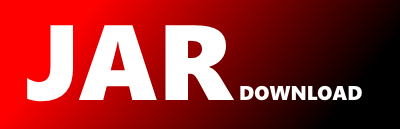
com.vmware.pscoe.iac.artifact.model.vrang.VraNgPackageDescriptor Maven / Gradle / Ivy
/*
* #%L
* artifact-manager
* %%
* Copyright (C) 2023 VMware
* %%
* Build Tools for VMware Aria
* Copyright 2023 VMware, Inc.
*
* This product is licensed to you under the BSD-2 license (the "License"). You may not use this product except in compliance with the BSD-2 License.
*
* This product may include a number of subcomponents with separate copyright notices and license terms. Your use of these subcomponents is subject to the terms and conditions of the subcomponent's license, as noted in the LICENSE file.
* #L%
*/
package com.vmware.pscoe.iac.artifact.model.vrang;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.vmware.pscoe.iac.artifact.model.PackageContent.Content;
import com.vmware.pscoe.iac.artifact.model.PackageDescriptor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class VraNgPackageDescriptor extends PackageDescriptor {
/**
* List of catalogItems.
*/
private List catalogItem;
/**
* List of blueprints.
*/
private List blueprint;
/**
* List of subscriptions.
*/
private List subscription;
/**
* List of catalogEntitlements.
*/
private List catalogEntitlement;
/**
* List of propertyGroups.
*/
private List propertyGroup;
/**
* List of customResources.
*/
private List customResource;
/**
* List of resourceActions.
*/
private List resourceAction;
/**
* List of contentSources.
*/
private List contentSource;
/**
* Container for lists of policies for each policy type.
*/
private VraNgPolicy policy;
/**
* Logger variable.
*/
private final Logger logger = LoggerFactory.getLogger(VraNgPackageDescriptor.class);
/**
* Getter.
*
* @return propertyGroup list.
*/
public List getPropertyGroup() {
return this.propertyGroup;
}
/**
* Setter.
*
* @param propertyGroup input values.
*/
public void setPropertyGroup(List propertyGroup) {
this.propertyGroup = propertyGroup;
}
/**
* Getter.
*
* @return blueprints.
*/
public List getBlueprint() {
return this.blueprint;
}
/**
* Setter.
*
* @param blueprint input values.
*/
public void setBlueprint(List blueprint) {
this.blueprint = blueprint;
}
/**
* Getter.
*
* @return catalogItems.
*/
public List getCatalogItem() {
return this.catalogItem;
}
/**
* Setter.
*
* @param catalogItem input values.
*/
public void setCatalogItem(List catalogItem) {
this.catalogItem = catalogItem;
}
/**
* Getter.
*
* @return subscriptions.
*/
public List getSubscription() {
return this.subscription;
}
/**
* Setter.
*
* @param subscription input values.
*/
public void setSubscription(List subscription) {
this.subscription = subscription;
}
/**
* Getter.
*
* @return catalog entitlements.
*/
public List getCatalogEntitlement() {
return catalogEntitlement;
}
/**
* Setter.
*
* @param catalogEntitlement input values.
*/
public void setCatalogEntitlement(List catalogEntitlement) {
this.catalogEntitlement = catalogEntitlement;
}
/**
* Getter.
*
* @return policy container.
*/
public VraNgPolicy getPolicy() {
return this.policy;
}
/**
* Setter.
*
* @param policy input value.
*/
public void setPolicy(VraNgPolicy policy) {
logger.info("VraNgPolicy.setPolicy {}", policy);
this.policy = policy;
}
/**
* Getter.
*
* @return customResources.
*/
public List getCustomResource() {
return this.customResource;
}
/**
* Setter.
*
* @param customResource input values.
*/
public void setCustomResource(List customResource) {
this.customResource = customResource;
}
/**
* Getter.
*
* @return resource actions.
*/
public List getResourceAction() {
return this.resourceAction;
}
/**
* Setter.
*
* @param resourceAction input values.
*/
public void setResourceAction(List resourceAction) {
this.resourceAction = resourceAction;
}
/**
* Getter.
*
* @return Content sources.
*/
public List getContentSource() {
return contentSource;
}
/**
* Setter.
*
* @param contentSource input values.
*/
public void setContentSource(List contentSource) {
this.contentSource = contentSource;
}
/**
* Getter.
*
* @param type VraNgPackageMemberType
* @return list of members of specific type.
*/
public List getMembersForType(VraNgPackageMemberType type) {
switch (type) {
case BLUEPRINT:
return getBlueprint();
case SUBSCRIPTION:
return getSubscription();
case CATALOG_ENTITLEMENT:
return getCatalogEntitlement();
case PROPERTY_GROUP:
return getPropertyGroup();
case CUSTOM_RESOURCE:
return getCustomResource();
case RESOURCE_ACTION:
return getResourceAction();
case CONTENT_SOURCE:
return getContentSource();
case CATALOG_ITEM:
return getCatalogItem();
default:
throw new RuntimeException("ContentType is not supported!");
}
}
/**
* List of values, filtered by type.isNativeContent().
*
* @return list
*/
public boolean hasNativeContent() {
return Arrays.stream(VraNgPackageMemberType.values())
.filter(type -> type.isNativeContent())
.anyMatch(type -> {
List memberNames = this.getMembersForType(type);
return memberNames != null && !memberNames.isEmpty();
});
}
/**
* Create a VraNgPackageDescriptor instance from file.
*
* @param filesystemPath the file
* @return VraNgPackageDescriptor instance
*/
public static VraNgPackageDescriptor getInstance(File filesystemPath) {
ObjectMapper mapper = new ObjectMapper(new YAMLFactory());
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.KEBAB_CASE);
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
try {
VraNgPackageDescriptor pkgDescriptor = mapper.readValue(filesystemPath, VraNgPackageDescriptor.class);
System.out.println(ReflectionToStringBuilder.toString(pkgDescriptor, ToStringStyle.MULTI_LINE_STYLE));
return pkgDescriptor;
} catch (Exception e) {
throw new RuntimeException(
"Unable to load vRA Package Descriptor[" + filesystemPath.getAbsolutePath() + "]", e);
}
}
/**
* Create a VraNgPackageDescriptor instance from VraNgPackageContent instance.
*
* @param content the package content instance
* @return VraNgPackageDescriptor instance
*/
public static VraNgPackageDescriptor getInstance(VraNgPackageContent content) {
Map> map = new HashMap<>();
for (VraNgPackageContent.ContentType type : VraNgPackageContent.ContentType.values()) {
map.put(type, new ArrayList<>());
}
for (Content c : content.getContent()) {
map.get(c.getType()).add(c.getName());
}
VraNgPackageDescriptor pd = new VraNgPackageDescriptor();
pd.blueprint = map.get(VraNgPackageContent.ContentType.BLUEPRINT);
pd.subscription = map.get(VraNgPackageContent.ContentType.SUBSCRIPTION);
pd.catalogEntitlement = map.get(VraNgPackageContent.ContentType.CATALOG_ENTITLEMENT);
pd.customResource = map.get(VraNgPackageContent.ContentType.CUSTOM_RESOURCE);
pd.resourceAction = map.get(VraNgPackageContent.ContentType.RESOURCE_ACTION);
pd.contentSource = map.get(VraNgPackageContent.ContentType.CONTENT_SOURCE);
pd.catalogItem = map.get(VraNgPackageContent.ContentType.CATALOG_ITEM);
pd.propertyGroup = map.get(VraNgPackageContent.ContentType.PROPERTY_GROUP);
// pd.policy = map.get(VraNgPackageContent.ContentType.POLICY);
return pd;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy