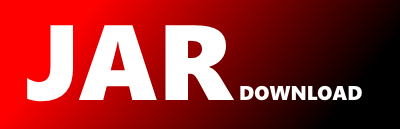
com.vmware.vim25.PlacementSpec Maven / Gradle / Ivy
package com.vmware.vim25;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for PlacementSpec complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PlacementSpec">
* <complexContent>
* <extension base="{urn:vim25}DynamicData">
* <sequence>
* <element name="priority" type="{urn:vim25}VirtualMachineMovePriority" minOccurs="0"/>
* <element name="vm" type="{urn:vim25}ManagedObjectReference" minOccurs="0"/>
* <element name="configSpec" type="{urn:vim25}VirtualMachineConfigSpec" minOccurs="0"/>
* <element name="relocateSpec" type="{urn:vim25}VirtualMachineRelocateSpec" minOccurs="0"/>
* <element name="hosts" type="{urn:vim25}ManagedObjectReference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="datastores" type="{urn:vim25}ManagedObjectReference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="storagePods" type="{urn:vim25}ManagedObjectReference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="disallowPrerequisiteMoves" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="rules" type="{urn:vim25}ClusterRuleInfo" maxOccurs="unbounded" minOccurs="0"/>
* <element name="key" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="placementType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cloneSpec" type="{urn:vim25}VirtualMachineCloneSpec" minOccurs="0"/>
* <element name="cloneName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PlacementSpec", propOrder = {
"priority",
"vm",
"configSpec",
"relocateSpec",
"hosts",
"datastores",
"storagePods",
"disallowPrerequisiteMoves",
"rules",
"key",
"placementType",
"cloneSpec",
"cloneName"
})
public class PlacementSpec
extends DynamicData
{
@XmlSchemaType(name = "string")
protected VirtualMachineMovePriority priority;
protected ManagedObjectReference vm;
protected VirtualMachineConfigSpec configSpec;
protected VirtualMachineRelocateSpec relocateSpec;
protected List hosts;
protected List datastores;
protected List storagePods;
protected Boolean disallowPrerequisiteMoves;
protected List rules;
protected String key;
protected String placementType;
protected VirtualMachineCloneSpec cloneSpec;
protected String cloneName;
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link VirtualMachineMovePriority }
*
*/
public VirtualMachineMovePriority getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link VirtualMachineMovePriority }
*
*/
public void setPriority(VirtualMachineMovePriority value) {
this.priority = value;
}
/**
* Gets the value of the vm property.
*
* @return
* possible object is
* {@link ManagedObjectReference }
*
*/
public ManagedObjectReference getVm() {
return vm;
}
/**
* Sets the value of the vm property.
*
* @param value
* allowed object is
* {@link ManagedObjectReference }
*
*/
public void setVm(ManagedObjectReference value) {
this.vm = value;
}
/**
* Gets the value of the configSpec property.
*
* @return
* possible object is
* {@link VirtualMachineConfigSpec }
*
*/
public VirtualMachineConfigSpec getConfigSpec() {
return configSpec;
}
/**
* Sets the value of the configSpec property.
*
* @param value
* allowed object is
* {@link VirtualMachineConfigSpec }
*
*/
public void setConfigSpec(VirtualMachineConfigSpec value) {
this.configSpec = value;
}
/**
* Gets the value of the relocateSpec property.
*
* @return
* possible object is
* {@link VirtualMachineRelocateSpec }
*
*/
public VirtualMachineRelocateSpec getRelocateSpec() {
return relocateSpec;
}
/**
* Sets the value of the relocateSpec property.
*
* @param value
* allowed object is
* {@link VirtualMachineRelocateSpec }
*
*/
public void setRelocateSpec(VirtualMachineRelocateSpec value) {
this.relocateSpec = value;
}
/**
* Gets the value of the hosts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hosts property.
*
*
* For example, to add a new item, do as follows:
*
* getHosts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ManagedObjectReference }
*
*
*/
public List getHosts() {
if (hosts == null) {
hosts = new ArrayList();
}
return this.hosts;
}
/**
* Gets the value of the datastores property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the datastores property.
*
*
* For example, to add a new item, do as follows:
*
* getDatastores().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ManagedObjectReference }
*
*
*/
public List getDatastores() {
if (datastores == null) {
datastores = new ArrayList();
}
return this.datastores;
}
/**
* Gets the value of the storagePods property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the storagePods property.
*
*
* For example, to add a new item, do as follows:
*
* getStoragePods().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ManagedObjectReference }
*
*
*/
public List getStoragePods() {
if (storagePods == null) {
storagePods = new ArrayList();
}
return this.storagePods;
}
/**
* Gets the value of the disallowPrerequisiteMoves property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isDisallowPrerequisiteMoves() {
return disallowPrerequisiteMoves;
}
/**
* Sets the value of the disallowPrerequisiteMoves property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setDisallowPrerequisiteMoves(Boolean value) {
this.disallowPrerequisiteMoves = value;
}
/**
* Gets the value of the rules property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rules property.
*
*
* For example, to add a new item, do as follows:
*
* getRules().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ClusterRuleInfo }
*
*
*/
public List getRules() {
if (rules == null) {
rules = new ArrayList();
}
return this.rules;
}
/**
* Gets the value of the key property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKey() {
return key;
}
/**
* Sets the value of the key property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKey(String value) {
this.key = value;
}
/**
* Gets the value of the placementType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlacementType() {
return placementType;
}
/**
* Sets the value of the placementType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPlacementType(String value) {
this.placementType = value;
}
/**
* Gets the value of the cloneSpec property.
*
* @return
* possible object is
* {@link VirtualMachineCloneSpec }
*
*/
public VirtualMachineCloneSpec getCloneSpec() {
return cloneSpec;
}
/**
* Sets the value of the cloneSpec property.
*
* @param value
* allowed object is
* {@link VirtualMachineCloneSpec }
*
*/
public void setCloneSpec(VirtualMachineCloneSpec value) {
this.cloneSpec = value;
}
/**
* Gets the value of the cloneName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCloneName() {
return cloneName;
}
/**
* Sets the value of the cloneName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCloneName(String value) {
this.cloneName = value;
}
}