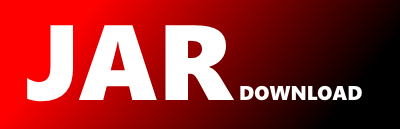
com.vmware.avi.sdk.model.StaticRoute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
The newest version!
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The StaticRoute is a POJO class extends AviRestResource that used for creating
* StaticRoute.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class StaticRoute {
@JsonProperty("disable_gateway_monitor")
private Boolean disableGatewayMonitor;
@JsonProperty("if_name")
private String ifName;
@JsonProperty("labels")
private List labels;
@JsonProperty("next_hop")
private IpAddr nextHop;
@JsonProperty("prefix")
private IpAddrPrefix prefix;
@JsonProperty("route_id")
private String routeId;
/**
* This is the getter method this will return the attribute value.
* Disable the gateway monitor for default gateway.
* They are monitored by default.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return disableGatewayMonitor
*/
public Boolean getDisableGatewayMonitor() {
return disableGatewayMonitor;
}
/**
* This is the setter method to the attribute.
* Disable the gateway monitor for default gateway.
* They are monitored by default.
* Field introduced in 17.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param disableGatewayMonitor set the disableGatewayMonitor.
*/
public void setDisableGatewayMonitor(Boolean disableGatewayMonitor) {
this.disableGatewayMonitor = disableGatewayMonitor;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ifName
*/
public String getIfName() {
return ifName;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param ifName set the ifName.
*/
public void setIfName(String ifName) {
this.ifName = ifName;
}
/**
* This is the getter method this will return the attribute value.
* Labels associated with this route.
* Field introduced in 20.1.1.
* Maximum of 1 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return labels
*/
public List getLabels() {
return labels;
}
/**
* This is the setter method. this will set the labels
* Labels associated with this route.
* Field introduced in 20.1.1.
* Maximum of 1 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return labels
*/
public void setLabels(List labels) {
this.labels = labels;
}
/**
* This is the setter method this will set the labels
* Labels associated with this route.
* Field introduced in 20.1.1.
* Maximum of 1 items allowed.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return labels
*/
public StaticRoute addLabelsItem(KeyValue labelsItem) {
if (this.labels == null) {
this.labels = new ArrayList();
}
this.labels.add(labelsItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return nextHop
*/
public IpAddr getNextHop() {
return nextHop;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param nextHop set the nextHop.
*/
public void setNextHop(IpAddr nextHop) {
this.nextHop = nextHop;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return prefix
*/
public IpAddrPrefix getPrefix() {
return prefix;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param prefix set the prefix.
*/
public void setPrefix(IpAddrPrefix prefix) {
this.prefix = prefix;
}
/**
* This is the getter method this will return the attribute value.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return routeId
*/
public String getRouteId() {
return routeId;
}
/**
* This is the setter method to the attribute.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param routeId set the routeId.
*/
public void setRouteId(String routeId) {
this.routeId = routeId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StaticRoute objStaticRoute = (StaticRoute) o;
return Objects.equals(this.prefix, objStaticRoute.prefix)&&
Objects.equals(this.nextHop, objStaticRoute.nextHop)&&
Objects.equals(this.ifName, objStaticRoute.ifName)&&
Objects.equals(this.routeId, objStaticRoute.routeId)&&
Objects.equals(this.disableGatewayMonitor, objStaticRoute.disableGatewayMonitor)&&
Objects.equals(this.labels, objStaticRoute.labels);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StaticRoute {\n");
sb.append(" disableGatewayMonitor: ").append(toIndentedString(disableGatewayMonitor)).append("\n");
sb.append(" ifName: ").append(toIndentedString(ifName)).append("\n");
sb.append(" labels: ").append(toIndentedString(labels)).append("\n");
sb.append(" nextHop: ").append(toIndentedString(nextHop)).append("\n");
sb.append(" prefix: ").append(toIndentedString(prefix)).append("\n");
sb.append(" routeId: ").append(toIndentedString(routeId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy