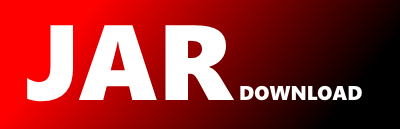
com.vmware.ovsdb.protocol.operation.notation.Row Maven / Gradle / Ivy
/*
* Copyright (c) 2018 VMware, Inc. All Rights Reserved.
*
* This product is licensed to you under the BSD-2 license (the "License").
* You may not use this product except in compliance with the BSD-2 License.
*
* This product may include a number of subcomponents with separate copyright
* notices and license terms. Your use of these subcomponents is subject to the
* terms and conditions of the subcomponent's license, as noted in the LICENSE
* file.
*
* SPDX-License-Identifier: BSD-2-Clause
*/
package com.vmware.ovsdb.protocol.operation.notation;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.vmware.ovsdb.protocol.operation.notation.deserializer.RowDeserializer;
import com.vmware.ovsdb.protocol.operation.notation.serializer.RowSerializer;
import java.util.HashMap;
import java.util.Objects;
import java.util.UUID;
import java.util.stream.Collectors;
/**
* Representation of {@literal }.
*
*
* {@literal
*
* A JSON object that describes a table row or a subset of a table
* row. Each member is the name of a table column paired with the
* of that column.
* }
*
*/
@JsonSerialize(using = RowSerializer.class)
@JsonDeserialize(using = RowDeserializer.class)
public class Row {
private java.util.Map columns;
public Row(java.util.Map columns) {
this.columns = columns;
}
public Row() {
this(new HashMap<>());
}
public java.util.Map getColumns() {
return columns;
}
private Row column(String name, Value value) {
columns.put(name, value);
return this;
}
public Row stringColumn(String name, String string) {
return column(name, Atom.string(string));
}
public Row integerColumn(String name, Long integer) {
return column(name, Atom.integer(integer));
}
public Row boolColumn(String name, Boolean bool) {
return column(name, Atom.bool(bool));
}
public Row uuidColumn(String name, Uuid uuid) {
return column(name, Atom.uuid(uuid));
}
public Row namedUuidColumn(String name, String uuidName) {
return column(name, Atom.namedUuid(uuidName));
}
public Row mapColumn(String name, java.util.Map map) {
return column(name, Map.of(map));
}
public Row setColumn(String name, java.util.Set set) {
return column(name, Set.of(set));
}
private T getAtomColumn(String name) {
Atom value = (Atom) columns.get(name);
return value == null ? null : value.getValue();
}
/**
* Get the value from a column whose type is {@literal }.
*
* @param name column name
* @return the value from the column
*/
public String getStringColumn(String name) {
return getAtomColumn(name);
}
/**
* Get the value from a column whose type is {@literal }.
*
* @param name column name
* @return the value from the column
*/
public Long getIntegerColumn(String name) {
return getAtomColumn(name);
}
/**
* Get the value from a column whose type is {@literal }.
*
* @param name column name
* @return the value from the column
*/
public Boolean getBooleanColumn(String name) {
return getAtomColumn(name);
}
/**
* Get the value from a column whose type is {@literal }.
*
* @param name column name
* @return the value from the column
*/
public Uuid getUuidColumn(String name) {
return getAtomColumn(name);
}
/**
* Get the value from a column whose type is {@literal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy