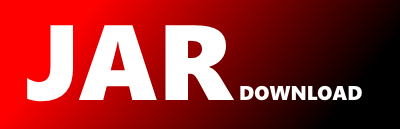
com.vmlens.trace.agent.bootstrap.callback.AnarsoftWeakHashMap Maven / Gradle / Ivy
package com.vmlens.trace.agent.bootstrap.callback;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.WeakReference;
public class AnarsoftWeakHashMap {
private static final int DEFAULT_INITIAL_CAPACITY = 16;
/**
* The maximum capacity, used if a higher value is implicitly specified
* by either of the constructors with arguments.
* MUST be a power of two <= 1<<30.
*/
private static final int MAXIMUM_CAPACITY = 1 << 30;
/**
* The load factor used when none specified in constructor.
*/
private static final float DEFAULT_LOAD_FACTOR = 0.75f;
/**
* The number of key-value mappings contained in this weak hash map.
*/
private int size;
/**
* The next size value at which to resize (capacity * load factor).
*/
private int threshold;
/**
* The load factor for the hash table.
*/
private final float loadFactor;
/**
* Reference queue for cleared WeakEntries
*/
private final ReferenceQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy