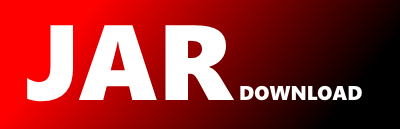
com.vk.api.sdk.actions.Storage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
The newest version!
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.actions;
import com.vk.api.sdk.client.AbstractAction;
import com.vk.api.sdk.client.VkApiClient;
import com.vk.api.sdk.client.actors.GroupActor;
import com.vk.api.sdk.client.actors.ServiceActor;
import com.vk.api.sdk.client.actors.UserActor;
import com.vk.api.sdk.objects.annotations.ApiMethod;
import com.vk.api.sdk.queries.storage.StorageGetKeysQuery;
import com.vk.api.sdk.queries.storage.StorageGetQuery;
import com.vk.api.sdk.queries.storage.StorageSetQuery;
/**
* List of Storage methods
*/
public class Storage extends AbstractAction {
/**
* Constructor
*
* @param client vk api client
*/
public Storage(VkApiClient client) {
super(client);
}
/**
* Returns a value of variable with the name set by key parameter.
*
* @param actor vk user actor
* @return query
*/
@ApiMethod("storage.get")
public StorageGetQuery get(UserActor actor) {
return new StorageGetQuery(getClient(), actor);
}
/**
* Returns a value of variable with the name set by key parameter.
*
* @param actor vk group actor
* @return query
*/
@ApiMethod("storage.get")
public StorageGetQuery get(GroupActor actor) {
return new StorageGetQuery(getClient(), actor);
}
/**
* Returns a value of variable with the name set by key parameter.
*
* @param actor vk service actor
* @return query
*/
@ApiMethod("storage.get")
public StorageGetQuery get(ServiceActor actor) {
return new StorageGetQuery(getClient(), actor);
}
/**
* Returns the names of all variables.
*
* @param actor vk user actor
* @return query
*/
@ApiMethod("storage.getKeys")
public StorageGetKeysQuery getKeys(UserActor actor) {
return new StorageGetKeysQuery(getClient(), actor);
}
/**
* Returns the names of all variables.
*
* @param actor vk group actor
* @return query
*/
@ApiMethod("storage.getKeys")
public StorageGetKeysQuery getKeys(GroupActor actor) {
return new StorageGetKeysQuery(getClient(), actor);
}
/**
* Returns the names of all variables.
*
* @param actor vk service actor
* @return query
*/
@ApiMethod("storage.getKeys")
public StorageGetKeysQuery getKeys(ServiceActor actor) {
return new StorageGetKeysQuery(getClient(), actor);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk user actor
* @param key
* @return query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(UserActor actor, String key) {
return new StorageSetQuery(getClient(), actor, key);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk user actor
* @return only actor query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(UserActor actor) {
return new StorageSetQuery(getClient(), actor);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk group actor
* @param key
* @return query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(GroupActor actor, String key) {
return new StorageSetQuery(getClient(), actor, key);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk group actor
* @return only actor query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(GroupActor actor) {
return new StorageSetQuery(getClient(), actor);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk service actor
* @param key
* @return query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(ServiceActor actor, String key) {
return new StorageSetQuery(getClient(), actor, key);
}
/**
* Saves a value of variable with the name set by 'key' parameter.
*
* @param actor vk service actor
* @return only actor query
*/
@ApiMethod("storage.set")
public StorageSetQuery set(ServiceActor actor) {
return new StorageSetQuery(getClient(), actor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy