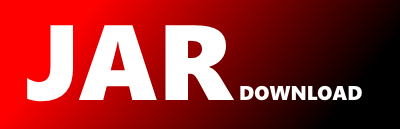
com.vmware.avi.sdk.model.GslbThirdPartySite Maven / Gradle / Ivy
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The GslbThirdPartySite is a POJO class extends AviRestResource that used for creating
* GslbThirdPartySite.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class GslbThirdPartySite {
@JsonProperty("cluster_uuid")
private String clusterUuid;
@JsonProperty("enabled")
private Boolean enabled = true;
@JsonProperty("hm_proxies")
private List hmProxies;
@JsonProperty("location")
private GslbGeoLocation location;
@JsonProperty("name")
private String name;
@JsonProperty("ratio")
private Integer ratio;
/**
* This is the getter method this will return the attribute value.
* Third-party-site identifier generated by avi.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials edition with any value, basic edition with any value, enterprise with cloud services
* edition.
* @return clusterUuid
*/
public String getClusterUuid() {
return clusterUuid;
}
/**
* This is the setter method to the attribute.
* Third-party-site identifier generated by avi.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials edition with any value, basic edition with any value, enterprise with cloud services
* edition.
* @param clusterUuid set the clusterUuid.
*/
public void setClusterUuid(String clusterUuid) {
this.clusterUuid = clusterUuid;
}
/**
* This is the getter method this will return the attribute value.
* Enable or disable the third-party site.
* This is useful in maintenance scenarios such as upgrade and routine maintenance.
* A disabled site's configuration shall be retained but it will not get any new configuration updates.
* Vips associated with the disabled site shall not be sent in dns response.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @return enabled
*/
public Boolean getEnabled() {
return enabled;
}
/**
* This is the setter method to the attribute.
* Enable or disable the third-party site.
* This is useful in maintenance scenarios such as upgrade and routine maintenance.
* A disabled site's configuration shall be retained but it will not get any new configuration updates.
* Vips associated with the disabled site shall not be sent in dns response.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @param enabled set the enabled.
*/
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
* This is the getter method this will return the attribute value.
* User can designate certain avi sites to run health monitor probes for vips/vs(es) for this site.
* This is useful in network deployments where the vips/vs(es) are reachable only from certain sites.
* A typical scenario is a firewall between two gslb sites.
* User may want to run health monitor probes from sites on either side of the firewall so that each designated site can derive a datapath view of
* the reachable members.
* If the health monitor proxies are not configured, then the default behavior is to run health monitor probes from all the active sites.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return hmProxies
*/
public List getHmProxies() {
return hmProxies;
}
/**
* This is the setter method. this will set the hmProxies
* User can designate certain avi sites to run health monitor probes for vips/vs(es) for this site.
* This is useful in network deployments where the vips/vs(es) are reachable only from certain sites.
* A typical scenario is a firewall between two gslb sites.
* User may want to run health monitor probes from sites on either side of the firewall so that each designated site can derive a datapath view of
* the reachable members.
* If the health monitor proxies are not configured, then the default behavior is to run health monitor probes from all the active sites.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return hmProxies
*/
public void setHmProxies(List hmProxies) {
this.hmProxies = hmProxies;
}
/**
* This is the setter method this will set the hmProxies
* User can designate certain avi sites to run health monitor probes for vips/vs(es) for this site.
* This is useful in network deployments where the vips/vs(es) are reachable only from certain sites.
* A typical scenario is a firewall between two gslb sites.
* User may want to run health monitor probes from sites on either side of the firewall so that each designated site can derive a datapath view of
* the reachable members.
* If the health monitor proxies are not configured, then the default behavior is to run health monitor probes from all the active sites.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return hmProxies
*/
public GslbThirdPartySite addHmProxiesItem(GslbHealthMonitorProxy hmProxiesItem) {
if (this.hmProxies == null) {
this.hmProxies = new ArrayList();
}
this.hmProxies.add(hmProxiesItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Geographic location of the site.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return location
*/
public GslbGeoLocation getLocation() {
return location;
}
/**
* This is the setter method to the attribute.
* Geographic location of the site.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param location set the location.
*/
public void setLocation(GslbGeoLocation location) {
this.location = location;
}
/**
* This is the getter method this will return the attribute value.
* Name of the third-party site.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return name
*/
public String getName() {
return name;
}
/**
* This is the setter method to the attribute.
* Name of the third-party site.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param name set the name.
*/
public void setName(String name) {
this.name = name;
}
/**
* This is the getter method this will return the attribute value.
* User can overide the individual gslbpoolmember ratio for all the vips of this site.
* If this field is not configured, then the gslbpoolmember ratio gets applied.
* Allowed values are 1-20.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ratio
*/
public Integer getRatio() {
return ratio;
}
/**
* This is the setter method to the attribute.
* User can overide the individual gslbpoolmember ratio for all the vips of this site.
* If this field is not configured, then the gslbpoolmember ratio gets applied.
* Allowed values are 1-20.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param ratio set the ratio.
*/
public void setRatio(Integer ratio) {
this.ratio = ratio;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GslbThirdPartySite objGslbThirdPartySite = (GslbThirdPartySite) o;
return Objects.equals(this.clusterUuid, objGslbThirdPartySite.clusterUuid)&&
Objects.equals(this.name, objGslbThirdPartySite.name)&&
Objects.equals(this.enabled, objGslbThirdPartySite.enabled)&&
Objects.equals(this.location, objGslbThirdPartySite.location)&&
Objects.equals(this.hmProxies, objGslbThirdPartySite.hmProxies)&&
Objects.equals(this.ratio, objGslbThirdPartySite.ratio);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GslbThirdPartySite {\n");
sb.append(" clusterUuid: ").append(toIndentedString(clusterUuid)).append("\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" hmProxies: ").append(toIndentedString(hmProxies)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ratio: ").append(toIndentedString(ratio)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy