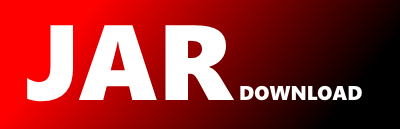
com.vk.api.sdk.queries.board.BoardDeleteCommentQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.queries.board;
import com.vk.api.sdk.client.AbstractQueryBuilder;
import com.vk.api.sdk.client.VkApiClient;
import com.vk.api.sdk.client.actors.GroupActor;
import com.vk.api.sdk.client.actors.UserActor;
import com.vk.api.sdk.objects.annotations.ApiParam;
import com.vk.api.sdk.objects.base.responses.OkResponse;
import java.util.Arrays;
import java.util.List;
/**
* Query for Board.deleteComment method
*/
public class BoardDeleteCommentQuery extends AbstractQueryBuilder {
/**
* Creates a AbstractQueryBuilder instance that can be used to build group api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param groupId value of "group id" parameter. Minimum is 1. Entity - owner
*
* @param topicId value of "topic id" parameter. Minimum is 1.
* @param commentId value of "comment id" parameter. Minimum is 1.
*/
public BoardDeleteCommentQuery(VkApiClient client, GroupActor actor, Long groupId,
Integer topicId, Integer commentId) {
super(client, "board.deleteComment", OkResponse.class);
accessToken(actor.getAccessToken());
groupId(actor.getGroupId());
groupId(groupId);
topicId(topicId);
commentId(commentId);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build group api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public BoardDeleteCommentQuery(VkApiClient client, GroupActor actor) {
super(client, "board.deleteComment", OkResponse.class);
accessToken(actor.getAccessToken());
groupId(actor.getGroupId());
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param groupId value of "group id" parameter. Minimum is 1. Entity - owner
*
* @param topicId value of "topic id" parameter. Minimum is 1.
* @param commentId value of "comment id" parameter. Minimum is 1.
*/
public BoardDeleteCommentQuery(VkApiClient client, UserActor actor, Long groupId,
Integer topicId, Integer commentId) {
super(client, "board.deleteComment", OkResponse.class);
accessToken(actor.getAccessToken());
groupId(groupId);
topicId(topicId);
commentId(commentId);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public BoardDeleteCommentQuery(VkApiClient client, UserActor actor) {
super(client, "board.deleteComment", OkResponse.class);
accessToken(actor.getAccessToken());
}
/**
* ID of the community that owns the discussion board.
*
* @param value value of "group id" parameter. Minimum is 1. Entity - owner
*
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("group_id")
public BoardDeleteCommentQuery groupId(Long value) {
return unsafeParam("group_id", value);
}
/**
* Topic ID.
*
* @param value value of "topic id" parameter. Minimum is 1.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("topic_id")
public BoardDeleteCommentQuery topicId(Integer value) {
return unsafeParam("topic_id", value);
}
/**
* Comment ID.
*
* @param value value of "comment id" parameter. Minimum is 1.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("comment_id")
public BoardDeleteCommentQuery commentId(Integer value) {
return unsafeParam("comment_id", value);
}
@Override
protected BoardDeleteCommentQuery getThis() {
return this;
}
@Override
protected List essentialKeys() {
return Arrays.asList("group_id", "topic_id", "comment_id", "access_token");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy