char close) {
StringBuilder accum = new StringBuilder();
int depth = 0;
char last = 0;
boolean inQuotes = false;
Character quote = null;
do {
if (isEmpty())
break;
Character c = consume();
if (last == 0 || last != ESC) {
if (!inQuotes) {
if (c.equals(singleQuote) || c.equals(doubleQuote)) {
inQuotes = true;
quote = c;
} else if (c.equals(open))
depth++;
else if (c.equals(close))
depth--;
} else {
if (c.equals(quote)) {
inQuotes = false;
}
}
}
if (depth > 0 && last != 0)
accum.append(c); // don't include the outer match pair in the return
last = c;
} while (depth > 0);
return accum.toString();
}
@Deprecated
public List parseFuncionParams() {
List params = new ArrayList();
StringBuilder accum = new StringBuilder();
while (!isEmpty()) {
consumeWhitespace();
if (matchChomp(",")) {
params.add(accum.toString());
accum = new StringBuilder();
} else if (matchesAny(quotes)) {
String quoteUsed = consumeAny(quotes);
accum.append(quoteUsed);
accum.append(consumeToUnescaped(quoteUsed));
accum.append(consume());
} else {
accum.append(consumeToAny("\"", "'", ","));
}
}
if (accum.length() > 0) {
params.add(accum.toString());
}
return params;
}
@Deprecated
public static List parseFuncionParams(String paramStr) {
XTokenQueue tq = new XTokenQueue(paramStr);
return tq.parseFuncionParams();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy