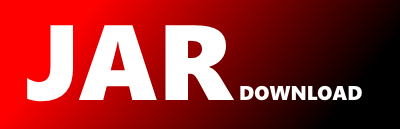
com.vmware.avi.sdk.model.BgpProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The BgpProfile is a POJO class extends AviRestResource that used for creating
* BgpProfile.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class BgpProfile {
@JsonProperty("community")
private List community;
@JsonProperty("hold_time")
private Integer holdTime = 180;
@JsonProperty("ibgp")
private Boolean ibgp = true;
@JsonProperty("ip_communities")
private List ipCommunities;
@JsonProperty("keepalive_interval")
private Integer keepaliveInterval = 60;
@JsonProperty("local_as")
private Integer localAs;
@JsonProperty("local_preference")
private Integer localPreference;
@JsonProperty("num_as_path_prepend")
private Integer numAsPathPrepend;
@JsonProperty("peers")
private List peers;
@JsonProperty("routing_options")
private List routingOptions;
@JsonProperty("send_community")
private Boolean sendCommunity = true;
@JsonProperty("shutdown")
private Boolean shutdown = false;
/**
* This is the getter method this will return the attribute value.
* Community string either in aa nn format where aa, nn is within [1,65535] or local-as|no-advertise|no-export|internet.
* Field introduced in 17.1.2.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return community
*/
public List getCommunity() {
return community;
}
/**
* This is the setter method. this will set the community
* Community string either in aa nn format where aa, nn is within [1,65535] or local-as|no-advertise|no-export|internet.
* Field introduced in 17.1.2.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return community
*/
public void setCommunity(List community) {
this.community = community;
}
/**
* This is the setter method this will set the community
* Community string either in aa nn format where aa, nn is within [1,65535] or local-as|no-advertise|no-export|internet.
* Field introduced in 17.1.2.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return community
*/
public BgpProfile addCommunityItem(String communityItem) {
if (this.community == null) {
this.community = new ArrayList();
}
this.community.add(communityItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Hold time for peers.
* Allowed values are 3-7200.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as 180.
* @return holdTime
*/
public Integer getHoldTime() {
return holdTime;
}
/**
* This is the setter method to the attribute.
* Hold time for peers.
* Allowed values are 3-7200.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as 180.
* @param holdTime set the holdTime.
*/
public void setHoldTime(Integer holdTime) {
this.holdTime = holdTime;
}
/**
* This is the getter method this will return the attribute value.
* Bgp peer type.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @return ibgp
*/
public Boolean getIbgp() {
return ibgp;
}
/**
* This is the setter method to the attribute.
* Bgp peer type.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @param ibgp set the ibgp.
*/
public void setIbgp(Boolean ibgp) {
this.ibgp = ibgp;
}
/**
* This is the getter method this will return the attribute value.
* Communities per ip address range.
* Field introduced in 17.1.3.
* Maximum of 1024 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ipCommunities
*/
public List getIpCommunities() {
return ipCommunities;
}
/**
* This is the setter method. this will set the ipCommunities
* Communities per ip address range.
* Field introduced in 17.1.3.
* Maximum of 1024 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ipCommunities
*/
public void setIpCommunities(List ipCommunities) {
this.ipCommunities = ipCommunities;
}
/**
* This is the setter method this will set the ipCommunities
* Communities per ip address range.
* Field introduced in 17.1.3.
* Maximum of 1024 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ipCommunities
*/
public BgpProfile addIpCommunitiesItem(IpCommunity ipCommunitiesItem) {
if (this.ipCommunities == null) {
this.ipCommunities = new ArrayList();
}
this.ipCommunities.add(ipCommunitiesItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Keepalive interval for peers.
* Allowed values are 0-3600.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as 60.
* @return keepaliveInterval
*/
public Integer getKeepaliveInterval() {
return keepaliveInterval;
}
/**
* This is the setter method to the attribute.
* Keepalive interval for peers.
* Allowed values are 0-3600.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as 60.
* @param keepaliveInterval set the keepaliveInterval.
*/
public void setKeepaliveInterval(Integer keepaliveInterval) {
this.keepaliveInterval = keepaliveInterval;
}
/**
* This is the getter method this will return the attribute value.
* Local autonomous system id.
* Allowed values are 1-4294967295.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return localAs
*/
public Integer getLocalAs() {
return localAs;
}
/**
* This is the setter method to the attribute.
* Local autonomous system id.
* Allowed values are 1-4294967295.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param localAs set the localAs.
*/
public void setLocalAs(Integer localAs) {
this.localAs = localAs;
}
/**
* This is the getter method this will return the attribute value.
* Local_pref to be used for routes advertised.
* Applicable only over ibgp.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return localPreference
*/
public Integer getLocalPreference() {
return localPreference;
}
/**
* This is the setter method to the attribute.
* Local_pref to be used for routes advertised.
* Applicable only over ibgp.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param localPreference set the localPreference.
*/
public void setLocalPreference(Integer localPreference) {
this.localPreference = localPreference;
}
/**
* This is the getter method this will return the attribute value.
* Number of times the local as should be prepended additionally.
* Allowed values are 1-10.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return numAsPathPrepend
*/
public Integer getNumAsPathPrepend() {
return numAsPathPrepend;
}
/**
* This is the setter method to the attribute.
* Number of times the local as should be prepended additionally.
* Allowed values are 1-10.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param numAsPathPrepend set the numAsPathPrepend.
*/
public void setNumAsPathPrepend(Integer numAsPathPrepend) {
this.numAsPathPrepend = numAsPathPrepend;
}
/**
* This is the getter method this will return the attribute value.
* Bgp peers.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return peers
*/
public List getPeers() {
return peers;
}
/**
* This is the setter method. this will set the peers
* Bgp peers.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return peers
*/
public void setPeers(List peers) {
this.peers = peers;
}
/**
* This is the setter method this will set the peers
* Bgp peers.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return peers
*/
public BgpProfile addPeersItem(BgpPeer peersItem) {
if (this.peers == null) {
this.peers = new ArrayList();
}
this.peers.add(peersItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Learning and advertising options for bgp peers.
* Field introduced in 20.1.1.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return routingOptions
*/
public List getRoutingOptions() {
return routingOptions;
}
/**
* This is the setter method. this will set the routingOptions
* Learning and advertising options for bgp peers.
* Field introduced in 20.1.1.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return routingOptions
*/
public void setRoutingOptions(List routingOptions) {
this.routingOptions = routingOptions;
}
/**
* This is the setter method this will set the routingOptions
* Learning and advertising options for bgp peers.
* Field introduced in 20.1.1.
* Maximum of 128 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return routingOptions
*/
public BgpProfile addRoutingOptionsItem(BgpRoutingOptions routingOptionsItem) {
if (this.routingOptions == null) {
this.routingOptions = new ArrayList();
}
this.routingOptions.add(routingOptionsItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Send community attribute to all peers.
* Field introduced in 17.1.2.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @return sendCommunity
*/
public Boolean getSendCommunity() {
return sendCommunity;
}
/**
* This is the setter method to the attribute.
* Send community attribute to all peers.
* Field introduced in 17.1.2.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @param sendCommunity set the sendCommunity.
*/
public void setSendCommunity(Boolean sendCommunity) {
this.sendCommunity = sendCommunity;
}
/**
* This is the getter method this will return the attribute value.
* Shutdown the bgp.
* Field introduced in 17.2.4.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as false.
* @return shutdown
*/
public Boolean getShutdown() {
return shutdown;
}
/**
* This is the setter method to the attribute.
* Shutdown the bgp.
* Field introduced in 17.2.4.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as false.
* @param shutdown set the shutdown.
*/
public void setShutdown(Boolean shutdown) {
this.shutdown = shutdown;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BgpProfile objBgpProfile = (BgpProfile) o;
return Objects.equals(this.localAs, objBgpProfile.localAs)&&
Objects.equals(this.ibgp, objBgpProfile.ibgp)&&
Objects.equals(this.peers, objBgpProfile.peers)&&
Objects.equals(this.keepaliveInterval, objBgpProfile.keepaliveInterval)&&
Objects.equals(this.holdTime, objBgpProfile.holdTime)&&
Objects.equals(this.sendCommunity, objBgpProfile.sendCommunity)&&
Objects.equals(this.community, objBgpProfile.community)&&
Objects.equals(this.ipCommunities, objBgpProfile.ipCommunities)&&
Objects.equals(this.localPreference, objBgpProfile.localPreference)&&
Objects.equals(this.numAsPathPrepend, objBgpProfile.numAsPathPrepend)&&
Objects.equals(this.routingOptions, objBgpProfile.routingOptions)&&
Objects.equals(this.shutdown, objBgpProfile.shutdown);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BgpProfile {\n");
sb.append(" community: ").append(toIndentedString(community)).append("\n");
sb.append(" holdTime: ").append(toIndentedString(holdTime)).append("\n");
sb.append(" ibgp: ").append(toIndentedString(ibgp)).append("\n");
sb.append(" ipCommunities: ").append(toIndentedString(ipCommunities)).append("\n");
sb.append(" keepaliveInterval: ").append(toIndentedString(keepaliveInterval)).append("\n");
sb.append(" localAs: ").append(toIndentedString(localAs)).append("\n");
sb.append(" localPreference: ").append(toIndentedString(localPreference)).append("\n");
sb.append(" numAsPathPrepend: ").append(toIndentedString(numAsPathPrepend)).append("\n");
sb.append(" peers: ").append(toIndentedString(peers)).append("\n");
sb.append(" routingOptions: ").append(toIndentedString(routingOptions)).append("\n");
sb.append(" sendCommunity: ").append(toIndentedString(sendCommunity)).append("\n");
sb.append(" shutdown: ").append(toIndentedString(shutdown)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy