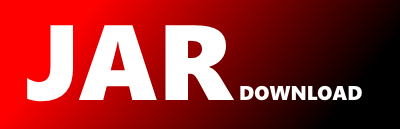
com.vmware.avi.sdk.model.WafRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avisdk Show documentation
Show all versions of avisdk Show documentation
Avi SDK is a java API which creates a session with controller and perform CRUD operations.
/*
* Copyright 2021 VMware, Inc.
* SPDX-License-Identifier: Apache License 2.0
*/
package com.vmware.avi.sdk.model;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* The WafRule is a POJO class extends AviRestResource that used for creating
* WafRule.
*
* @version 1.0
* @since
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class WafRule {
@JsonProperty("enable")
private Boolean enable = true;
@JsonProperty("exclude_list")
private List excludeList;
@JsonProperty("index")
private Integer index;
@JsonProperty("is_sensitive")
private Boolean isSensitive = false;
@JsonProperty("mode")
private String mode;
@JsonProperty("name")
private String name;
@JsonProperty("paranoia_level")
private String paranoiaLevel;
@JsonProperty("phase")
private String phase;
@JsonProperty("rule")
private String rule;
@JsonProperty("rule_id")
private String ruleId;
@JsonProperty("tags")
private List tags;
/**
* This is the getter method this will return the attribute value.
* Enable or disable waf rule group.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @return enable
*/
public Boolean getEnable() {
return enable;
}
/**
* This is the setter method to the attribute.
* Enable or disable waf rule group.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as true.
* @param enable set the enable.
*/
public void setEnable(Boolean enable) {
this.enable = enable;
}
/**
* This is the getter method this will return the attribute value.
* Exclude list for the waf rule.
* The fields in the exclude list entry are logically and'ed to deduce the exclusion criteria.
* If there are multiple excludelist entries, it will be 'logical or' of them.
* Field introduced in 17.2.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return excludeList
*/
public List getExcludeList() {
return excludeList;
}
/**
* This is the setter method. this will set the excludeList
* Exclude list for the waf rule.
* The fields in the exclude list entry are logically and'ed to deduce the exclusion criteria.
* If there are multiple excludelist entries, it will be 'logical or' of them.
* Field introduced in 17.2.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return excludeList
*/
public void setExcludeList(List excludeList) {
this.excludeList = excludeList;
}
/**
* This is the setter method this will set the excludeList
* Exclude list for the waf rule.
* The fields in the exclude list entry are logically and'ed to deduce the exclusion criteria.
* If there are multiple excludelist entries, it will be 'logical or' of them.
* Field introduced in 17.2.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return excludeList
*/
public WafRule addExcludeListItem(WafExcludeListEntry excludeListItem) {
if (this.excludeList == null) {
this.excludeList = new ArrayList();
}
this.excludeList.add(excludeListItem);
return this;
}
/**
* This is the getter method this will return the attribute value.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return index
*/
public Integer getIndex() {
return index;
}
/**
* This is the setter method to the attribute.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param index set the index.
*/
public void setIndex(Integer index) {
this.index = index;
}
/**
* This is the getter method this will return the attribute value.
* The rule field is sensitive and will not be displayed.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as false.
* @return isSensitive
*/
public Boolean getIsSensitive() {
return isSensitive;
}
/**
* This is the setter method to the attribute.
* The rule field is sensitive and will not be displayed.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as false.
* @param isSensitive set the isSensitive.
*/
public void setIsSensitive(Boolean isSensitive) {
this.isSensitive = isSensitive;
}
/**
* This is the getter method this will return the attribute value.
* Waf rule mode.
* This can be detection or enforcement.
* If this is not set, the policy mode is used.
* This only takes effect if the policy allows delegation.
* Enum options - WAF_MODE_DETECTION_ONLY, WAF_MODE_ENFORCEMENT.
* Field introduced in 18.1.5, 18.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return mode
*/
public String getMode() {
return mode;
}
/**
* This is the setter method to the attribute.
* Waf rule mode.
* This can be detection or enforcement.
* If this is not set, the policy mode is used.
* This only takes effect if the policy allows delegation.
* Enum options - WAF_MODE_DETECTION_ONLY, WAF_MODE_ENFORCEMENT.
* Field introduced in 18.1.5, 18.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param mode set the mode.
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
* This is the getter method this will return the attribute value.
* User-friendly optional name for a rule.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return name
*/
public String getName() {
return name;
}
/**
* This is the setter method to the attribute.
* User-friendly optional name for a rule.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param name set the name.
*/
public void setName(String name) {
this.name = name;
}
/**
* This is the getter method this will return the attribute value.
* Waf rule paranoia level.
* This field is informative, like rule_id and tags, it is generated by the system from the rule text.
* This field is filled for crs rules.
* Enum options - WAF_PARANOIA_LEVEL_LOW, WAF_PARANOIA_LEVEL_MEDIUM, WAF_PARANOIA_LEVEL_HIGH, WAF_PARANOIA_LEVEL_EXTREME.
* Field introduced in 22.1.3.
* Allowed in enterprise edition with any value, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return paranoiaLevel
*/
public String getParanoiaLevel() {
return paranoiaLevel;
}
/**
* This is the setter method to the attribute.
* Waf rule paranoia level.
* This field is informative, like rule_id and tags, it is generated by the system from the rule text.
* This field is filled for crs rules.
* Enum options - WAF_PARANOIA_LEVEL_LOW, WAF_PARANOIA_LEVEL_MEDIUM, WAF_PARANOIA_LEVEL_HIGH, WAF_PARANOIA_LEVEL_EXTREME.
* Field introduced in 22.1.3.
* Allowed in enterprise edition with any value, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param paranoiaLevel set the paranoiaLevel.
*/
public void setParanoiaLevel(String paranoiaLevel) {
this.paranoiaLevel = paranoiaLevel;
}
/**
* This is the getter method this will return the attribute value.
* The execution phase where this rule will be executed.
* Enum options - WAF_PHASE_CONNECTION, WAF_PHASE_REQUEST_HEADER, WAF_PHASE_REQUEST_BODY, WAF_PHASE_RESPONSE_HEADER, WAF_PHASE_RESPONSE_BODY,
* WAF_PHASE_LOGGING.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials edition with any value, basic edition with any value, enterprise with cloud services
* edition.
* @return phase
*/
public String getPhase() {
return phase;
}
/**
* This is the setter method to the attribute.
* The execution phase where this rule will be executed.
* Enum options - WAF_PHASE_CONNECTION, WAF_PHASE_REQUEST_HEADER, WAF_PHASE_REQUEST_BODY, WAF_PHASE_RESPONSE_HEADER, WAF_PHASE_RESPONSE_BODY,
* WAF_PHASE_LOGGING.
* Field introduced in 20.1.1.
* Allowed in enterprise edition with any value, essentials edition with any value, basic edition with any value, enterprise with cloud services
* edition.
* @param phase set the phase.
*/
public void setPhase(String phase) {
this.phase = phase;
}
/**
* This is the getter method this will return the attribute value.
* Rule as per modsec language.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return rule
*/
public String getRule() {
return rule;
}
/**
* This is the setter method to the attribute.
* Rule as per modsec language.
* Field introduced in 17.2.1.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param rule set the rule.
*/
public void setRule(String rule) {
this.rule = rule;
}
/**
* This is the getter method this will return the attribute value.
* Identifier (id) for a rule per modsec language.
* All secrule and secaction directives require an id.
* It is extracted from the id action in a modsec rule.
* Rules within a single waf policy are required to have unique rule_ids.
* Field introduced in 17.2.2.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return ruleId
*/
public String getRuleId() {
return ruleId;
}
/**
* This is the setter method to the attribute.
* Identifier (id) for a rule per modsec language.
* All secrule and secaction directives require an id.
* It is extracted from the id action in a modsec rule.
* Rules within a single waf policy are required to have unique rule_ids.
* Field introduced in 17.2.2.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @param ruleId set the ruleId.
*/
public void setRuleId(String ruleId) {
this.ruleId = ruleId;
}
/**
* This is the getter method this will return the attribute value.
* Tags for waf rule as per modsec language.
* They are extracted from the tag action in a modsec rule.
* Field introduced in 18.1.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return tags
*/
public List getTags() {
return tags;
}
/**
* This is the setter method. this will set the tags
* Tags for waf rule as per modsec language.
* They are extracted from the tag action in a modsec rule.
* Field introduced in 18.1.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return tags
*/
public void setTags(List tags) {
this.tags = tags;
}
/**
* This is the setter method this will set the tags
* Tags for waf rule as per modsec language.
* They are extracted from the tag action in a modsec rule.
* Field introduced in 18.1.3.
* Allowed in enterprise edition with any value, essentials, basic, enterprise with cloud services edition.
* Default value when not specified in API or module is interpreted by Avi Controller as null.
* @return tags
*/
public WafRule addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList();
}
this.tags.add(tagsItem);
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WafRule objWafRule = (WafRule) o;
return Objects.equals(this.index, objWafRule.index)&&
Objects.equals(this.name, objWafRule.name)&&
Objects.equals(this.ruleId, objWafRule.ruleId)&&
Objects.equals(this.enable, objWafRule.enable)&&
Objects.equals(this.rule, objWafRule.rule)&&
Objects.equals(this.excludeList, objWafRule.excludeList)&&
Objects.equals(this.tags, objWafRule.tags)&&
Objects.equals(this.mode, objWafRule.mode)&&
Objects.equals(this.isSensitive, objWafRule.isSensitive)&&
Objects.equals(this.phase, objWafRule.phase)&&
Objects.equals(this.paranoiaLevel, objWafRule.paranoiaLevel);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WafRule {\n");
sb.append(" enable: ").append(toIndentedString(enable)).append("\n");
sb.append(" excludeList: ").append(toIndentedString(excludeList)).append("\n");
sb.append(" index: ").append(toIndentedString(index)).append("\n");
sb.append(" isSensitive: ").append(toIndentedString(isSensitive)).append("\n");
sb.append(" mode: ").append(toIndentedString(mode)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" paranoiaLevel: ").append(toIndentedString(paranoiaLevel)).append("\n");
sb.append(" phase: ").append(toIndentedString(phase)).append("\n");
sb.append(" rule: ").append(toIndentedString(rule)).append("\n");
sb.append(" ruleId: ").append(toIndentedString(ruleId)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy