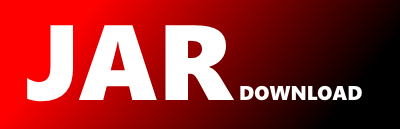
com.vmware.vim25.OvfParseDescriptorResult Maven / Gradle / Ivy
package com.vmware.vim25;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for OvfParseDescriptorResult complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="OvfParseDescriptorResult">
* <complexContent>
* <extension base="{urn:vim25}DynamicData">
* <sequence>
* <element name="eula" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="network" type="{urn:vim25}OvfNetworkInfo" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ipAllocationScheme" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ipProtocols" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="property" type="{urn:vim25}VAppPropertyInfo" maxOccurs="unbounded" minOccurs="0"/>
* <element name="productInfo" type="{urn:vim25}VAppProductInfo" minOccurs="0"/>
* <element name="annotation" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="approximateDownloadSize" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="approximateFlatDeploymentSize" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="approximateSparseDeploymentSize" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="defaultEntityName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="virtualApp" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="deploymentOption" type="{urn:vim25}OvfDeploymentOption" maxOccurs="unbounded" minOccurs="0"/>
* <element name="defaultDeploymentOption" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="entityName" type="{urn:vim25}KeyValue" maxOccurs="unbounded" minOccurs="0"/>
* <element name="annotatedOst" type="{urn:vim25}OvfConsumerOstNode" minOccurs="0"/>
* <element name="error" type="{urn:vim25}LocalizedMethodFault" maxOccurs="unbounded" minOccurs="0"/>
* <element name="warning" type="{urn:vim25}LocalizedMethodFault" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "OvfParseDescriptorResult", propOrder = {
"eula",
"network",
"ipAllocationScheme",
"ipProtocols",
"property",
"productInfo",
"annotation",
"approximateDownloadSize",
"approximateFlatDeploymentSize",
"approximateSparseDeploymentSize",
"defaultEntityName",
"virtualApp",
"deploymentOption",
"defaultDeploymentOption",
"entityName",
"annotatedOst",
"error",
"warning"
})
public class OvfParseDescriptorResult
extends DynamicData
{
protected List eula;
protected List network;
protected List ipAllocationScheme;
protected List ipProtocols;
protected List property;
protected VAppProductInfo productInfo;
@XmlElement(required = true)
protected String annotation;
protected Long approximateDownloadSize;
protected Long approximateFlatDeploymentSize;
protected Long approximateSparseDeploymentSize;
@XmlElement(required = true)
protected String defaultEntityName;
protected boolean virtualApp;
protected List deploymentOption;
@XmlElement(required = true)
protected String defaultDeploymentOption;
protected List entityName;
protected OvfConsumerOstNode annotatedOst;
protected List error;
protected List warning;
/**
* Gets the value of the eula property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the eula property.
*
*
* For example, to add a new item, do as follows:
*
* getEula().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getEula() {
if (eula == null) {
eula = new ArrayList();
}
return this.eula;
}
/**
* Gets the value of the network property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the network property.
*
*
* For example, to add a new item, do as follows:
*
* getNetwork().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OvfNetworkInfo }
*
*
*/
public List getNetwork() {
if (network == null) {
network = new ArrayList();
}
return this.network;
}
/**
* Gets the value of the ipAllocationScheme property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ipAllocationScheme property.
*
*
* For example, to add a new item, do as follows:
*
* getIpAllocationScheme().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getIpAllocationScheme() {
if (ipAllocationScheme == null) {
ipAllocationScheme = new ArrayList();
}
return this.ipAllocationScheme;
}
/**
* Gets the value of the ipProtocols property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ipProtocols property.
*
*
* For example, to add a new item, do as follows:
*
* getIpProtocols().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getIpProtocols() {
if (ipProtocols == null) {
ipProtocols = new ArrayList();
}
return this.ipProtocols;
}
/**
* Gets the value of the property property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the property property.
*
*
* For example, to add a new item, do as follows:
*
* getProperty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VAppPropertyInfo }
*
*
*/
public List getProperty() {
if (property == null) {
property = new ArrayList();
}
return this.property;
}
/**
* Gets the value of the productInfo property.
*
* @return
* possible object is
* {@link VAppProductInfo }
*
*/
public VAppProductInfo getProductInfo() {
return productInfo;
}
/**
* Sets the value of the productInfo property.
*
* @param value
* allowed object is
* {@link VAppProductInfo }
*
*/
public void setProductInfo(VAppProductInfo value) {
this.productInfo = value;
}
/**
* Gets the value of the annotation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAnnotation() {
return annotation;
}
/**
* Sets the value of the annotation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAnnotation(String value) {
this.annotation = value;
}
/**
* Gets the value of the approximateDownloadSize property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getApproximateDownloadSize() {
return approximateDownloadSize;
}
/**
* Sets the value of the approximateDownloadSize property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setApproximateDownloadSize(Long value) {
this.approximateDownloadSize = value;
}
/**
* Gets the value of the approximateFlatDeploymentSize property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getApproximateFlatDeploymentSize() {
return approximateFlatDeploymentSize;
}
/**
* Sets the value of the approximateFlatDeploymentSize property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setApproximateFlatDeploymentSize(Long value) {
this.approximateFlatDeploymentSize = value;
}
/**
* Gets the value of the approximateSparseDeploymentSize property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getApproximateSparseDeploymentSize() {
return approximateSparseDeploymentSize;
}
/**
* Sets the value of the approximateSparseDeploymentSize property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setApproximateSparseDeploymentSize(Long value) {
this.approximateSparseDeploymentSize = value;
}
/**
* Gets the value of the defaultEntityName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefaultEntityName() {
return defaultEntityName;
}
/**
* Sets the value of the defaultEntityName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefaultEntityName(String value) {
this.defaultEntityName = value;
}
/**
* Gets the value of the virtualApp property.
*
*/
public boolean isVirtualApp() {
return virtualApp;
}
/**
* Sets the value of the virtualApp property.
*
*/
public void setVirtualApp(boolean value) {
this.virtualApp = value;
}
/**
* Gets the value of the deploymentOption property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the deploymentOption property.
*
*
* For example, to add a new item, do as follows:
*
* getDeploymentOption().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OvfDeploymentOption }
*
*
*/
public List getDeploymentOption() {
if (deploymentOption == null) {
deploymentOption = new ArrayList();
}
return this.deploymentOption;
}
/**
* Gets the value of the defaultDeploymentOption property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefaultDeploymentOption() {
return defaultDeploymentOption;
}
/**
* Sets the value of the defaultDeploymentOption property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefaultDeploymentOption(String value) {
this.defaultDeploymentOption = value;
}
/**
* Gets the value of the entityName property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the entityName property.
*
*
* For example, to add a new item, do as follows:
*
* getEntityName().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link KeyValue }
*
*
*/
public List getEntityName() {
if (entityName == null) {
entityName = new ArrayList();
}
return this.entityName;
}
/**
* Gets the value of the annotatedOst property.
*
* @return
* possible object is
* {@link OvfConsumerOstNode }
*
*/
public OvfConsumerOstNode getAnnotatedOst() {
return annotatedOst;
}
/**
* Sets the value of the annotatedOst property.
*
* @param value
* allowed object is
* {@link OvfConsumerOstNode }
*
*/
public void setAnnotatedOst(OvfConsumerOstNode value) {
this.annotatedOst = value;
}
/**
* Gets the value of the error property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the error property.
*
*
* For example, to add a new item, do as follows:
*
* getError().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LocalizedMethodFault }
*
*
*/
public List getError() {
if (error == null) {
error = new ArrayList();
}
return this.error;
}
/**
* Gets the value of the warning property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the warning property.
*
*
* For example, to add a new item, do as follows:
*
* getWarning().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LocalizedMethodFault }
*
*
*/
public List getWarning() {
if (warning == null) {
warning = new ArrayList();
}
return this.warning;
}
}