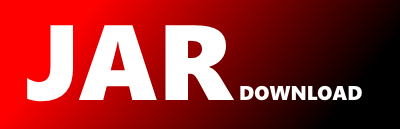
com.vladsch.flexmark.ast.util.LineCollectingVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flexmark Show documentation
Show all versions of flexmark Show documentation
Core of flexmark-java (implementation of CommonMark for parsing markdown and rendering to HTML)
package com.vladsch.flexmark.ast.util;
import com.vladsch.flexmark.ast.*;
import com.vladsch.flexmark.util.ast.Node;
import com.vladsch.flexmark.util.ast.NodeVisitor;
import com.vladsch.flexmark.util.ast.VisitHandler;
import com.vladsch.flexmark.util.sequence.Range;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
@SuppressWarnings("WeakerAccess")
public class LineCollectingVisitor {
final private NodeVisitor myVisitor;
private List myLines;
private List myEOLs;
private int myStartOffset;
private int myEndOffset;
public LineCollectingVisitor() {
myVisitor = new NodeVisitor(
new VisitHandler<>(Text.class, this::visit),
new VisitHandler<>(TextBase.class, this::visit),
new VisitHandler<>(HtmlEntity.class, this::visit),
new VisitHandler<>(HtmlInline.class, this::visit),
new VisitHandler<>(SoftLineBreak.class, this::visit),
new VisitHandler<>(HardLineBreak.class, this::visit)
);
myLines = Collections.EMPTY_LIST;
}
private void finalizeLines() {
if (myStartOffset < myEndOffset) {
Range range = Range.of(myStartOffset, myEndOffset);
myLines.add(range);
myEOLs.add(0);
myStartOffset = myEndOffset;
}
}
public List getLines() {
finalizeLines();
return myLines;
}
public List getEOLs() {
finalizeLines();
return myEOLs;
}
public void collect(Node node) {
myLines = new ArrayList<>();
myEOLs = new ArrayList<>();
myStartOffset = node.getStartOffset();
myEndOffset = node.getEndOffset();
myVisitor.visit(node);
}
public List collectAndGetRanges(Node node) {
collect(node);
return getLines();
}
private void visit(SoftLineBreak node) {
Range range = Range.of(myStartOffset, node.getEndOffset());
myLines.add(range);
myEOLs.add(node.getTextLength());
myStartOffset = node.getEndOffset();
}
private void visit(HardLineBreak node) {
Range range = Range.of(myStartOffset, node.getEndOffset());
myLines.add(range);
myEOLs.add(node.getTextLength());
myStartOffset = node.getEndOffset();
}
private void visit(HtmlEntity node) {
myEndOffset = node.getEndOffset();
}
private void visit(HtmlInline node) {
myEndOffset = node.getEndOffset();
}
private void visit(Text node) {
myEndOffset = node.getEndOffset();
}
private void visit(TextBase node) {
myEndOffset = node.getEndOffset();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy