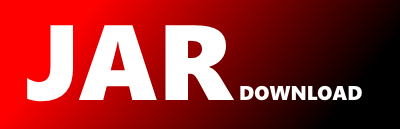
com.vmware.avi.sdk.model.CloudVipParkingIntf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
The newest version!
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The CloudVipParkingIntf is a POJO class extends AviRestResource that used for creating
* CloudVipParkingIntf.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class CloudVipParkingIntf {
@JsonProperty("cc_id")
private String ccId;
@JsonProperty("error_string")
private String errorString;
@JsonProperty("intf_id")
private String intfId;
@JsonProperty("subnet_id")
private String subnetId;
@JsonProperty("vtype")
private String vtype;
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ccId
*/
public String getCcId() {
return ccId;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param ccId set the ccId.
*/
public void setCcId(String ccId) {
this.ccId = ccId;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return errorString
*/
public String getErrorString() {
return errorString;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param errorString set the errorString.
*/
public void setErrorString(String errorString) {
this.errorString = errorString;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return intfId
*/
public String getIntfId() {
return intfId;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param intfId set the intfId.
*/
public void setIntfId(String intfId) {
this.intfId = intfId;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return subnetId
*/
public String getSubnetId() {
return subnetId;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param subnetId set the subnetId.
*/
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
/**
* This is the getter method this will return the attribute value.
* Enum options - CLOUD_NONE, CLOUD_VCENTER, CLOUD_OPENSTACK, CLOUD_AWS, CLOUD_VCA, CLOUD_APIC, CLOUD_MESOS, CLOUD_LINUXSERVER, CLOUD_DOCKER_UCP,
* CLOUD_RANCHER, CLOUD_OSHIFT_K8S, CLOUD_AZURE, CLOUD_GCP, CLOUD_NSXT.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return vtype
*/
public String getVtype() {
return vtype;
}
/**
* This is the setter method to the attribute.
* Enum options - CLOUD_NONE, CLOUD_VCENTER, CLOUD_OPENSTACK, CLOUD_AWS, CLOUD_VCA, CLOUD_APIC, CLOUD_MESOS, CLOUD_LINUXSERVER, CLOUD_DOCKER_UCP,
* CLOUD_RANCHER, CLOUD_OSHIFT_K8S, CLOUD_AZURE, CLOUD_GCP, CLOUD_NSXT.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param vtype set the vtype.
*/
public void setVtype(String vtype) {
this.vtype = vtype;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CloudVipParkingIntf objCloudVipParkingIntf = (CloudVipParkingIntf) o;
return Objects.equals(this.ccId, objCloudVipParkingIntf.ccId)&&
Objects.equals(this.vtype, objCloudVipParkingIntf.vtype)&&
Objects.equals(this.subnetId, objCloudVipParkingIntf.subnetId)&&
Objects.equals(this.intfId, objCloudVipParkingIntf.intfId)&&
Objects.equals(this.errorString, objCloudVipParkingIntf.errorString);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CloudVipParkingIntf {\n");
sb.append(" ccId: ").append(toIndentedString(ccId)).append("\n");
sb.append(" errorString: ").append(toIndentedString(errorString)).append("\n");
sb.append(" intfId: ").append(toIndentedString(intfId)).append("\n");
sb.append(" subnetId: ").append(toIndentedString(subnetId)).append("\n");
sb.append(" vtype: ").append(toIndentedString(vtype)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy