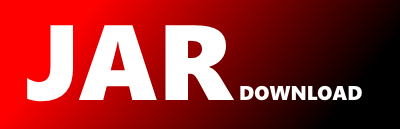
com.vladsch.boxed.json.BoxedJson Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boxed-json Show documentation
Show all versions of boxed-json Show documentation
Mutable JSON classes with easy search and manipulation in Java
package com.vladsch.boxed.json;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.json.*;
import java.io.InputStream;
import java.io.Reader;
import java.io.StringReader;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import static java.lang.Character.isDigit;
public class BoxedJson {
public static BoxedJsValue boxedOf(JsonValue jsonValue) {
if (jsonValue instanceof BoxedJsValue) return (BoxedJsValue) jsonValue;
if (jsonValue == null) {
return new BoxedJsValueLiteral(JsonValue.NULL);
}
switch (jsonValue.getValueType()) {
case ARRAY:
return new BoxedJsArrayImpl((JsonArray) jsonValue);
case OBJECT:
return new BoxedJsObjectImpl((JsonObject) jsonValue);
case STRING:
return new BoxedJsStringLiteral((JsonString) jsonValue);
case NUMBER:
return new BoxedJsNumberLiteral((JsonNumber) jsonValue);
case TRUE:
case FALSE:
case NULL:
default:
return new BoxedJsValueLiteral(jsonValue);
}
}
public static BoxedJsArray boxedOf(final JsonArray jsonValue) {
return jsonValue instanceof BoxedJsArray ? (BoxedJsArray) jsonValue : jsonValue == null ? BoxedJsObject.HAD_NULL_ARRAY : new BoxedJsArrayImpl(jsonValue);
}
public static BoxedJsObject boxedOf(final JsonObject jsonValue) {
return jsonValue instanceof BoxedJsObject ? (BoxedJsObject) jsonValue : jsonValue == null ? BoxedJsObject.HAD_NULL_OBJECT : new BoxedJsObjectImpl(jsonValue);
}
public static BoxedJsNumber boxedOf(final JsonNumber jsonValue) {
return jsonValue instanceof BoxedJsNumber ? (BoxedJsNumber) jsonValue : jsonValue == null ? BoxedJsObject.HAD_NULL_NUMBER : new BoxedJsNumberLiteral(jsonValue);
}
public static BoxedJsString boxedOf(final JsonString jsonValue) {
return jsonValue instanceof BoxedJsString ? (BoxedJsString) jsonValue : new BoxedJsStringLiteral(jsonValue);
}
public static BoxedJsValue boxedOf(final boolean jsonValue) {
return new BoxedJsValueLiteral(jsonValue);
}
public static BoxedJsNumber boxedOf(final int jsonValue) {
return new BoxedJsNumberLiteral(JsNumber.of(jsonValue));
}
public static BoxedJsNumber boxedOf(final long jsonValue) {
return new BoxedJsNumberLiteral(JsNumber.of(jsonValue));
}
public static BoxedJsNumber boxedOf(final BigInteger jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_NUMBER : new BoxedJsNumberLiteral(JsNumber.of(jsonValue));
}
public static BoxedJsNumber boxedOf(final double jsonValue) {
return new BoxedJsNumberLiteral(JsNumber.of(jsonValue));
}
public static BoxedJsNumber boxedOf(final BigDecimal jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_NUMBER : new BoxedJsNumberLiteral(JsNumber.of(jsonValue));
}
public static BoxedJsString boxedOf(final String jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_STRING : new BoxedJsStringLiteral(JsString.of(jsonValue));
}
public static BoxedJsObject boxedFrom(final @NotNull Reader json) {
return boxedOf(Json.createReader(json).readObject());
}
public static BoxedJsObject boxedFrom(final @NotNull InputStream json) {
return boxedOf(Json.createReader(json).readObject());
}
public static BoxedJsObject boxedFrom(final @Nullable String json) {
return json == null ? BoxedJsObject.HAD_NULL_OBJECT : boxedFrom(new StringReader(json));
}
public static BoxedJsObject from(final @NotNull Reader json) {
return boxedOf(MutableJson.from(json));
}
public static BoxedJsObject from(final @NotNull InputStream json) {
return boxedOf(MutableJson.from(json));
}
public static BoxedJsObject from(final @Nullable String json) {
return boxedOf(json == null ? BoxedJsObject.HAD_NULL_OBJECT : MutableJson.from(json));
}
public static BoxedJsValue of(JsonValue jsonValue) {
return boxedOf(MutableJson.of(jsonValue));
}
public static BoxedJsArray of(JsonArray jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_ARRAY : boxedOf(jsonValue instanceof BoxedJsArray && ((BoxedJsArray) jsonValue).isValid() ? of((JsonArray) ((BoxedJsArray) jsonValue).jsonValue()) : jsonValue);
}
public static BoxedJsObject of(JsonObject jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_OBJECT : boxedOf(jsonValue instanceof BoxedJsObject && ((BoxedJsObject) jsonValue).isValid() ? of((JsonObject) ((BoxedJsObject) jsonValue).jsonValue()) : jsonValue);
}
public static BoxedJsNumber of(JsonNumber jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_NUMBER : boxedOf(jsonValue instanceof BoxedJsNumber && ((BoxedJsNumber) jsonValue).isValid() ? of((JsonNumber) ((BoxedJsNumber) jsonValue).jsonValue()) : jsonValue);
}
public static BoxedJsString of(JsonString jsonValue) {
return jsonValue == null ? BoxedJsObject.HAD_NULL_STRING : boxedOf(jsonValue instanceof BoxedJsString && ((BoxedJsString) jsonValue).isValid() ? of((JsonString) ((BoxedJsString) jsonValue).jsonValue()) : jsonValue);
}
public static BoxedJsValue of(final boolean jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsNumber of(final int jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsNumber of(final long jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsNumber of(final BigInteger jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsNumber of(final double jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsNumber of(final BigDecimal jsonValue) {
return boxedOf(jsonValue);
}
public static BoxedJsString of(final String jsonValue) {
return boxedOf(jsonValue);
}
/**
* Deep copy of the passed JsonValue to mutable boxed json
*
* @param jsonValue json value for which to create a deep copy, literal values are return simply wrapped
* @return boxed mutable deep copy of jsonValue
*/
public static JsonValue copyOf(JsonValue jsonValue) {
if (jsonValue instanceof BoxedJsValue) {
// get inner copy and make a copy of it
jsonValue = ((BoxedJsValue) jsonValue).jsonValue();
}
if (jsonValue == null) {
return JsonValue.NULL;
}
switch (jsonValue.getValueType()) {
case ARRAY: {
int iMax = ((JsonArray) jsonValue).size();
BoxedJsArray jsArray = new BoxedJsArrayImpl(new MutableJsArray(iMax));
for (int i = 0; i < iMax; i++) {
jsArray.add(copyOf(((JsonArray) jsonValue).get(i)));
}
return jsArray;
}
case OBJECT: {
int iMax = ((JsonObject) jsonValue).size();
BoxedJsObject jsObject = new BoxedJsObjectImpl(new MutableJsObject(iMax));
for (String key : ((JsonObject) jsonValue).keySet()) {
jsObject.put(key, copyOf(((JsonObject) jsonValue).get(key)));
}
return jsObject;
}
case STRING:
case NUMBER:
case TRUE:
case FALSE:
case NULL:
}
return jsonValue;
}
public static @Nullable Object[] parseEvalPath(final String partPath, final boolean allowEmptyIndex) {
String path = partPath.trim();
if (path.isEmpty()) return null;
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy