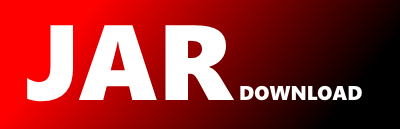
com.vk.api.sdk.queries.docs.DocsSaveQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.queries.docs;
import com.vk.api.sdk.client.AbstractQueryBuilder;
import com.vk.api.sdk.client.VkApiClient;
import com.vk.api.sdk.client.actors.GroupActor;
import com.vk.api.sdk.client.actors.UserActor;
import com.vk.api.sdk.objects.annotations.ApiParam;
import com.vk.api.sdk.objects.docs.responses.SaveResponse;
import java.util.Arrays;
import java.util.List;
/**
* Query for Docs.save method
*/
public class DocsSaveQuery extends AbstractQueryBuilder {
/**
* Creates a AbstractQueryBuilder instance that can be used to build group api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param file value of "file" parameter.
*/
public DocsSaveQuery(VkApiClient client, GroupActor actor, String file) {
super(client, "docs.save", SaveResponse.class);
accessToken(actor.getAccessToken());
file(file);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build group api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public DocsSaveQuery(VkApiClient client, GroupActor actor) {
super(client, "docs.save", SaveResponse.class);
accessToken(actor.getAccessToken());
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
* @param file value of "file" parameter.
*/
public DocsSaveQuery(VkApiClient client, UserActor actor, String file) {
super(client, "docs.save", SaveResponse.class);
accessToken(actor.getAccessToken());
file(file);
}
/**
* Creates a AbstractQueryBuilder instance that can be used to build user api request with various parameters
*
* @param client VK API client
* @param actor actor with access token
*/
public DocsSaveQuery(VkApiClient client, UserActor actor) {
super(client, "docs.save", SaveResponse.class);
accessToken(actor.getAccessToken());
}
/**
* This parameter is returned when the file is [vk.com/dev/upload_files_2|uploaded to the server].
*
* @param value value of "file" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("file")
public DocsSaveQuery file(String value) {
return unsafeParam("file", value);
}
/**
* Document title.
*
* @param value value of "title" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("title")
public DocsSaveQuery title(String value) {
return unsafeParam("title", value);
}
/**
* Document tags.
*
* @param value value of "tags" parameter.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("tags")
public DocsSaveQuery tags(String value) {
return unsafeParam("tags", value);
}
/**
* Set return tags
*
* @param value value of "return tags" parameter. By default false.
* @return a reference to this {@code AbstractQueryBuilder} object to fulfill the "Builder" pattern.
*/
@ApiParam("return_tags")
public DocsSaveQuery returnTags(Boolean value) {
return unsafeParam("return_tags", value);
}
@Override
protected DocsSaveQuery getThis() {
return this;
}
@Override
protected List essentialKeys() {
return Arrays.asList("file", "access_token");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy