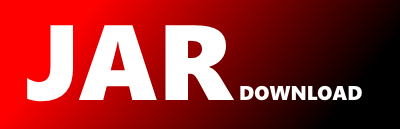
com.vmware.l10n.utils.SourceUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of singleton-manager-l10n Show documentation
Show all versions of singleton-manager-l10n Show documentation
A service that provides support for Software Internationalization and Localization
/*
* Copyright 2019-2023 VMware, Inc.
* SPDX-License-Identifier: EPL-2.0
*/
package com.vmware.l10n.utils;
import java.util.Iterator;
import java.util.Map;
import com.vmware.l10n.record.model.RecordModel;
import com.vmware.vip.common.constants.ConstantsChar;
import org.json.simple.parser.ContainerFactory;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanUtils;
import org.springframework.util.StringUtils;
import com.vmware.l10n.source.dto.SourceAPIResponseDTO;
import com.vmware.vip.common.constants.ConstantsKeys;
import com.vmware.vip.common.i18n.dto.SingleComponentDTO;
import com.vmware.vip.common.i18n.status.APIResponseStatus;
import com.vmware.vip.common.l10n.source.dto.ComponentMessagesDTO;
import com.vmware.vip.common.l10n.source.dto.StringSourceDTO;
public class SourceUtils {
private static Logger logger = LoggerFactory.getLogger(SourceUtils.class);
private SourceUtils() {}
/*
* create a StringSourceDTO object.
*/
public static StringSourceDTO createSourceDTO(String productName, String version, String component, String locale, String key, String source, String commentForSource, String sourceFormat){
StringSourceDTO stringSourceDTO = new StringSourceDTO();
stringSourceDTO.setProductName(productName);
stringSourceDTO.setComponent(component);
stringSourceDTO.setVersion(version);
stringSourceDTO.setLocale(locale);
stringSourceDTO.setKey(key);
stringSourceDTO.setSource(source);
stringSourceDTO.setComment(commentForSource);
stringSourceDTO.setSourceFormat(sourceFormat);
return stringSourceDTO;
}
public static SourceAPIResponseDTO handleSourceResponse(boolean isSourceCached){
SourceAPIResponseDTO sourceAPIResponseDTO = new SourceAPIResponseDTO();
if (isSourceCached) {
sourceAPIResponseDTO.setResponse(APIResponseStatus.TRANSLATION_COLLECT_REQUEST_SUCCESS);
} else {
sourceAPIResponseDTO.setResponse(APIResponseStatus.TRANSLATION_COLLECT_FAILURE);
}
return sourceAPIResponseDTO;
}
/*
* merge the cache content with JSON bundle by component, the structure of
* componentJSON is same with the bundle file.
*/
@SuppressWarnings("unchecked")
public static SingleComponentDTO mergeCacheWithBundle(
final SingleComponentDTO cachedComponentSourceDTO, final String componentJSON) {
ComponentMessagesDTO componentMessagesDTO = new ComponentMessagesDTO();
BeanUtils.copyProperties(cachedComponentSourceDTO, componentMessagesDTO);
if (!StringUtils.isEmpty(componentJSON)) {
JSONParser parser = new JSONParser();
ContainerFactory containerFactory = MapUtil.getContainerFactory();
Map messages;
Map bundle = null;
try {
bundle = (Map) parser.parse(componentJSON,
containerFactory);
} catch (ParseException e) {
logger.error(e.getMessage(), e);
}
if (bundle != null && !bundle.isEmpty()) {
messages = (Map) bundle.get(ConstantsKeys.MESSAGES);
Iterator> it = ((Map)cachedComponentSourceDTO
.getMessages()).entrySet().iterator();
boolean isChanged = false ;
while (it.hasNext()) {
Map.Entry entry = it.next();
String key = entry.getKey();
String value = entry.getValue() == null ? "" : (String)entry.getValue();
String v = messages.get(key) == null ? "" : (String)messages.get(key);
if(!value.equals(v) && !isChanged) {
isChanged = true;
}
MapUtil.updateKeyValue(messages, key, value);
}
if(isChanged) {
componentMessagesDTO.setId(System.currentTimeMillis());
} else {
componentMessagesDTO.setId(Long.parseLong(bundle.get(ConstantsKeys.ID) == null ? "0" : bundle.get(ConstantsKeys.ID).toString()));
}
componentMessagesDTO.setMessages(messages);
}
}
return componentMessagesDTO;
}
/**
* base on key parse the update source record
* @param key
* @param productParentDir
* @param lastModify
* @return
*/
public static RecordModel parseKeyStr2Record(String key, String productParentDir, long lastModify) {
String keyStr = key.replace(productParentDir, "");
String[] keyArry = keyStr.split(ConstantsChar.BACKSLASH);
RecordModel record = new RecordModel();
record.setProduct(keyArry[0]);
record.setVersion(keyArry[1]);
record.setComponent(keyArry[2]);
record.setLocale(ConstantsKeys.LATEST);
record.setStatus(lastModify);
return record;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy