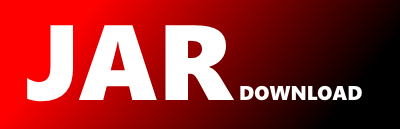
com.vmware.avi.sdk.model.GslbSiteHealthStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The GslbSiteHealthStatus is a POJO class extends AviRestResource that used for creating
* GslbSiteHealthStatus.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class GslbSiteHealthStatus {
@JsonProperty("controller_gsinfo")
private List controllerGsinfo;
@JsonProperty("datapath_gsinfo")
private List datapathGsinfo;
@JsonProperty("dns_info")
private GslbDnsInfo dnsInfo;
@JsonProperty("gap_table")
private List gapTable;
@JsonProperty("geo_table")
private List geoTable;
@JsonProperty("ghm_table")
private List ghmTable;
@JsonProperty("glb_table")
private List glbTable;
@JsonProperty("gs_table")
private List gsTable;
@JsonProperty("sw_version")
private String swVersion;
@JsonProperty("timestamp")
private Float timestamp;
/**
* This is the getter method this will return the attribute value.
* Controller retrieved gslb service operational info based of virtual service state.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return controllerGsinfo
*/
public List getControllerGsinfo() {
return controllerGsinfo;
}
/**
* This is the setter method. this will set the controllerGsinfo
* Controller retrieved gslb service operational info based of virtual service state.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return controllerGsinfo
*/
public void setControllerGsinfo(List controllerGsinfo) {
this.controllerGsinfo = controllerGsinfo;
}
/**
* This is the setter method this will set the controllerGsinfo
* Controller retrieved gslb service operational info based of virtual service state.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return controllerGsinfo
*/
public GslbSiteHealthStatus addControllerGsinfoItem(GslbPoolMemberRuntimeInfo controllerGsinfoItem) {
if (this.controllerGsinfo == null) {
this.controllerGsinfo = new ArrayList();
}
this.controllerGsinfo.add(controllerGsinfoItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Controller retrieved gslb service operational info based of dns datapath resolution.
* This information is generated only on those sites that have dns-vs participating in gslb.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return datapathGsinfo
*/
public List getDatapathGsinfo() {
return datapathGsinfo;
}
/**
* This is the setter method. this will set the datapathGsinfo
* Controller retrieved gslb service operational info based of dns datapath resolution.
* This information is generated only on those sites that have dns-vs participating in gslb.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return datapathGsinfo
*/
public void setDatapathGsinfo(List datapathGsinfo) {
this.datapathGsinfo = datapathGsinfo;
}
/**
* This is the setter method this will set the datapathGsinfo
* Controller retrieved gslb service operational info based of dns datapath resolution.
* This information is generated only on those sites that have dns-vs participating in gslb.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return datapathGsinfo
*/
public GslbSiteHealthStatus addDatapathGsinfoItem(GslbPoolMemberRuntimeInfo datapathGsinfoItem) {
if (this.datapathGsinfo == null) {
this.datapathGsinfo = new ArrayList();
}
this.datapathGsinfo.add(datapathGsinfoItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Dns info at the site.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return dnsInfo
*/
public GslbDnsInfo getDnsInfo() {
return dnsInfo;
}
/**
* This is the setter method to the attribute.
* Dns info at the site.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param dnsInfo set the dnsInfo.
*/
public void setDnsInfo(GslbDnsInfo dnsInfo) {
this.dnsInfo = dnsInfo;
}
/**
* This is the getter method this will return the attribute value.
* Gslb application persistence profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gapTable
*/
public List getGapTable() {
return gapTable;
}
/**
* This is the setter method. this will set the gapTable
* Gslb application persistence profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gapTable
*/
public void setGapTable(List gapTable) {
this.gapTable = gapTable;
}
/**
* This is the setter method this will set the gapTable
* Gslb application persistence profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gapTable
*/
public GslbSiteHealthStatus addGapTableItem(CfgState gapTableItem) {
if (this.gapTable == null) {
this.gapTable = new ArrayList();
}
this.gapTable.add(gapTableItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Gslb geo db profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return geoTable
*/
public List getGeoTable() {
return geoTable;
}
/**
* This is the setter method. this will set the geoTable
* Gslb geo db profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return geoTable
*/
public void setGeoTable(List geoTable) {
this.geoTable = geoTable;
}
/**
* This is the setter method this will set the geoTable
* Gslb geo db profile state at member.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return geoTable
*/
public GslbSiteHealthStatus addGeoTableItem(CfgState geoTableItem) {
if (this.geoTable == null) {
this.geoTable = new ArrayList();
}
this.geoTable.add(geoTableItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Gslb health monitor state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ghmTable
*/
public List getGhmTable() {
return ghmTable;
}
/**
* This is the setter method. this will set the ghmTable
* Gslb health monitor state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ghmTable
*/
public void setGhmTable(List ghmTable) {
this.ghmTable = ghmTable;
}
/**
* This is the setter method this will set the ghmTable
* Gslb health monitor state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ghmTable
*/
public GslbSiteHealthStatus addGhmTableItem(CfgState ghmTableItem) {
if (this.ghmTable == null) {
this.ghmTable = new ArrayList();
}
this.ghmTable.add(ghmTableItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Gslb state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return glbTable
*/
public List getGlbTable() {
return glbTable;
}
/**
* This is the setter method. this will set the glbTable
* Gslb state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return glbTable
*/
public void setGlbTable(List glbTable) {
this.glbTable = glbTable;
}
/**
* This is the setter method this will set the glbTable
* Gslb state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return glbTable
*/
public GslbSiteHealthStatus addGlbTableItem(CfgState glbTableItem) {
if (this.glbTable == null) {
this.glbTable = new ArrayList();
}
this.glbTable.add(glbTableItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Gslb service state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gsTable
*/
public List getGsTable() {
return gsTable;
}
/**
* This is the setter method. this will set the gsTable
* Gslb service state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gsTable
*/
public void setGsTable(List gsTable) {
this.gsTable = gsTable;
}
/**
* This is the setter method this will set the gsTable
* Gslb service state at member.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return gsTable
*/
public GslbSiteHealthStatus addGsTableItem(CfgState gsTableItem) {
if (this.gsTable == null) {
this.gsTable = new ArrayList();
}
this.gsTable.add(gsTableItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Current software version of the site.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return swVersion
*/
public String getSwVersion() {
return swVersion;
}
/**
* This is the setter method to the attribute.
* Current software version of the site.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param swVersion set the swVersion.
*/
public void setSwVersion(String swVersion) {
this.swVersion = swVersion;
}
/**
* This is the getter method this will return the attribute value.
* Timestamp of health-status generation.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return timestamp
*/
public Float getTimestamp() {
return timestamp;
}
/**
* This is the setter method to the attribute.
* Timestamp of health-status generation.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param timestamp set the timestamp.
*/
public void setTimestamp(Float timestamp) {
this.timestamp = timestamp;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GslbSiteHealthStatus objGslbSiteHealthStatus = (GslbSiteHealthStatus) o;
return Objects.equals(this.timestamp, objGslbSiteHealthStatus.timestamp)&&
Objects.equals(this.swVersion, objGslbSiteHealthStatus.swVersion)&&
Objects.equals(this.dnsInfo, objGslbSiteHealthStatus.dnsInfo)&&
Objects.equals(this.controllerGsinfo, objGslbSiteHealthStatus.controllerGsinfo)&&
Objects.equals(this.datapathGsinfo, objGslbSiteHealthStatus.datapathGsinfo)&&
Objects.equals(this.glbTable, objGslbSiteHealthStatus.glbTable)&&
Objects.equals(this.ghmTable, objGslbSiteHealthStatus.ghmTable)&&
Objects.equals(this.gsTable, objGslbSiteHealthStatus.gsTable)&&
Objects.equals(this.geoTable, objGslbSiteHealthStatus.geoTable)&&
Objects.equals(this.gapTable, objGslbSiteHealthStatus.gapTable);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GslbSiteHealthStatus {\n");
sb.append(" controllerGsinfo: ").append(toIndentedString(controllerGsinfo)).append("\n");
sb.append(" datapathGsinfo: ").append(toIndentedString(datapathGsinfo)).append("\n");
sb.append(" dnsInfo: ").append(toIndentedString(dnsInfo)).append("\n");
sb.append(" gapTable: ").append(toIndentedString(gapTable)).append("\n");
sb.append(" geoTable: ").append(toIndentedString(geoTable)).append("\n");
sb.append(" ghmTable: ").append(toIndentedString(ghmTable)).append("\n");
sb.append(" glbTable: ").append(toIndentedString(glbTable)).append("\n");
sb.append(" gsTable: ").append(toIndentedString(gsTable)).append("\n");
sb.append(" swVersion: ").append(toIndentedString(swVersion)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy