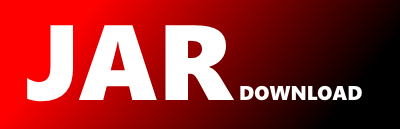
com.virtusa.gto.nyql.engine.repo.QScriptFolders.groovy Maven / Gradle / Ivy
package com.virtusa.gto.nyql.engine.repo
import com.virtusa.gto.nyql.exceptions.NyConfigurationException
import com.virtusa.gto.nyql.exceptions.NyException
import com.virtusa.gto.nyql.model.QScriptMapper
import com.virtusa.gto.nyql.model.QSource
import groovy.transform.CompileStatic
/**
* Stores script mapping from several folders in the system.
*
* @author IWEERARATHNA
*/
@CompileStatic
class QScriptFolders implements QScriptMapper {
private final List scriptsFolderList = []
private final Map fileMap = [:]
QScriptFolders(Collection folders) {
if (folders != null) {
folders.each { addScriptFolder(it) }
}
}
QScriptFolders addScriptFolder(File file) {
def impl = new QScriptsFolder(file).scanDir()
impl.allSources().each {
def scriptId = it.id
if (fileMap.containsKey(scriptsFolderList)) {
throw new NyConfigurationException("Script by id '$scriptId' already loaded! You are trying to load it " +
"from ${file.absolutePath} too!")
}
fileMap.put(scriptId, it)
}
scriptsFolderList << impl
this
}
@SuppressWarnings("UnnecessaryGetter")
static QScriptFolders createNew(Map args) throws NyException {
if (args == null || args.size() == 0 || !args.baseDirs) {
throw new NyConfigurationException('To create a new QScriptsFolder requires at least ' +
'one parameter with specifying one or many directories!')
}
List paths = (List) args.baseDirs
List folders = []
for (String path : paths) {
File dir = new File(path)
if (!dir.exists()) {
String configFilePath = args._location
if (configFilePath != null) {
File activeDir = new File(configFilePath).getCanonicalFile().getParentFile()
if (activeDir.exists() && !dir.isAbsolute()) {
folders << activeDir.toPath().resolve(path).toFile()
continue
}
}
throw new NyConfigurationException("One of script folder does not exist! $path")
}
}
new QScriptFolders(folders)
}
@Override
QSource map(String id) {
fileMap[id]
}
@Override
Collection allSources() {
fileMap.values()
}
@Override
boolean canCacheAtStartup() {
true
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy