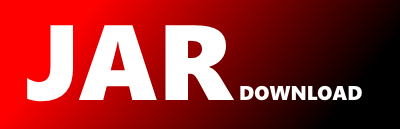
com.vk.api.sdk.objects.base.LinkProduct Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.objects.base;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.vk.api.sdk.objects.Validable;
import com.vk.api.sdk.objects.annotations.Required;
import com.vk.api.sdk.objects.market.Price;
import java.util.Objects;
/**
* LinkProduct object
*/
public class LinkProduct implements Validable {
@SerializedName("category")
private LinkProductCategory category;
@SerializedName("city")
private String city;
@SerializedName("distance")
private Integer distance;
@SerializedName("geo")
private GeoCoordinates geo;
@SerializedName("merchant")
private String merchant;
@SerializedName("orders_count")
private Integer ordersCount;
@SerializedName("price")
@Required
private Price price;
@SerializedName("status")
private LinkProductStatus status;
@SerializedName("type")
private LinkProductType type;
public LinkProductCategory getCategory() {
return category;
}
public LinkProduct setCategory(LinkProductCategory category) {
this.category = category;
return this;
}
public String getCity() {
return city;
}
public LinkProduct setCity(String city) {
this.city = city;
return this;
}
public Integer getDistance() {
return distance;
}
public LinkProduct setDistance(Integer distance) {
this.distance = distance;
return this;
}
public GeoCoordinates getGeo() {
return geo;
}
public LinkProduct setGeo(GeoCoordinates geo) {
this.geo = geo;
return this;
}
public String getMerchant() {
return merchant;
}
public LinkProduct setMerchant(String merchant) {
this.merchant = merchant;
return this;
}
public Integer getOrdersCount() {
return ordersCount;
}
public LinkProduct setOrdersCount(Integer ordersCount) {
this.ordersCount = ordersCount;
return this;
}
public Price getPrice() {
return price;
}
public LinkProduct setPrice(Price price) {
this.price = price;
return this;
}
public LinkProductStatus getStatus() {
return status;
}
public LinkProduct setStatus(LinkProductStatus status) {
this.status = status;
return this;
}
public LinkProductType getType() {
return type;
}
public LinkProduct setType(LinkProductType type) {
this.type = type;
return this;
}
@Override
public int hashCode() {
return Objects.hash(geo, ordersCount, distance, city, price, merchant, category, type, status);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LinkProduct linkProduct = (LinkProduct) o;
return Objects.equals(geo, linkProduct.geo) &&
Objects.equals(ordersCount, linkProduct.ordersCount) &&
Objects.equals(distance, linkProduct.distance) &&
Objects.equals(city, linkProduct.city) &&
Objects.equals(price, linkProduct.price) &&
Objects.equals(merchant, linkProduct.merchant) &&
Objects.equals(category, linkProduct.category) &&
Objects.equals(type, linkProduct.type) &&
Objects.equals(status, linkProduct.status);
}
@Override
public String toString() {
final Gson gson = new Gson();
return gson.toJson(this);
}
public String toPrettyString() {
final StringBuilder sb = new StringBuilder("LinkProduct{");
sb.append("geo=").append(geo);
sb.append(", ordersCount=").append(ordersCount);
sb.append(", distance=").append(distance);
sb.append(", city='").append(city).append("'");
sb.append(", price=").append(price);
sb.append(", merchant='").append(merchant).append("'");
sb.append(", category=").append(category);
sb.append(", type='").append(type).append("'");
sb.append(", status=").append(status);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy