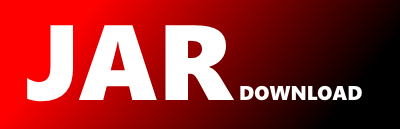
com.vk.api.sdk.objects.messages.ActionOneOf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.objects.messages;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.vk.api.sdk.objects.Validable;
import com.vk.api.sdk.objects.annotations.Required;
import java.util.Objects;
/**
* ActionOneOf object
*/
public class ActionOneOf implements Validable {
/**
* Message ID
*/
@SerializedName("conversation_message_id")
private Integer conversationMessageId;
/**
* Email address for chat_invite_user or chat_kick_user actions
*/
@SerializedName("email")
private String email;
/**
* User or email peer ID
* Entity: owner
*/
@SerializedName("member_id")
private Long memberId;
/**
* Message body of related message
*/
@SerializedName("message")
private String message;
@SerializedName("photo")
private MessageActionPhoto photo;
/**
* New chat title for chat_create and chat_title_update actions
*/
@SerializedName("text")
private String text;
@SerializedName("type")
@Required
private MessageActionStatus type;
public Integer getConversationMessageId() {
return conversationMessageId;
}
public ActionOneOf setConversationMessageId(Integer conversationMessageId) {
this.conversationMessageId = conversationMessageId;
return this;
}
public String getEmail() {
return email;
}
public ActionOneOf setEmail(String email) {
this.email = email;
return this;
}
public Long getMemberId() {
return memberId;
}
public ActionOneOf setMemberId(Long memberId) {
this.memberId = memberId;
return this;
}
public String getMessage() {
return message;
}
public ActionOneOf setMessage(String message) {
this.message = message;
return this;
}
public MessageActionPhoto getPhoto() {
return photo;
}
public ActionOneOf setPhoto(MessageActionPhoto photo) {
this.photo = photo;
return this;
}
public String getText() {
return text;
}
public ActionOneOf setText(String text) {
this.text = text;
return this;
}
public MessageActionStatus getType() {
return type;
}
public ActionOneOf setType(MessageActionStatus type) {
this.type = type;
return this;
}
@Override
public int hashCode() {
return Objects.hash(conversationMessageId, photo, text, message, type, email, memberId);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ActionOneOf actionOneOf = (ActionOneOf) o;
return Objects.equals(memberId, actionOneOf.memberId) &&
Objects.equals(photo, actionOneOf.photo) &&
Objects.equals(text, actionOneOf.text) &&
Objects.equals(message, actionOneOf.message) &&
Objects.equals(type, actionOneOf.type) &&
Objects.equals(email, actionOneOf.email) &&
Objects.equals(conversationMessageId, actionOneOf.conversationMessageId);
}
@Override
public String toString() {
final Gson gson = new Gson();
return gson.toJson(this);
}
public String toPrettyString() {
final StringBuilder sb = new StringBuilder("ActionOneOf{");
sb.append("memberId=").append(memberId);
sb.append(", photo=").append(photo);
sb.append(", text='").append(text).append("'");
sb.append(", message='").append(message).append("'");
sb.append(", type=").append(type);
sb.append(", email='").append(email).append("'");
sb.append(", conversationMessageId=").append(conversationMessageId);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy