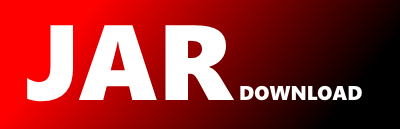
com.vk.api.sdk.queries.ads.AdsGetDemographicsQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.queries.ads;
import com.vk.api.sdk.client.AbstractQueryBuilder;
import com.vk.api.sdk.client.Utils;
import com.vk.api.sdk.client.VkApiClient;
import com.vk.api.sdk.client.actors.UserActor;
import com.vk.api.sdk.objects.ads.GetDemographicsIdsType;
import com.vk.api.sdk.objects.ads.GetDemographicsPeriod;
import com.vk.api.sdk.objects.ads.responses.GetDemographicsResponse;
import com.vk.api.sdk.objects.annotations.ApiParam;
import java.util.Arrays;
import java.util.List;
/**
* Query for Ads.getDemographics method
*/
public class AdsGetDemographicsQuery extends AbstractQueryBuilder> {
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param accountId value of "account id" parameter.
* @param idsType value of "ids type" parameter.
* @param ids value of "ids" parameter.
* @param period value of "period" parameter.
* @param dateFrom value of "date from" parameter.
* @param dateTo value of "date to" parameter.
*/
public AdsGetDemographicsQuery(VkApiClient client, UserActor actor, Integer accountId,
GetDemographicsIdsType idsType, String ids, GetDemographicsPeriod period,
String dateFrom, String dateTo) {
super(client, "ads.getDemographics", Utils.buildParametrizedType(List.class, GetDemographicsResponse.class));
accessToken(actor.getAccessToken());
accountId(accountId);
idsType(idsType);
ids(ids);
period(period);
dateFrom(dateFrom);
dateTo(dateTo);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public AdsGetDemographicsQuery(VkApiClient client, UserActor actor) {
super(client, "ads.getDemographics", Utils.buildParametrizedType(List.class, GetDemographicsResponse.class));
accessToken(actor.getAccessToken());
}
/**
* Advertising account ID.
*
* @param value value of "account id" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("account_id")
public AdsGetDemographicsQuery accountId(Integer value) {
return unsafeParam("account_id", value);
}
/**
* Type of requested objects listed in 'ids' parameter: *ad - ads,, *campaign - campaigns.
*
* @param value value of "ids type" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("ids_type")
public AdsGetDemographicsQuery idsType(GetDemographicsIdsType value) {
return unsafeParam("ids_type", value);
}
/**
* IDs requested ads or campaigns, separated with a comma, depending on the value set in 'ids_type'. Maximum 2000 objects.
*
* @param value value of "ids" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("ids")
public AdsGetDemographicsQuery ids(String value) {
return unsafeParam("ids", value);
}
/**
* Data grouping by dates: *day - statistics by days,, *month - statistics by months,, *overall - overall statistics. 'date_from' and 'date_to' parameters set temporary limits.
*
* @param value value of "period" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("period")
public AdsGetDemographicsQuery period(GetDemographicsPeriod value) {
return unsafeParam("period", value);
}
/**
* Date to show statistics from. For different value of 'period' different date format is used: *day: YYYY-MM-DD, example: 2011-09-27 - September 27, 2011, **0 - day it was created on,, *month: YYYY-MM, example: 2011-09 - September 2011, **0 - month it was created in,, *overall: 0.
*
* @param value value of "date from" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("date_from")
public AdsGetDemographicsQuery dateFrom(String value) {
return unsafeParam("date_from", value);
}
/**
* Date to show statistics to. For different value of 'period' different date format is used: *day: YYYY-MM-DD, example: 2011-09-27 - September 27, 2011, **0 - current day,, *month: YYYY-MM, example: 2011-09 - September 2011, **0 - current month,, *overall: 0.
*
* @param value value of "date to" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("date_to")
public AdsGetDemographicsQuery dateTo(String value) {
return unsafeParam("date_to", value);
}
@Override
protected AdsGetDemographicsQuery getThis() {
return this;
}
@Override
protected List essentialKeys() {
return Arrays.asList("ids_type", "date_from", "period", "ids", "account_id", "access_token", "date_to");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy