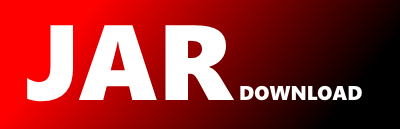
com.vk.api.sdk.queries.board.BoardAddTopicQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.queries.board;
import com.vk.api.sdk.client.AbstractQueryBuilder;
import com.vk.api.sdk.client.VkApiClient;
import com.vk.api.sdk.client.actors.UserActor;
import com.vk.api.sdk.objects.annotations.ApiParam;
import java.util.Arrays;
import java.util.List;
/**
* Query for Board.addTopic method
*/
public class BoardAddTopicQuery extends AbstractQueryBuilder {
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param groupId value of "group id" parameter. Minimum is 1. Entity - owner
*
* @param title value of "title" parameter.
*/
public BoardAddTopicQuery(VkApiClient client, UserActor actor, Long groupId, String title) {
super(client, "board.addTopic", Integer.class);
accessToken(actor.getAccessToken());
groupId(groupId);
title(title);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public BoardAddTopicQuery(VkApiClient client, UserActor actor) {
super(client, "board.addTopic", Integer.class);
accessToken(actor.getAccessToken());
}
/**
* ID of the community that owns the discussion board.
*
* @param value value of "group id" parameter. Minimum is 1. Entity - owner
*
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("group_id")
public BoardAddTopicQuery groupId(Long value) {
return unsafeParam("group_id", value);
}
/**
* Topic title.
*
* @param value value of "title" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("title")
public BoardAddTopicQuery title(String value) {
return unsafeParam("title", value);
}
/**
* Text of the topic.
*
* @param value value of "text" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("text")
public BoardAddTopicQuery text(String value) {
return unsafeParam("text", value);
}
/**
* For a community: '1' - to post the topic as by the community, '0' - to post the topic as by the user (default)
*
* @param value value of "from group" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("from_group")
public BoardAddTopicQuery fromGroup(Boolean value) {
return unsafeParam("from_group", value);
}
/**
* attachments
* List of media objects attached to the topic, in the following format: "_,_", ' - Type of media object: 'photo' - photo, 'video' - video, 'audio' - audio, 'doc' - document, '' - ID of the media owner. '' - Media ID. Example: "photo100172_166443618,photo66748_265827614", , "NOTE: If you try to attach more than one reference, an error will be thrown.",
*
* @param value value of "attachments" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("attachments")
public BoardAddTopicQuery attachments(String... value) {
return unsafeParam("attachments", value);
}
/**
* List of media objects attached to the topic, in the following format: "_,_", ' - Type of media object: 'photo' - photo, 'video' - video, 'audio' - audio, 'doc' - document, '' - ID of the media owner. '' - Media ID. Example: "photo100172_166443618,photo66748_265827614", , "NOTE: If you try to attach more than one reference, an error will be thrown.",
*
* @param value value of "attachments" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("attachments")
public BoardAddTopicQuery attachments(List value) {
return unsafeParam("attachments", value);
}
@Override
protected BoardAddTopicQuery getThis() {
return this;
}
@Override
protected List essentialKeys() {
return Arrays.asList("group_id", "title", "access_token");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy