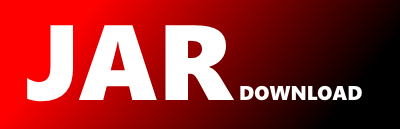
com.vk.api.sdk.objects.video.Video Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java library for VK API interaction, includes OAuth 2.0 authorization and API methods.
The newest version!
// Autogenerated from vk-api-schema. Please don't edit it manually.
package com.vk.api.sdk.objects.video;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.vk.api.sdk.objects.Validable;
import com.vk.api.sdk.objects.base.BoolInt;
import com.vk.api.sdk.objects.base.Likes;
import com.vk.api.sdk.objects.base.PropertyExists;
import com.vk.api.sdk.objects.base.RepostsInfo;
import java.net.URI;
import java.util.List;
import java.util.Objects;
/**
* Video object
*/
public class Video implements Validable {
/**
* Video access key
*/
@SerializedName("access_key")
private String accessKey;
/**
* 1 if video is added to user's albums
*/
@SerializedName("added")
private BoolInt added;
/**
* Date when the video has been added in Unixtime
*/
@SerializedName("adding_date")
private Integer addingDate;
/**
* Live donations balance
*/
@SerializedName("balance")
private Integer balance;
/**
* Information whether current user can add the video
*/
@SerializedName("can_add")
private BoolInt canAdd;
/**
* Information whether current user can add the video to favourites
*/
@SerializedName("can_add_to_faves")
private BoolInt canAddToFaves;
/**
* Information whether current user can attach action button to the video
*/
@SerializedName("can_attach_link")
private BoolInt canAttachLink;
/**
* Information whether current user can comment the video
*/
@SerializedName("can_comment")
private BoolInt canComment;
/**
* Information whether current user can delete the video
*/
@SerializedName("can_delete")
private BoolInt canDelete;
/**
* Information whether current user can edit the video
*/
@SerializedName("can_edit")
private BoolInt canEdit;
/**
* Information whether current user can edit the video privacy
*/
@SerializedName("can_edit_privacy")
private BoolInt canEditPrivacy;
/**
* Information whether current user can like the video
*/
@SerializedName("can_like")
private BoolInt canLike;
/**
* Information whether current user can repost the video
*/
@SerializedName("can_repost")
private Integer canRepost;
/**
* Information whether current user can subscribe to author of the video
*/
@SerializedName("can_subscribe")
private BoolInt canSubscribe;
/**
* Number of comments
*/
@SerializedName("comments")
private Integer comments;
/**
* Restriction code
*/
@SerializedName("content_restricted")
private Integer contentRestricted;
/**
* Restriction text
*/
@SerializedName("content_restricted_message")
private String contentRestrictedMessage;
/**
* 1 if video is being converted
*/
@SerializedName("converting")
private BoolInt converting;
/**
* Date when video has been uploaded in Unixtime
*/
@SerializedName("date")
private Integer date;
/**
* Video description
*/
@SerializedName("description")
private String description;
/**
* Video duration in seconds
*/
@SerializedName("duration")
private Integer duration;
@SerializedName("first_frame")
private List firstFrame;
/**
* Video height
*/
@SerializedName("height")
private Integer height;
/**
* Video ID
*/
@SerializedName("id")
private Integer id;
@SerializedName("image")
private List image;
/**
* Whether video is added to bookmarks
*/
@SerializedName("is_favorite")
private Boolean isFavorite;
/**
* 1 if video is private
*/
@SerializedName("is_private")
private BoolInt isPrivate;
/**
* 1 if user is subscribed to author of the video
*/
@SerializedName("is_subscribed")
private BoolInt isSubscribed;
@SerializedName("likes")
private Likes likes;
/**
* 1 if the video is a live stream
*/
@SerializedName("live")
private PropertyExists live;
/**
* Whether current user is subscribed to the upcoming live stream notification (if not subscribed to the author)
*/
@SerializedName("live_notify")
private BoolInt liveNotify;
/**
* Date in Unixtime when the live stream is scheduled to start by the author
*/
@SerializedName("live_start_time")
private Integer liveStartTime;
/**
* If video is external, number of views on vk
*/
@SerializedName("local_views")
private Integer localViews;
/**
* Video owner ID
* Entity: owner
*/
@SerializedName("owner_id")
private Long ownerId;
/**
* External platform
*/
@SerializedName("platform")
private String platform;
/**
* Video embed URL
*/
@SerializedName("player")
private URI player;
/**
* Returns if the video is processing
*/
@SerializedName("processing")
private PropertyExists processing;
/**
* Information whether the video is repeated
*/
@SerializedName("repeat")
private PropertyExists repeat;
@SerializedName("reposts")
private RepostsInfo reposts;
@SerializedName("response_type")
private VideoResponseType responseType;
/**
* Number of spectators of the stream
*/
@SerializedName("spectators")
private Integer spectators;
/**
* Video title
*/
@SerializedName("title")
private String title;
@SerializedName("track_code")
private String trackCode;
@SerializedName("type")
private VideoType type;
/**
* 1 if the video is an upcoming stream
*/
@SerializedName("upcoming")
private PropertyExists upcoming;
/**
* Id of the user who uploaded the video if it was uploaded to a group by member
* Entity: owner
*/
@SerializedName("user_id")
private Long userId;
/**
* Number of views
*/
@SerializedName("views")
private Integer views;
/**
* Video width
*/
@SerializedName("width")
private Integer width;
public String getAccessKey() {
return accessKey;
}
public Video setAccessKey(String accessKey) {
this.accessKey = accessKey;
return this;
}
public boolean isAdded() {
return added == BoolInt.YES;
}
public BoolInt getAdded() {
return added;
}
public Integer getAddingDate() {
return addingDate;
}
public Video setAddingDate(Integer addingDate) {
this.addingDate = addingDate;
return this;
}
public Integer getBalance() {
return balance;
}
public Video setBalance(Integer balance) {
this.balance = balance;
return this;
}
public boolean canAdd() {
return canAdd == BoolInt.YES;
}
public BoolInt getCanAdd() {
return canAdd;
}
public boolean canAddToFaves() {
return canAddToFaves == BoolInt.YES;
}
public BoolInt getCanAddToFaves() {
return canAddToFaves;
}
public boolean canAttachLink() {
return canAttachLink == BoolInt.YES;
}
public BoolInt getCanAttachLink() {
return canAttachLink;
}
public boolean canComment() {
return canComment == BoolInt.YES;
}
public BoolInt getCanComment() {
return canComment;
}
public boolean canDelete() {
return canDelete == BoolInt.YES;
}
public BoolInt getCanDelete() {
return canDelete;
}
public boolean canEdit() {
return canEdit == BoolInt.YES;
}
public BoolInt getCanEdit() {
return canEdit;
}
public boolean canEditPrivacy() {
return canEditPrivacy == BoolInt.YES;
}
public BoolInt getCanEditPrivacy() {
return canEditPrivacy;
}
public boolean canLike() {
return canLike == BoolInt.YES;
}
public BoolInt getCanLike() {
return canLike;
}
public Integer getCanRepost() {
return canRepost;
}
public Video setCanRepost(Integer canRepost) {
this.canRepost = canRepost;
return this;
}
public boolean canSubscribe() {
return canSubscribe == BoolInt.YES;
}
public BoolInt getCanSubscribe() {
return canSubscribe;
}
public Integer getComments() {
return comments;
}
public Video setComments(Integer comments) {
this.comments = comments;
return this;
}
public Integer getContentRestricted() {
return contentRestricted;
}
public Video setContentRestricted(Integer contentRestricted) {
this.contentRestricted = contentRestricted;
return this;
}
public String getContentRestrictedMessage() {
return contentRestrictedMessage;
}
public Video setContentRestrictedMessage(String contentRestrictedMessage) {
this.contentRestrictedMessage = contentRestrictedMessage;
return this;
}
public boolean isConverting() {
return converting == BoolInt.YES;
}
public BoolInt getConverting() {
return converting;
}
public Integer getDate() {
return date;
}
public Video setDate(Integer date) {
this.date = date;
return this;
}
public String getDescription() {
return description;
}
public Video setDescription(String description) {
this.description = description;
return this;
}
public Integer getDuration() {
return duration;
}
public Video setDuration(Integer duration) {
this.duration = duration;
return this;
}
public List getFirstFrame() {
return firstFrame;
}
public Video setFirstFrame(List firstFrame) {
this.firstFrame = firstFrame;
return this;
}
public Integer getHeight() {
return height;
}
public Video setHeight(Integer height) {
this.height = height;
return this;
}
public Integer getId() {
return id;
}
public Video setId(Integer id) {
this.id = id;
return this;
}
public List getImage() {
return image;
}
public Video setImage(List image) {
this.image = image;
return this;
}
public Boolean getIsFavorite() {
return isFavorite;
}
public Video setIsFavorite(Boolean isFavorite) {
this.isFavorite = isFavorite;
return this;
}
public boolean isPrivate() {
return isPrivate == BoolInt.YES;
}
public BoolInt getIsPrivate() {
return isPrivate;
}
public boolean isSubscribed() {
return isSubscribed == BoolInt.YES;
}
public BoolInt getIsSubscribed() {
return isSubscribed;
}
public Likes getLikes() {
return likes;
}
public Video setLikes(Likes likes) {
this.likes = likes;
return this;
}
public boolean isLive() {
return live == PropertyExists.PROPERTY_EXISTS;
}
public boolean isLiveNotify() {
return liveNotify == BoolInt.YES;
}
public BoolInt getLiveNotify() {
return liveNotify;
}
public Integer getLiveStartTime() {
return liveStartTime;
}
public Video setLiveStartTime(Integer liveStartTime) {
this.liveStartTime = liveStartTime;
return this;
}
public Integer getLocalViews() {
return localViews;
}
public Video setLocalViews(Integer localViews) {
this.localViews = localViews;
return this;
}
public Long getOwnerId() {
return ownerId;
}
public Video setOwnerId(Long ownerId) {
this.ownerId = ownerId;
return this;
}
public String getPlatform() {
return platform;
}
public Video setPlatform(String platform) {
this.platform = platform;
return this;
}
public URI getPlayer() {
return player;
}
public Video setPlayer(URI player) {
this.player = player;
return this;
}
public boolean isProcessing() {
return processing == PropertyExists.PROPERTY_EXISTS;
}
public boolean isRepeat() {
return repeat == PropertyExists.PROPERTY_EXISTS;
}
public RepostsInfo getReposts() {
return reposts;
}
public Video setReposts(RepostsInfo reposts) {
this.reposts = reposts;
return this;
}
public VideoResponseType getResponseType() {
return responseType;
}
public Video setResponseType(VideoResponseType responseType) {
this.responseType = responseType;
return this;
}
public Integer getSpectators() {
return spectators;
}
public Video setSpectators(Integer spectators) {
this.spectators = spectators;
return this;
}
public String getTitle() {
return title;
}
public Video setTitle(String title) {
this.title = title;
return this;
}
public String getTrackCode() {
return trackCode;
}
public Video setTrackCode(String trackCode) {
this.trackCode = trackCode;
return this;
}
public VideoType getType() {
return type;
}
public Video setType(VideoType type) {
this.type = type;
return this;
}
public boolean isUpcoming() {
return upcoming == PropertyExists.PROPERTY_EXISTS;
}
public Long getUserId() {
return userId;
}
public Video setUserId(Long userId) {
this.userId = userId;
return this;
}
public Integer getViews() {
return views;
}
public Video setViews(Integer views) {
this.views = views;
return this;
}
public Integer getWidth() {
return width;
}
public Video setWidth(Integer width) {
this.width = width;
return this;
}
@Override
public int hashCode() {
return Objects.hash(date, added, converting, canLike, canEdit, canAdd, canAddToFaves, description, canEditPrivacy, isPrivate, ownerId, liveNotify, title, type, liveStartTime, platform, duration, canSubscribe, responseType, isSubscribed, balance, firstFrame, repeat, canDelete, canComment, id, addingDate, live, views, height, likes, player, trackCode, image, comments, contentRestrictedMessage, canRepost, contentRestricted, spectators, userId, localViews, canAttachLink, accessKey, width, processing, reposts, upcoming, isFavorite);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Video video = (Video) o;
return Objects.equals(date, video.date) &&
Objects.equals(isPrivate, video.isPrivate) &&
Objects.equals(isFavorite, video.isFavorite) &&
Objects.equals(addingDate, video.addingDate) &&
Objects.equals(canAddToFaves, video.canAddToFaves) &&
Objects.equals(added, video.added) &&
Objects.equals(converting, video.converting) &&
Objects.equals(ownerId, video.ownerId) &&
Objects.equals(liveNotify, video.liveNotify) &&
Objects.equals(contentRestrictedMessage, video.contentRestrictedMessage) &&
Objects.equals(description, video.description) &&
Objects.equals(responseType, video.responseType) &&
Objects.equals(canAttachLink, video.canAttachLink) &&
Objects.equals(title, video.title) &&
Objects.equals(type, video.type) &&
Objects.equals(platform, video.platform) &&
Objects.equals(duration, video.duration) &&
Objects.equals(canComment, video.canComment) &&
Objects.equals(localViews, video.localViews) &&
Objects.equals(balance, video.balance) &&
Objects.equals(canLike, video.canLike) &&
Objects.equals(canDelete, video.canDelete) &&
Objects.equals(repeat, video.repeat) &&
Objects.equals(id, video.id) &&
Objects.equals(live, video.live) &&
Objects.equals(views, video.views) &&
Objects.equals(height, video.height) &&
Objects.equals(likes, video.likes) &&
Objects.equals(player, video.player) &&
Objects.equals(liveStartTime, video.liveStartTime) &&
Objects.equals(image, video.image) &&
Objects.equals(comments, video.comments) &&
Objects.equals(contentRestricted, video.contentRestricted) &&
Objects.equals(canEdit, video.canEdit) &&
Objects.equals(spectators, video.spectators) &&
Objects.equals(canSubscribe, video.canSubscribe) &&
Objects.equals(canRepost, video.canRepost) &&
Objects.equals(canEditPrivacy, video.canEditPrivacy) &&
Objects.equals(userId, video.userId) &&
Objects.equals(accessKey, video.accessKey) &&
Objects.equals(width, video.width) &&
Objects.equals(processing, video.processing) &&
Objects.equals(isSubscribed, video.isSubscribed) &&
Objects.equals(trackCode, video.trackCode) &&
Objects.equals(canAdd, video.canAdd) &&
Objects.equals(reposts, video.reposts) &&
Objects.equals(upcoming, video.upcoming) &&
Objects.equals(firstFrame, video.firstFrame);
}
@Override
public String toString() {
final Gson gson = new Gson();
return gson.toJson(this);
}
public String toPrettyString() {
final StringBuilder sb = new StringBuilder("Video{");
sb.append("date=").append(date);
sb.append(", isPrivate=").append(isPrivate);
sb.append(", isFavorite=").append(isFavorite);
sb.append(", addingDate=").append(addingDate);
sb.append(", canAddToFaves=").append(canAddToFaves);
sb.append(", added=").append(added);
sb.append(", converting=").append(converting);
sb.append(", ownerId=").append(ownerId);
sb.append(", liveNotify=").append(liveNotify);
sb.append(", contentRestrictedMessage='").append(contentRestrictedMessage).append("'");
sb.append(", description='").append(description).append("'");
sb.append(", responseType='").append(responseType).append("'");
sb.append(", canAttachLink=").append(canAttachLink);
sb.append(", title='").append(title).append("'");
sb.append(", type='").append(type).append("'");
sb.append(", platform='").append(platform).append("'");
sb.append(", duration=").append(duration);
sb.append(", canComment=").append(canComment);
sb.append(", localViews=").append(localViews);
sb.append(", balance=").append(balance);
sb.append(", canLike=").append(canLike);
sb.append(", canDelete=").append(canDelete);
sb.append(", repeat=").append(repeat);
sb.append(", id=").append(id);
sb.append(", live=").append(live);
sb.append(", views=").append(views);
sb.append(", height=").append(height);
sb.append(", likes=").append(likes);
sb.append(", player=").append(player);
sb.append(", liveStartTime=").append(liveStartTime);
sb.append(", image=").append(image);
sb.append(", comments=").append(comments);
sb.append(", contentRestricted=").append(contentRestricted);
sb.append(", canEdit=").append(canEdit);
sb.append(", spectators=").append(spectators);
sb.append(", canSubscribe=").append(canSubscribe);
sb.append(", canRepost=").append(canRepost);
sb.append(", canEditPrivacy=").append(canEditPrivacy);
sb.append(", userId=").append(userId);
sb.append(", accessKey='").append(accessKey).append("'");
sb.append(", width=").append(width);
sb.append(", processing=").append(processing);
sb.append(", isSubscribed=").append(isSubscribed);
sb.append(", trackCode='").append(trackCode).append("'");
sb.append(", canAdd=").append(canAdd);
sb.append(", reposts=").append(reposts);
sb.append(", upcoming=").append(upcoming);
sb.append(", firstFrame=").append(firstFrame);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy