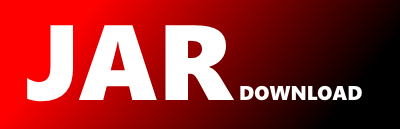
com.vmware.avi.sdk.model.MethodMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
The newest version!
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The MethodMatch is a POJO class extends AviRestResource that used for creating
* MethodMatch.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class MethodMatch {
@JsonProperty("match_criteria")
private String matchCriteria;
@JsonProperty("methods")
private List methods;
/**
* This is the getter method this will return the attribute value.
* Criterion to use for http method matching the method in the http request.
* Enum options - IS_IN, IS_NOT_IN.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return matchCriteria
*/
public String getMatchCriteria() {
return matchCriteria;
}
/**
* This is the setter method to the attribute.
* Criterion to use for http method matching the method in the http request.
* Enum options - IS_IN, IS_NOT_IN.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param matchCriteria set the matchCriteria.
*/
public void setMatchCriteria(String matchCriteria) {
this.matchCriteria = matchCriteria;
}
/**
* This is the getter method this will return the attribute value.
* Configure http method(s).
* Enum options - HTTP_METHOD_GET, HTTP_METHOD_HEAD, HTTP_METHOD_PUT, HTTP_METHOD_DELETE, HTTP_METHOD_POST, HTTP_METHOD_OPTIONS, HTTP_METHOD_TRACE,
* HTTP_METHOD_CONNECT, HTTP_METHOD_PATCH, HTTP_METHOD_PROPFIND, HTTP_METHOD_PROPPATCH, HTTP_METHOD_MKCOL, HTTP_METHOD_COPY, HTTP_METHOD_MOVE,
* HTTP_METHOD_LOCK, HTTP_METHOD_UNLOCK.
* Minimum of 1 items required.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), basic edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return methods
*/
public List getMethods() {
return methods;
}
/**
* This is the setter method. this will set the methods
* Configure http method(s).
* Enum options - HTTP_METHOD_GET, HTTP_METHOD_HEAD, HTTP_METHOD_PUT, HTTP_METHOD_DELETE, HTTP_METHOD_POST, HTTP_METHOD_OPTIONS, HTTP_METHOD_TRACE,
* HTTP_METHOD_CONNECT, HTTP_METHOD_PATCH, HTTP_METHOD_PROPFIND, HTTP_METHOD_PROPPATCH, HTTP_METHOD_MKCOL, HTTP_METHOD_COPY, HTTP_METHOD_MOVE,
* HTTP_METHOD_LOCK, HTTP_METHOD_UNLOCK.
* Minimum of 1 items required.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), basic edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return methods
*/
public void setMethods(List methods) {
this.methods = methods;
}
/**
* This is the setter method this will set the methods
* Configure http method(s).
* Enum options - HTTP_METHOD_GET, HTTP_METHOD_HEAD, HTTP_METHOD_PUT, HTTP_METHOD_DELETE, HTTP_METHOD_POST, HTTP_METHOD_OPTIONS, HTTP_METHOD_TRACE,
* HTTP_METHOD_CONNECT, HTTP_METHOD_PATCH, HTTP_METHOD_PROPFIND, HTTP_METHOD_PROPPATCH, HTTP_METHOD_MKCOL, HTTP_METHOD_COPY, HTTP_METHOD_MOVE,
* HTTP_METHOD_LOCK, HTTP_METHOD_UNLOCK.
* Minimum of 1 items required.
* Maximum of 16 items allowed.
* Allowed in enterprise edition with any value, essentials edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), basic edition(allowed values-
* http_method_get,http_method_put,http_method_post,http_method_head,http_method_options), enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return methods
*/
public MethodMatch addMethodsItem(String methodsItem) {
if (this.methods == null) {
this.methods = new ArrayList();
}
this.methods.add(methodsItem);
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MethodMatch objMethodMatch = (MethodMatch) o;
return Objects.equals(this.matchCriteria, objMethodMatch.matchCriteria)&&
Objects.equals(this.methods, objMethodMatch.methods);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MethodMatch {\n");
sb.append(" matchCriteria: ").append(toIndentedString(matchCriteria)).append("\n");
sb.append(" methods: ").append(toIndentedString(methods)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy