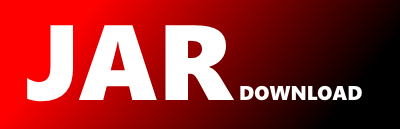
com.vmware.vim25.NetworkProfile Maven / Gradle / Ivy
package com.vmware.vim25;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for NetworkProfile complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="NetworkProfile">
* <complexContent>
* <extension base="{urn:vim25}ApplyProfile">
* <sequence>
* <element name="vswitch" type="{urn:vim25}VirtualSwitchProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="vmPortGroup" type="{urn:vim25}VmPortGroupProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="hostPortGroup" type="{urn:vim25}HostPortGroupProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="serviceConsolePortGroup" type="{urn:vim25}ServiceConsolePortGroupProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dnsConfig" type="{urn:vim25}NetworkProfileDnsConfigProfile" minOccurs="0"/>
* <element name="ipRouteConfig" type="{urn:vim25}IpRouteProfile" minOccurs="0"/>
* <element name="consoleIpRouteConfig" type="{urn:vim25}IpRouteProfile" minOccurs="0"/>
* <element name="pnic" type="{urn:vim25}PhysicalNicProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dvswitch" type="{urn:vim25}DvsProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dvsServiceConsoleNic" type="{urn:vim25}DvsServiceConsoleVNicProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dvsHostNic" type="{urn:vim25}DvsHostVNicProfile" maxOccurs="unbounded" minOccurs="0"/>
* <element name="netStackInstance" type="{urn:vim25}NetStackInstanceProfile" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "NetworkProfile", propOrder = {
"vswitch",
"vmPortGroup",
"hostPortGroup",
"serviceConsolePortGroup",
"dnsConfig",
"ipRouteConfig",
"consoleIpRouteConfig",
"pnic",
"dvswitch",
"dvsServiceConsoleNic",
"dvsHostNic",
"netStackInstance"
})
public class NetworkProfile
extends ApplyProfile
{
protected List vswitch;
protected List vmPortGroup;
protected List hostPortGroup;
protected List serviceConsolePortGroup;
protected NetworkProfileDnsConfigProfile dnsConfig;
protected IpRouteProfile ipRouteConfig;
protected IpRouteProfile consoleIpRouteConfig;
protected List pnic;
protected List dvswitch;
protected List dvsServiceConsoleNic;
protected List dvsHostNic;
protected List netStackInstance;
/**
* Gets the value of the vswitch property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the vswitch property.
*
*
* For example, to add a new item, do as follows:
*
* getVswitch().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VirtualSwitchProfile }
*
*
*/
public List getVswitch() {
if (vswitch == null) {
vswitch = new ArrayList();
}
return this.vswitch;
}
/**
* Gets the value of the vmPortGroup property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the vmPortGroup property.
*
*
* For example, to add a new item, do as follows:
*
* getVmPortGroup().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VmPortGroupProfile }
*
*
*/
public List getVmPortGroup() {
if (vmPortGroup == null) {
vmPortGroup = new ArrayList();
}
return this.vmPortGroup;
}
/**
* Gets the value of the hostPortGroup property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hostPortGroup property.
*
*
* For example, to add a new item, do as follows:
*
* getHostPortGroup().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HostPortGroupProfile }
*
*
*/
public List getHostPortGroup() {
if (hostPortGroup == null) {
hostPortGroup = new ArrayList();
}
return this.hostPortGroup;
}
/**
* Gets the value of the serviceConsolePortGroup property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the serviceConsolePortGroup property.
*
*
* For example, to add a new item, do as follows:
*
* getServiceConsolePortGroup().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ServiceConsolePortGroupProfile }
*
*
*/
public List getServiceConsolePortGroup() {
if (serviceConsolePortGroup == null) {
serviceConsolePortGroup = new ArrayList();
}
return this.serviceConsolePortGroup;
}
/**
* Gets the value of the dnsConfig property.
*
* @return
* possible object is
* {@link NetworkProfileDnsConfigProfile }
*
*/
public NetworkProfileDnsConfigProfile getDnsConfig() {
return dnsConfig;
}
/**
* Sets the value of the dnsConfig property.
*
* @param value
* allowed object is
* {@link NetworkProfileDnsConfigProfile }
*
*/
public void setDnsConfig(NetworkProfileDnsConfigProfile value) {
this.dnsConfig = value;
}
/**
* Gets the value of the ipRouteConfig property.
*
* @return
* possible object is
* {@link IpRouteProfile }
*
*/
public IpRouteProfile getIpRouteConfig() {
return ipRouteConfig;
}
/**
* Sets the value of the ipRouteConfig property.
*
* @param value
* allowed object is
* {@link IpRouteProfile }
*
*/
public void setIpRouteConfig(IpRouteProfile value) {
this.ipRouteConfig = value;
}
/**
* Gets the value of the consoleIpRouteConfig property.
*
* @return
* possible object is
* {@link IpRouteProfile }
*
*/
public IpRouteProfile getConsoleIpRouteConfig() {
return consoleIpRouteConfig;
}
/**
* Sets the value of the consoleIpRouteConfig property.
*
* @param value
* allowed object is
* {@link IpRouteProfile }
*
*/
public void setConsoleIpRouteConfig(IpRouteProfile value) {
this.consoleIpRouteConfig = value;
}
/**
* Gets the value of the pnic property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the pnic property.
*
*
* For example, to add a new item, do as follows:
*
* getPnic().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PhysicalNicProfile }
*
*
*/
public List getPnic() {
if (pnic == null) {
pnic = new ArrayList();
}
return this.pnic;
}
/**
* Gets the value of the dvswitch property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dvswitch property.
*
*
* For example, to add a new item, do as follows:
*
* getDvswitch().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DvsProfile }
*
*
*/
public List getDvswitch() {
if (dvswitch == null) {
dvswitch = new ArrayList();
}
return this.dvswitch;
}
/**
* Gets the value of the dvsServiceConsoleNic property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dvsServiceConsoleNic property.
*
*
* For example, to add a new item, do as follows:
*
* getDvsServiceConsoleNic().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DvsServiceConsoleVNicProfile }
*
*
*/
public List getDvsServiceConsoleNic() {
if (dvsServiceConsoleNic == null) {
dvsServiceConsoleNic = new ArrayList();
}
return this.dvsServiceConsoleNic;
}
/**
* Gets the value of the dvsHostNic property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dvsHostNic property.
*
*
* For example, to add a new item, do as follows:
*
* getDvsHostNic().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DvsHostVNicProfile }
*
*
*/
public List getDvsHostNic() {
if (dvsHostNic == null) {
dvsHostNic = new ArrayList();
}
return this.dvsHostNic;
}
/**
* Gets the value of the netStackInstance property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the netStackInstance property.
*
*
* For example, to add a new item, do as follows:
*
* getNetStackInstance().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NetStackInstanceProfile }
*
*
*/
public List getNetStackInstance() {
if (netStackInstance == null) {
netStackInstance = new ArrayList();
}
return this.netStackInstance;
}
}