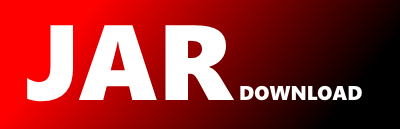
com.vmware.avi.sdk.model.ALBServicesUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
The newest version!
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The ALBServicesUser is a POJO class extends AviRestResource that used for creating
* ALBServicesUser.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class ALBServicesUser {
@JsonProperty("account_id")
private String accountId;
@JsonProperty("account_name")
private String accountName;
@JsonProperty("email")
private String email;
@JsonProperty("managed_accounts")
private List managedAccounts;
@JsonProperty("name")
private String name;
@JsonProperty("phone")
private String phone;
/**
* This is the getter method this will return the attribute value.
* Id of primary account of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return accountId
*/
public String getAccountId() {
return accountId;
}
/**
* This is the setter method to the attribute.
* Id of primary account of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param accountId set the accountId.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
* This is the getter method this will return the attribute value.
* Name of primary account of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return accountName
*/
public String getAccountName() {
return accountName;
}
/**
* This is the setter method to the attribute.
* Name of primary account of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param accountName set the accountName.
*/
public void setAccountName(String accountName) {
this.accountName = accountName;
}
/**
* This is the getter method this will return the attribute value.
* Email id of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return email
*/
public String getEmail() {
return email;
}
/**
* This is the setter method to the attribute.
* Email id of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param email set the email.
*/
public void setEmail(String email) {
this.email = email;
}
/**
* This is the getter method this will return the attribute value.
* Information about all the accounts managed by user in the customer portal.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return managedAccounts
*/
public List getManagedAccounts() {
return managedAccounts;
}
/**
* This is the setter method. this will set the managedAccounts
* Information about all the accounts managed by user in the customer portal.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return managedAccounts
*/
public void setManagedAccounts(List managedAccounts) {
this.managedAccounts = managedAccounts;
}
/**
* This is the setter method this will set the managedAccounts
* Information about all the accounts managed by user in the customer portal.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return managedAccounts
*/
public ALBServicesUser addManagedAccountsItem(ALBServicesAccount managedAccountsItem) {
if (this.managedAccounts == null) {
this.managedAccounts = new ArrayList();
}
this.managedAccounts.add(managedAccountsItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Name of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return name
*/
public String getName() {
return name;
}
/**
* This is the setter method to the attribute.
* Name of the portal user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param name set the name.
*/
public void setName(String name) {
this.name = name;
}
/**
* This is the getter method this will return the attribute value.
* Phone number of the user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return phone
*/
public String getPhone() {
return phone;
}
/**
* This is the setter method to the attribute.
* Phone number of the user.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param phone set the phone.
*/
public void setPhone(String phone) {
this.phone = phone;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ALBServicesUser objALBServicesUser = (ALBServicesUser) o;
return Objects.equals(this.name, objALBServicesUser.name)&&
Objects.equals(this.email, objALBServicesUser.email)&&
Objects.equals(this.accountName, objALBServicesUser.accountName)&&
Objects.equals(this.accountId, objALBServicesUser.accountId)&&
Objects.equals(this.managedAccounts, objALBServicesUser.managedAccounts)&&
Objects.equals(this.phone, objALBServicesUser.phone);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ALBServicesUser {\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountName: ").append(toIndentedString(accountName)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" managedAccounts: ").append(toIndentedString(managedAccounts)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy