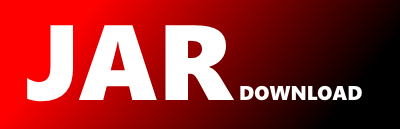
com.vk.api.sdk.objects.ads.TargetGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
The newest version!
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.objects.ads;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.vk.api.sdk.objects.Validable;
import java.util.List;
import java.util.Objects;
/**
* TargetGroup object
*/
public class TargetGroup implements Validable {
/**
* API source
*/
@SerializedName("api_source")
private Boolean apiSource;
/**
* Audience
*/
@SerializedName("audience_count")
private Integer audienceCount;
/**
* Site domain
*/
@SerializedName("domain")
private String domain;
/**
* File source
*/
@SerializedName("file_source")
private Boolean fileSource;
/**
* Group ID
*/
@SerializedName("id")
private Integer id;
/**
* Is audience
*/
@SerializedName("is_audience")
private Boolean isAudience;
/**
* Is shared
*/
@SerializedName("is_shared")
private Boolean isShared;
/**
* Last updated
*/
@SerializedName("last_updated")
private Integer lastUpdated;
/**
* Number of days for user to be in group
*/
@SerializedName("lifetime")
private Integer lifetime;
/**
* File source
*/
@SerializedName("lookalike_source")
private Boolean lookalikeSource;
/**
* Group name
*/
@SerializedName("name")
private String name;
/**
* Pixel code
*/
@SerializedName("pixel")
private String pixel;
/**
* Target Pixel id
*/
@SerializedName("target_pixel_id")
private Integer targetPixelId;
/**
* Target Pixel rules
*/
@SerializedName("target_pixel_rules")
private List targetPixelRules;
public Boolean getApiSource() {
return apiSource;
}
public TargetGroup setApiSource(Boolean apiSource) {
this.apiSource = apiSource;
return this;
}
public Integer getAudienceCount() {
return audienceCount;
}
public TargetGroup setAudienceCount(Integer audienceCount) {
this.audienceCount = audienceCount;
return this;
}
public String getDomain() {
return domain;
}
public TargetGroup setDomain(String domain) {
this.domain = domain;
return this;
}
public Boolean getFileSource() {
return fileSource;
}
public TargetGroup setFileSource(Boolean fileSource) {
this.fileSource = fileSource;
return this;
}
public Integer getId() {
return id;
}
public TargetGroup setId(Integer id) {
this.id = id;
return this;
}
public Boolean getIsAudience() {
return isAudience;
}
public TargetGroup setIsAudience(Boolean isAudience) {
this.isAudience = isAudience;
return this;
}
public Boolean getIsShared() {
return isShared;
}
public TargetGroup setIsShared(Boolean isShared) {
this.isShared = isShared;
return this;
}
public Integer getLastUpdated() {
return lastUpdated;
}
public TargetGroup setLastUpdated(Integer lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
public Integer getLifetime() {
return lifetime;
}
public TargetGroup setLifetime(Integer lifetime) {
this.lifetime = lifetime;
return this;
}
public Boolean getLookalikeSource() {
return lookalikeSource;
}
public TargetGroup setLookalikeSource(Boolean lookalikeSource) {
this.lookalikeSource = lookalikeSource;
return this;
}
public String getName() {
return name;
}
public TargetGroup setName(String name) {
this.name = name;
return this;
}
public String getPixel() {
return pixel;
}
public TargetGroup setPixel(String pixel) {
this.pixel = pixel;
return this;
}
public Integer getTargetPixelId() {
return targetPixelId;
}
public TargetGroup setTargetPixelId(Integer targetPixelId) {
this.targetPixelId = targetPixelId;
return this;
}
public List getTargetPixelRules() {
return targetPixelRules;
}
public TargetGroup setTargetPixelRules(List targetPixelRules) {
this.targetPixelRules = targetPixelRules;
return this;
}
@Override
public int hashCode() {
return Objects.hash(apiSource, lifetime, fileSource, isAudience, lastUpdated, targetPixelId, domain, audienceCount, name, targetPixelRules, id, lookalikeSource, pixel, isShared);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TargetGroup targetGroup = (TargetGroup) o;
return Objects.equals(apiSource, targetGroup.apiSource) &&
Objects.equals(lastUpdated, targetGroup.lastUpdated) &&
Objects.equals(targetPixelRules, targetGroup.targetPixelRules) &&
Objects.equals(lifetime, targetGroup.lifetime) &&
Objects.equals(fileSource, targetGroup.fileSource) &&
Objects.equals(targetPixelId, targetGroup.targetPixelId) &&
Objects.equals(isAudience, targetGroup.isAudience) &&
Objects.equals(domain, targetGroup.domain) &&
Objects.equals(isShared, targetGroup.isShared) &&
Objects.equals(lookalikeSource, targetGroup.lookalikeSource) &&
Objects.equals(audienceCount, targetGroup.audienceCount) &&
Objects.equals(name, targetGroup.name) &&
Objects.equals(id, targetGroup.id) &&
Objects.equals(pixel, targetGroup.pixel);
}
@Override
public String toString() {
final Gson gson = new Gson();
return gson.toJson(this);
}
public String toPrettyString() {
final StringBuilder sb = new StringBuilder("TargetGroup{");
sb.append("apiSource=").append(apiSource);
sb.append(", lastUpdated=").append(lastUpdated);
sb.append(", targetPixelRules=").append(targetPixelRules);
sb.append(", lifetime=").append(lifetime);
sb.append(", fileSource=").append(fileSource);
sb.append(", targetPixelId=").append(targetPixelId);
sb.append(", isAudience=").append(isAudience);
sb.append(", domain='").append(domain).append("'");
sb.append(", isShared=").append(isShared);
sb.append(", lookalikeSource=").append(lookalikeSource);
sb.append(", audienceCount=").append(audienceCount);
sb.append(", name='").append(name).append("'");
sb.append(", id=").append(id);
sb.append(", pixel='").append(pixel).append("'");
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy