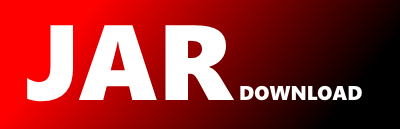
com.volcengine.model.tls.pb.PutLogRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of volc-sdk-java Show documentation
Show all versions of volc-sdk-java Show documentation
The VOLC Engine SDK for Java
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: PutLogRequest.proto
package com.volcengine.model.tls.pb;
public final class PutLogRequest {
private PutLogRequest() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface LogContentOrBuilder extends
// @@protoc_insertion_point(interface_extends:LogContent)
com.google.protobuf.MessageOrBuilder {
/**
* string Key = 1;
* @return The key.
*/
java.lang.String getKey();
/**
* string Key = 1;
* @return The bytes for key.
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* string Value = 2;
* @return The value.
*/
java.lang.String getValue();
/**
* string Value = 2;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code LogContent}
*/
public static final class LogContent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:LogContent)
LogContentOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogContent.newBuilder() to construct.
private LogContent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogContent() {
key_ = "";
value_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogContent();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogContent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
value_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogContent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogContent.class, PutLogRequest.LogContent.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* string Key = 1;
* @return The key.
*/
@java.lang.Override
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
* string Key = 1;
* @return The bytes for key.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private volatile java.lang.Object value_;
/**
* string Value = 2;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* string Value = 2;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, key_);
}
if (!getValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, key_);
}
if (!getValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof PutLogRequest.LogContent)) {
return super.equals(obj);
}
PutLogRequest.LogContent other = (PutLogRequest.LogContent) obj;
if (!getKey()
.equals(other.getKey())) return false;
if (!getValue()
.equals(other.getValue())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static PutLogRequest.LogContent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogContent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogContent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogContent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogContent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogContent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogContent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogContent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogContent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static PutLogRequest.LogContent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogContent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogContent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(PutLogRequest.LogContent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code LogContent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:LogContent)
PutLogRequest.LogContentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogContent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogContent.class, PutLogRequest.LogContent.Builder.class);
}
// Construct using PutLogRequest.LogContent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
key_ = "";
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return PutLogRequest.internal_static_pb_LogContent_descriptor;
}
@java.lang.Override
public PutLogRequest.LogContent getDefaultInstanceForType() {
return PutLogRequest.LogContent.getDefaultInstance();
}
@java.lang.Override
public PutLogRequest.LogContent build() {
PutLogRequest.LogContent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public PutLogRequest.LogContent buildPartial() {
PutLogRequest.LogContent result = new PutLogRequest.LogContent(this);
result.key_ = key_;
result.value_ = value_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof PutLogRequest.LogContent) {
return mergeFrom((PutLogRequest.LogContent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(PutLogRequest.LogContent other) {
if (other == PutLogRequest.LogContent.getDefaultInstance()) return this;
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (!other.getValue().isEmpty()) {
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
PutLogRequest.LogContent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (PutLogRequest.LogContent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object key_ = "";
/**
* string Key = 1;
* @return The key.
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string Key = 1;
* @return The bytes for key.
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string Key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* string Key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* string Key = 1;
* @param value The bytes for key to set.
* @return This builder for chaining.
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* string Value = 2;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string Value = 2;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string Value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* string Value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* string Value = 2;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
value_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:LogContent)
}
// @@protoc_insertion_point(class_scope:LogContent)
private static final PutLogRequest.LogContent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PutLogRequest.LogContent();
}
public static PutLogRequest.LogContent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogContent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogContent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public PutLogRequest.LogContent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogOrBuilder extends
// @@protoc_insertion_point(interface_extends:Log)
com.google.protobuf.MessageOrBuilder {
/**
*
* UNIX Time Format
*
*
* int64 Time = 1;
* @return The time.
*/
long getTime();
/**
* repeated .LogContent Contents = 2;
*/
java.util.List
getContentsList();
/**
* repeated .LogContent Contents = 2;
*/
PutLogRequest.LogContent getContents(int index);
/**
* repeated .LogContent Contents = 2;
*/
int getContentsCount();
/**
* repeated .LogContent Contents = 2;
*/
java.util.List extends PutLogRequest.LogContentOrBuilder>
getContentsOrBuilderList();
/**
* repeated .LogContent Contents = 2;
*/
PutLogRequest.LogContentOrBuilder getContentsOrBuilder(
int index);
}
/**
* Protobuf type {@code Log}
*/
public static final class Log extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:Log)
LogOrBuilder {
private static final long serialVersionUID = 0L;
// Use Log.newBuilder() to construct.
private Log(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Log() {
contents_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Log();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Log(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
time_ = input.readInt64();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
contents_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
contents_.add(
input.readMessage(PutLogRequest.LogContent.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
contents_ = java.util.Collections.unmodifiableList(contents_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_Log_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_Log_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.Log.class, PutLogRequest.Log.Builder.class);
}
public static final int TIME_FIELD_NUMBER = 1;
private long time_;
/**
*
* UNIX Time Format
*
*
* int64 Time = 1;
* @return The time.
*/
@java.lang.Override
public long getTime() {
return time_;
}
public static final int CONTENTS_FIELD_NUMBER = 2;
private java.util.List contents_;
/**
* repeated .LogContent Contents = 2;
*/
@java.lang.Override
public java.util.List getContentsList() {
return contents_;
}
/**
* repeated .LogContent Contents = 2;
*/
@java.lang.Override
public java.util.List extends PutLogRequest.LogContentOrBuilder>
getContentsOrBuilderList() {
return contents_;
}
/**
* repeated .LogContent Contents = 2;
*/
@java.lang.Override
public int getContentsCount() {
return contents_.size();
}
/**
* repeated .LogContent Contents = 2;
*/
@java.lang.Override
public PutLogRequest.LogContent getContents(int index) {
return contents_.get(index);
}
/**
* repeated .LogContent Contents = 2;
*/
@java.lang.Override
public PutLogRequest.LogContentOrBuilder getContentsOrBuilder(
int index) {
return contents_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (time_ != 0L) {
output.writeInt64(1, time_);
}
for (int i = 0; i < contents_.size(); i++) {
output.writeMessage(2, contents_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (time_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, time_);
}
for (int i = 0; i < contents_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, contents_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof PutLogRequest.Log)) {
return super.equals(obj);
}
PutLogRequest.Log other = (PutLogRequest.Log) obj;
if (getTime()
!= other.getTime()) return false;
if (!getContentsList()
.equals(other.getContentsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTime());
if (getContentsCount() > 0) {
hash = (37 * hash) + CONTENTS_FIELD_NUMBER;
hash = (53 * hash) + getContentsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static PutLogRequest.Log parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.Log parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.Log parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.Log parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.Log parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.Log parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.Log parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.Log parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.Log parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static PutLogRequest.Log parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.Log parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.Log parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(PutLogRequest.Log prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Log}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:Log)
PutLogRequest.LogOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_Log_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_Log_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.Log.class, PutLogRequest.Log.Builder.class);
}
// Construct using PutLogRequest.Log.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getContentsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
time_ = 0L;
if (contentsBuilder_ == null) {
contents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
contentsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return PutLogRequest.internal_static_pb_Log_descriptor;
}
@java.lang.Override
public PutLogRequest.Log getDefaultInstanceForType() {
return PutLogRequest.Log.getDefaultInstance();
}
@java.lang.Override
public PutLogRequest.Log build() {
PutLogRequest.Log result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public PutLogRequest.Log buildPartial() {
PutLogRequest.Log result = new PutLogRequest.Log(this);
int from_bitField0_ = bitField0_;
result.time_ = time_;
if (contentsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
contents_ = java.util.Collections.unmodifiableList(contents_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.contents_ = contents_;
} else {
result.contents_ = contentsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof PutLogRequest.Log) {
return mergeFrom((PutLogRequest.Log)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(PutLogRequest.Log other) {
if (other == PutLogRequest.Log.getDefaultInstance()) return this;
if (other.getTime() != 0L) {
setTime(other.getTime());
}
if (contentsBuilder_ == null) {
if (!other.contents_.isEmpty()) {
if (contents_.isEmpty()) {
contents_ = other.contents_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureContentsIsMutable();
contents_.addAll(other.contents_);
}
onChanged();
}
} else {
if (!other.contents_.isEmpty()) {
if (contentsBuilder_.isEmpty()) {
contentsBuilder_.dispose();
contentsBuilder_ = null;
contents_ = other.contents_;
bitField0_ = (bitField0_ & ~0x00000001);
contentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getContentsFieldBuilder() : null;
} else {
contentsBuilder_.addAllMessages(other.contents_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
PutLogRequest.Log parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (PutLogRequest.Log) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long time_ ;
/**
*
* UNIX Time Format
*
*
* int64 Time = 1;
* @return The time.
*/
@java.lang.Override
public long getTime() {
return time_;
}
/**
*
* UNIX Time Format
*
*
* int64 Time = 1;
* @param value The time to set.
* @return This builder for chaining.
*/
public Builder setTime(long value) {
time_ = value;
onChanged();
return this;
}
/**
*
* UNIX Time Format
*
*
* int64 Time = 1;
* @return This builder for chaining.
*/
public Builder clearTime() {
time_ = 0L;
onChanged();
return this;
}
private java.util.List contents_ =
java.util.Collections.emptyList();
private void ensureContentsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
contents_ = new java.util.ArrayList(contents_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogContent, PutLogRequest.LogContent.Builder, PutLogRequest.LogContentOrBuilder> contentsBuilder_;
/**
* repeated .LogContent Contents = 2;
*/
public java.util.List getContentsList() {
if (contentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(contents_);
} else {
return contentsBuilder_.getMessageList();
}
}
/**
* repeated .LogContent Contents = 2;
*/
public int getContentsCount() {
if (contentsBuilder_ == null) {
return contents_.size();
} else {
return contentsBuilder_.getCount();
}
}
/**
* repeated .LogContent Contents = 2;
*/
public PutLogRequest.LogContent getContents(int index) {
if (contentsBuilder_ == null) {
return contents_.get(index);
} else {
return contentsBuilder_.getMessage(index);
}
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder setContents(
int index, PutLogRequest.LogContent value) {
if (contentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentsIsMutable();
contents_.set(index, value);
onChanged();
} else {
contentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder setContents(
int index, PutLogRequest.LogContent.Builder builderForValue) {
if (contentsBuilder_ == null) {
ensureContentsIsMutable();
contents_.set(index, builderForValue.build());
onChanged();
} else {
contentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder addContents(PutLogRequest.LogContent value) {
if (contentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentsIsMutable();
contents_.add(value);
onChanged();
} else {
contentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder addContents(
int index, PutLogRequest.LogContent value) {
if (contentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentsIsMutable();
contents_.add(index, value);
onChanged();
} else {
contentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder addContents(
PutLogRequest.LogContent.Builder builderForValue) {
if (contentsBuilder_ == null) {
ensureContentsIsMutable();
contents_.add(builderForValue.build());
onChanged();
} else {
contentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder addContents(
int index, PutLogRequest.LogContent.Builder builderForValue) {
if (contentsBuilder_ == null) {
ensureContentsIsMutable();
contents_.add(index, builderForValue.build());
onChanged();
} else {
contentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder addAllContents(
java.lang.Iterable extends PutLogRequest.LogContent> values) {
if (contentsBuilder_ == null) {
ensureContentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, contents_);
onChanged();
} else {
contentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder clearContents() {
if (contentsBuilder_ == null) {
contents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
contentsBuilder_.clear();
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public Builder removeContents(int index) {
if (contentsBuilder_ == null) {
ensureContentsIsMutable();
contents_.remove(index);
onChanged();
} else {
contentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .LogContent Contents = 2;
*/
public PutLogRequest.LogContent.Builder getContentsBuilder(
int index) {
return getContentsFieldBuilder().getBuilder(index);
}
/**
* repeated .LogContent Contents = 2;
*/
public PutLogRequest.LogContentOrBuilder getContentsOrBuilder(
int index) {
if (contentsBuilder_ == null) {
return contents_.get(index); } else {
return contentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .LogContent Contents = 2;
*/
public java.util.List extends PutLogRequest.LogContentOrBuilder>
getContentsOrBuilderList() {
if (contentsBuilder_ != null) {
return contentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(contents_);
}
}
/**
* repeated .LogContent Contents = 2;
*/
public PutLogRequest.LogContent.Builder addContentsBuilder() {
return getContentsFieldBuilder().addBuilder(
PutLogRequest.LogContent.getDefaultInstance());
}
/**
* repeated .LogContent Contents = 2;
*/
public PutLogRequest.LogContent.Builder addContentsBuilder(
int index) {
return getContentsFieldBuilder().addBuilder(
index, PutLogRequest.LogContent.getDefaultInstance());
}
/**
* repeated .LogContent Contents = 2;
*/
public java.util.List
getContentsBuilderList() {
return getContentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogContent, PutLogRequest.LogContent.Builder, PutLogRequest.LogContentOrBuilder>
getContentsFieldBuilder() {
if (contentsBuilder_ == null) {
contentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogContent, PutLogRequest.LogContent.Builder, PutLogRequest.LogContentOrBuilder>(
contents_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
contents_ = null;
}
return contentsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:Log)
}
// @@protoc_insertion_point(class_scope:Log)
private static final PutLogRequest.Log DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PutLogRequest.Log();
}
public static PutLogRequest.Log getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Log parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Log(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public PutLogRequest.Log getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogTagOrBuilder extends
// @@protoc_insertion_point(interface_extends:LogTag)
com.google.protobuf.MessageOrBuilder {
/**
* string Key = 1;
* @return The key.
*/
java.lang.String getKey();
/**
* string Key = 1;
* @return The bytes for key.
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* string Value = 2;
* @return The value.
*/
java.lang.String getValue();
/**
* string Value = 2;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code LogTag}
*/
public static final class LogTag extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:LogTag)
LogTagOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogTag.newBuilder() to construct.
private LogTag(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogTag() {
key_ = "";
value_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogTag();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogTag(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
value_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogTag_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogTag_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogTag.class, PutLogRequest.LogTag.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* string Key = 1;
* @return The key.
*/
@java.lang.Override
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
* string Key = 1;
* @return The bytes for key.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private volatile java.lang.Object value_;
/**
* string Value = 2;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* string Value = 2;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, key_);
}
if (!getValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, key_);
}
if (!getValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof PutLogRequest.LogTag)) {
return super.equals(obj);
}
PutLogRequest.LogTag other = (PutLogRequest.LogTag) obj;
if (!getKey()
.equals(other.getKey())) return false;
if (!getValue()
.equals(other.getValue())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static PutLogRequest.LogTag parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogTag parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogTag parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogTag parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogTag parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogTag parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogTag parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogTag parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogTag parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static PutLogRequest.LogTag parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogTag parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogTag parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(PutLogRequest.LogTag prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code LogTag}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:LogTag)
PutLogRequest.LogTagOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogTag_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogTag_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogTag.class, PutLogRequest.LogTag.Builder.class);
}
// Construct using PutLogRequest.LogTag.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
key_ = "";
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return PutLogRequest.internal_static_pb_LogTag_descriptor;
}
@java.lang.Override
public PutLogRequest.LogTag getDefaultInstanceForType() {
return PutLogRequest.LogTag.getDefaultInstance();
}
@java.lang.Override
public PutLogRequest.LogTag build() {
PutLogRequest.LogTag result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public PutLogRequest.LogTag buildPartial() {
PutLogRequest.LogTag result = new PutLogRequest.LogTag(this);
result.key_ = key_;
result.value_ = value_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof PutLogRequest.LogTag) {
return mergeFrom((PutLogRequest.LogTag)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(PutLogRequest.LogTag other) {
if (other == PutLogRequest.LogTag.getDefaultInstance()) return this;
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (!other.getValue().isEmpty()) {
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
PutLogRequest.LogTag parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (PutLogRequest.LogTag) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object key_ = "";
/**
* string Key = 1;
* @return The key.
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string Key = 1;
* @return The bytes for key.
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string Key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* string Key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* string Key = 1;
* @param value The bytes for key to set.
* @return This builder for chaining.
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* string Value = 2;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string Value = 2;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string Value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* string Value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* string Value = 2;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
value_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:LogTag)
}
// @@protoc_insertion_point(class_scope:LogTag)
private static final PutLogRequest.LogTag DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PutLogRequest.LogTag();
}
public static PutLogRequest.LogTag getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogTag parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogTag(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public PutLogRequest.LogTag getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogGroupOrBuilder extends
// @@protoc_insertion_point(interface_extends:LogGroup)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .Log Logs = 1;
*/
java.util.List
getLogsList();
/**
* repeated .Log Logs = 1;
*/
PutLogRequest.Log getLogs(int index);
/**
* repeated .Log Logs = 1;
*/
int getLogsCount();
/**
* repeated .Log Logs = 1;
*/
java.util.List extends PutLogRequest.LogOrBuilder>
getLogsOrBuilderList();
/**
* repeated .Log Logs = 1;
*/
PutLogRequest.LogOrBuilder getLogsOrBuilder(
int index);
/**
* string Source = 2;
* @return The source.
*/
java.lang.String getSource();
/**
* string Source = 2;
* @return The bytes for source.
*/
com.google.protobuf.ByteString
getSourceBytes();
/**
* repeated .LogTag LogTags = 3;
*/
java.util.List
getLogTagsList();
/**
* repeated .LogTag LogTags = 3;
*/
PutLogRequest.LogTag getLogTags(int index);
/**
* repeated .LogTag LogTags = 3;
*/
int getLogTagsCount();
/**
* repeated .LogTag LogTags = 3;
*/
java.util.List extends PutLogRequest.LogTagOrBuilder>
getLogTagsOrBuilderList();
/**
* repeated .LogTag LogTags = 3;
*/
PutLogRequest.LogTagOrBuilder getLogTagsOrBuilder(
int index);
/**
* string FileName = 4;
* @return The fileName.
*/
java.lang.String getFileName();
/**
* string FileName = 4;
* @return The bytes for fileName.
*/
com.google.protobuf.ByteString
getFileNameBytes();
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The contextFlow.
*/
java.lang.String getContextFlow();
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The bytes for contextFlow.
*/
com.google.protobuf.ByteString
getContextFlowBytes();
}
/**
* Protobuf type {@code LogGroup}
*/
public static final class LogGroup extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:LogGroup)
LogGroupOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogGroup.newBuilder() to construct.
private LogGroup(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogGroup() {
logs_ = java.util.Collections.emptyList();
source_ = "";
logTags_ = java.util.Collections.emptyList();
fileName_ = "";
contextFlow_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogGroup();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogGroup(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logs_.add(
input.readMessage(PutLogRequest.Log.parser(), extensionRegistry));
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
source_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
logTags_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
logTags_.add(
input.readMessage(PutLogRequest.LogTag.parser(), extensionRegistry));
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
fileName_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
contextFlow_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
logTags_ = java.util.Collections.unmodifiableList(logTags_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogGroup.class, PutLogRequest.LogGroup.Builder.class);
}
public static final int LOGS_FIELD_NUMBER = 1;
private java.util.List logs_;
/**
* repeated .Log Logs = 1;
*/
@java.lang.Override
public java.util.List getLogsList() {
return logs_;
}
/**
* repeated .Log Logs = 1;
*/
@java.lang.Override
public java.util.List extends PutLogRequest.LogOrBuilder>
getLogsOrBuilderList() {
return logs_;
}
/**
* repeated .Log Logs = 1;
*/
@java.lang.Override
public int getLogsCount() {
return logs_.size();
}
/**
* repeated .Log Logs = 1;
*/
@java.lang.Override
public PutLogRequest.Log getLogs(int index) {
return logs_.get(index);
}
/**
* repeated .Log Logs = 1;
*/
@java.lang.Override
public PutLogRequest.LogOrBuilder getLogsOrBuilder(
int index) {
return logs_.get(index);
}
public static final int SOURCE_FIELD_NUMBER = 2;
private volatile java.lang.Object source_;
/**
* string Source = 2;
* @return The source.
*/
@java.lang.Override
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
}
}
/**
* string Source = 2;
* @return The bytes for source.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOGTAGS_FIELD_NUMBER = 3;
private java.util.List logTags_;
/**
* repeated .LogTag LogTags = 3;
*/
@java.lang.Override
public java.util.List getLogTagsList() {
return logTags_;
}
/**
* repeated .LogTag LogTags = 3;
*/
@java.lang.Override
public java.util.List extends PutLogRequest.LogTagOrBuilder>
getLogTagsOrBuilderList() {
return logTags_;
}
/**
* repeated .LogTag LogTags = 3;
*/
@java.lang.Override
public int getLogTagsCount() {
return logTags_.size();
}
/**
* repeated .LogTag LogTags = 3;
*/
@java.lang.Override
public PutLogRequest.LogTag getLogTags(int index) {
return logTags_.get(index);
}
/**
* repeated .LogTag LogTags = 3;
*/
@java.lang.Override
public PutLogRequest.LogTagOrBuilder getLogTagsOrBuilder(
int index) {
return logTags_.get(index);
}
public static final int FILENAME_FIELD_NUMBER = 4;
private volatile java.lang.Object fileName_;
/**
* string FileName = 4;
* @return The fileName.
*/
@java.lang.Override
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
}
}
/**
* string FileName = 4;
* @return The bytes for fileName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTEXTFLOW_FIELD_NUMBER = 5;
private volatile java.lang.Object contextFlow_;
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The contextFlow.
*/
@java.lang.Override
public java.lang.String getContextFlow() {
java.lang.Object ref = contextFlow_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextFlow_ = s;
return s;
}
}
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The bytes for contextFlow.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContextFlowBytes() {
java.lang.Object ref = contextFlow_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextFlow_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < logs_.size(); i++) {
output.writeMessage(1, logs_.get(i));
}
if (!getSourceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, source_);
}
for (int i = 0; i < logTags_.size(); i++) {
output.writeMessage(3, logTags_.get(i));
}
if (!getFileNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, fileName_);
}
if (!getContextFlowBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, contextFlow_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < logs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, logs_.get(i));
}
if (!getSourceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, source_);
}
for (int i = 0; i < logTags_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, logTags_.get(i));
}
if (!getFileNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, fileName_);
}
if (!getContextFlowBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, contextFlow_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof PutLogRequest.LogGroup)) {
return super.equals(obj);
}
PutLogRequest.LogGroup other = (PutLogRequest.LogGroup) obj;
if (!getLogsList()
.equals(other.getLogsList())) return false;
if (!getSource()
.equals(other.getSource())) return false;
if (!getLogTagsList()
.equals(other.getLogTagsList())) return false;
if (!getFileName()
.equals(other.getFileName())) return false;
if (!getContextFlow()
.equals(other.getContextFlow())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getLogsCount() > 0) {
hash = (37 * hash) + LOGS_FIELD_NUMBER;
hash = (53 * hash) + getLogsList().hashCode();
}
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
if (getLogTagsCount() > 0) {
hash = (37 * hash) + LOGTAGS_FIELD_NUMBER;
hash = (53 * hash) + getLogTagsList().hashCode();
}
hash = (37 * hash) + FILENAME_FIELD_NUMBER;
hash = (53 * hash) + getFileName().hashCode();
hash = (37 * hash) + CONTEXTFLOW_FIELD_NUMBER;
hash = (53 * hash) + getContextFlow().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static PutLogRequest.LogGroup parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroup parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroup parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroup parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroup parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroup parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroup parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroup parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogGroup parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroup parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogGroup parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroup parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(PutLogRequest.LogGroup prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code LogGroup}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:LogGroup)
PutLogRequest.LogGroupOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogGroup.class, PutLogRequest.LogGroup.Builder.class);
}
// Construct using PutLogRequest.LogGroup.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogsFieldBuilder();
getLogTagsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logsBuilder_.clear();
}
source_ = "";
if (logTagsBuilder_ == null) {
logTags_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
logTagsBuilder_.clear();
}
fileName_ = "";
contextFlow_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return PutLogRequest.internal_static_pb_LogGroup_descriptor;
}
@java.lang.Override
public PutLogRequest.LogGroup getDefaultInstanceForType() {
return PutLogRequest.LogGroup.getDefaultInstance();
}
@java.lang.Override
public PutLogRequest.LogGroup build() {
PutLogRequest.LogGroup result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public PutLogRequest.LogGroup buildPartial() {
PutLogRequest.LogGroup result = new PutLogRequest.LogGroup(this);
int from_bitField0_ = bitField0_;
if (logsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logs_ = logs_;
} else {
result.logs_ = logsBuilder_.build();
}
result.source_ = source_;
if (logTagsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
logTags_ = java.util.Collections.unmodifiableList(logTags_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.logTags_ = logTags_;
} else {
result.logTags_ = logTagsBuilder_.build();
}
result.fileName_ = fileName_;
result.contextFlow_ = contextFlow_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof PutLogRequest.LogGroup) {
return mergeFrom((PutLogRequest.LogGroup)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(PutLogRequest.LogGroup other) {
if (other == PutLogRequest.LogGroup.getDefaultInstance()) return this;
if (logsBuilder_ == null) {
if (!other.logs_.isEmpty()) {
if (logs_.isEmpty()) {
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogsIsMutable();
logs_.addAll(other.logs_);
}
onChanged();
}
} else {
if (!other.logs_.isEmpty()) {
if (logsBuilder_.isEmpty()) {
logsBuilder_.dispose();
logsBuilder_ = null;
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
logsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogsFieldBuilder() : null;
} else {
logsBuilder_.addAllMessages(other.logs_);
}
}
}
if (!other.getSource().isEmpty()) {
source_ = other.source_;
onChanged();
}
if (logTagsBuilder_ == null) {
if (!other.logTags_.isEmpty()) {
if (logTags_.isEmpty()) {
logTags_ = other.logTags_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureLogTagsIsMutable();
logTags_.addAll(other.logTags_);
}
onChanged();
}
} else {
if (!other.logTags_.isEmpty()) {
if (logTagsBuilder_.isEmpty()) {
logTagsBuilder_.dispose();
logTagsBuilder_ = null;
logTags_ = other.logTags_;
bitField0_ = (bitField0_ & ~0x00000002);
logTagsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogTagsFieldBuilder() : null;
} else {
logTagsBuilder_.addAllMessages(other.logTags_);
}
}
}
if (!other.getFileName().isEmpty()) {
fileName_ = other.fileName_;
onChanged();
}
if (!other.getContextFlow().isEmpty()) {
contextFlow_ = other.contextFlow_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
PutLogRequest.LogGroup parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (PutLogRequest.LogGroup) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List logs_ =
java.util.Collections.emptyList();
private void ensureLogsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList(logs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.Log, PutLogRequest.Log.Builder, PutLogRequest.LogOrBuilder> logsBuilder_;
/**
* repeated .Log Logs = 1;
*/
public java.util.List getLogsList() {
if (logsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logs_);
} else {
return logsBuilder_.getMessageList();
}
}
/**
* repeated .Log Logs = 1;
*/
public int getLogsCount() {
if (logsBuilder_ == null) {
return logs_.size();
} else {
return logsBuilder_.getCount();
}
}
/**
* repeated .Log Logs = 1;
*/
public PutLogRequest.Log getLogs(int index) {
if (logsBuilder_ == null) {
return logs_.get(index);
} else {
return logsBuilder_.getMessage(index);
}
}
/**
* repeated .Log Logs = 1;
*/
public Builder setLogs(
int index, PutLogRequest.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.set(index, value);
onChanged();
} else {
logsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder setLogs(
int index, PutLogRequest.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.set(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder addLogs(PutLogRequest.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(value);
onChanged();
} else {
logsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder addLogs(
int index, PutLogRequest.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(index, value);
onChanged();
} else {
logsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder addLogs(
PutLogRequest.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder addLogs(
int index, PutLogRequest.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder addAllLogs(
java.lang.Iterable extends PutLogRequest.Log> values) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logs_);
onChanged();
} else {
logsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder clearLogs() {
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logsBuilder_.clear();
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public Builder removeLogs(int index) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.remove(index);
onChanged();
} else {
logsBuilder_.remove(index);
}
return this;
}
/**
* repeated .Log Logs = 1;
*/
public PutLogRequest.Log.Builder getLogsBuilder(
int index) {
return getLogsFieldBuilder().getBuilder(index);
}
/**
* repeated .Log Logs = 1;
*/
public PutLogRequest.LogOrBuilder getLogsOrBuilder(
int index) {
if (logsBuilder_ == null) {
return logs_.get(index); } else {
return logsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Log Logs = 1;
*/
public java.util.List extends PutLogRequest.LogOrBuilder>
getLogsOrBuilderList() {
if (logsBuilder_ != null) {
return logsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logs_);
}
}
/**
* repeated .Log Logs = 1;
*/
public PutLogRequest.Log.Builder addLogsBuilder() {
return getLogsFieldBuilder().addBuilder(
PutLogRequest.Log.getDefaultInstance());
}
/**
* repeated .Log Logs = 1;
*/
public PutLogRequest.Log.Builder addLogsBuilder(
int index) {
return getLogsFieldBuilder().addBuilder(
index, PutLogRequest.Log.getDefaultInstance());
}
/**
* repeated .Log Logs = 1;
*/
public java.util.List
getLogsBuilderList() {
return getLogsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.Log, PutLogRequest.Log.Builder, PutLogRequest.LogOrBuilder>
getLogsFieldBuilder() {
if (logsBuilder_ == null) {
logsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.Log, PutLogRequest.Log.Builder, PutLogRequest.LogOrBuilder>(
logs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
logs_ = null;
}
return logsBuilder_;
}
private java.lang.Object source_ = "";
/**
* string Source = 2;
* @return The source.
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string Source = 2;
* @return The bytes for source.
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string Source = 2;
* @param value The source to set.
* @return This builder for chaining.
*/
public Builder setSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
source_ = value;
onChanged();
return this;
}
/**
* string Source = 2;
* @return This builder for chaining.
*/
public Builder clearSource() {
source_ = getDefaultInstance().getSource();
onChanged();
return this;
}
/**
* string Source = 2;
* @param value The bytes for source to set.
* @return This builder for chaining.
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
source_ = value;
onChanged();
return this;
}
private java.util.List logTags_ =
java.util.Collections.emptyList();
private void ensureLogTagsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
logTags_ = new java.util.ArrayList(logTags_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogTag, PutLogRequest.LogTag.Builder, PutLogRequest.LogTagOrBuilder> logTagsBuilder_;
/**
* repeated .LogTag LogTags = 3;
*/
public java.util.List getLogTagsList() {
if (logTagsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logTags_);
} else {
return logTagsBuilder_.getMessageList();
}
}
/**
* repeated .LogTag LogTags = 3;
*/
public int getLogTagsCount() {
if (logTagsBuilder_ == null) {
return logTags_.size();
} else {
return logTagsBuilder_.getCount();
}
}
/**
* repeated .LogTag LogTags = 3;
*/
public PutLogRequest.LogTag getLogTags(int index) {
if (logTagsBuilder_ == null) {
return logTags_.get(index);
} else {
return logTagsBuilder_.getMessage(index);
}
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder setLogTags(
int index, PutLogRequest.LogTag value) {
if (logTagsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogTagsIsMutable();
logTags_.set(index, value);
onChanged();
} else {
logTagsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder setLogTags(
int index, PutLogRequest.LogTag.Builder builderForValue) {
if (logTagsBuilder_ == null) {
ensureLogTagsIsMutable();
logTags_.set(index, builderForValue.build());
onChanged();
} else {
logTagsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder addLogTags(PutLogRequest.LogTag value) {
if (logTagsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogTagsIsMutable();
logTags_.add(value);
onChanged();
} else {
logTagsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder addLogTags(
int index, PutLogRequest.LogTag value) {
if (logTagsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogTagsIsMutable();
logTags_.add(index, value);
onChanged();
} else {
logTagsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder addLogTags(
PutLogRequest.LogTag.Builder builderForValue) {
if (logTagsBuilder_ == null) {
ensureLogTagsIsMutable();
logTags_.add(builderForValue.build());
onChanged();
} else {
logTagsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder addLogTags(
int index, PutLogRequest.LogTag.Builder builderForValue) {
if (logTagsBuilder_ == null) {
ensureLogTagsIsMutable();
logTags_.add(index, builderForValue.build());
onChanged();
} else {
logTagsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder addAllLogTags(
java.lang.Iterable extends PutLogRequest.LogTag> values) {
if (logTagsBuilder_ == null) {
ensureLogTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logTags_);
onChanged();
} else {
logTagsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder clearLogTags() {
if (logTagsBuilder_ == null) {
logTags_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
logTagsBuilder_.clear();
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public Builder removeLogTags(int index) {
if (logTagsBuilder_ == null) {
ensureLogTagsIsMutable();
logTags_.remove(index);
onChanged();
} else {
logTagsBuilder_.remove(index);
}
return this;
}
/**
* repeated .LogTag LogTags = 3;
*/
public PutLogRequest.LogTag.Builder getLogTagsBuilder(
int index) {
return getLogTagsFieldBuilder().getBuilder(index);
}
/**
* repeated .LogTag LogTags = 3;
*/
public PutLogRequest.LogTagOrBuilder getLogTagsOrBuilder(
int index) {
if (logTagsBuilder_ == null) {
return logTags_.get(index); } else {
return logTagsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .LogTag LogTags = 3;
*/
public java.util.List extends PutLogRequest.LogTagOrBuilder>
getLogTagsOrBuilderList() {
if (logTagsBuilder_ != null) {
return logTagsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logTags_);
}
}
/**
* repeated .LogTag LogTags = 3;
*/
public PutLogRequest.LogTag.Builder addLogTagsBuilder() {
return getLogTagsFieldBuilder().addBuilder(
PutLogRequest.LogTag.getDefaultInstance());
}
/**
* repeated .LogTag LogTags = 3;
*/
public PutLogRequest.LogTag.Builder addLogTagsBuilder(
int index) {
return getLogTagsFieldBuilder().addBuilder(
index, PutLogRequest.LogTag.getDefaultInstance());
}
/**
* repeated .LogTag LogTags = 3;
*/
public java.util.List
getLogTagsBuilderList() {
return getLogTagsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogTag, PutLogRequest.LogTag.Builder, PutLogRequest.LogTagOrBuilder>
getLogTagsFieldBuilder() {
if (logTagsBuilder_ == null) {
logTagsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogTag, PutLogRequest.LogTag.Builder, PutLogRequest.LogTagOrBuilder>(
logTags_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
logTags_ = null;
}
return logTagsBuilder_;
}
private java.lang.Object fileName_ = "";
/**
* string FileName = 4;
* @return The fileName.
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string FileName = 4;
* @return The bytes for fileName.
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string FileName = 4;
* @param value The fileName to set.
* @return This builder for chaining.
*/
public Builder setFileName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fileName_ = value;
onChanged();
return this;
}
/**
* string FileName = 4;
* @return This builder for chaining.
*/
public Builder clearFileName() {
fileName_ = getDefaultInstance().getFileName();
onChanged();
return this;
}
/**
* string FileName = 4;
* @param value The bytes for fileName to set.
* @return This builder for chaining.
*/
public Builder setFileNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fileName_ = value;
onChanged();
return this;
}
private java.lang.Object contextFlow_ = "";
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The contextFlow.
*/
public java.lang.String getContextFlow() {
java.lang.Object ref = contextFlow_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextFlow_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return The bytes for contextFlow.
*/
public com.google.protobuf.ByteString
getContextFlowBytes() {
java.lang.Object ref = contextFlow_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextFlow_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @param value The contextFlow to set.
* @return This builder for chaining.
*/
public Builder setContextFlow(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contextFlow_ = value;
onChanged();
return this;
}
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @return This builder for chaining.
*/
public Builder clearContextFlow() {
contextFlow_ = getDefaultInstance().getContextFlow();
onChanged();
return this;
}
/**
*
*该字段暂无效用
*
*
* string ContextFlow = 5;
* @param value The bytes for contextFlow to set.
* @return This builder for chaining.
*/
public Builder setContextFlowBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contextFlow_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:LogGroup)
}
// @@protoc_insertion_point(class_scope:LogGroup)
private static final PutLogRequest.LogGroup DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PutLogRequest.LogGroup();
}
public static PutLogRequest.LogGroup getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogGroup parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogGroup(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public PutLogRequest.LogGroup getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogGroupListOrBuilder extends
// @@protoc_insertion_point(interface_extends:LogGroupList)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .LogGroup LogGroups = 1;
*/
java.util.List
getLogGroupsList();
/**
* repeated .LogGroup LogGroups = 1;
*/
PutLogRequest.LogGroup getLogGroups(int index);
/**
* repeated .LogGroup LogGroups = 1;
*/
int getLogGroupsCount();
/**
* repeated .LogGroup LogGroups = 1;
*/
java.util.List extends PutLogRequest.LogGroupOrBuilder>
getLogGroupsOrBuilderList();
/**
* repeated .LogGroup LogGroups = 1;
*/
PutLogRequest.LogGroupOrBuilder getLogGroupsOrBuilder(
int index);
}
/**
* Protobuf type {@code LogGroupList}
*/
public static final class LogGroupList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:LogGroupList)
LogGroupListOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogGroupList.newBuilder() to construct.
private LogGroupList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogGroupList() {
logGroups_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogGroupList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogGroupList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
logGroups_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logGroups_.add(
input.readMessage(PutLogRequest.LogGroup.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
logGroups_ = java.util.Collections.unmodifiableList(logGroups_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogGroupList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogGroupList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogGroupList.class, PutLogRequest.LogGroupList.Builder.class);
}
public static final int LOGGROUPS_FIELD_NUMBER = 1;
private java.util.List logGroups_;
/**
* repeated .LogGroup LogGroups = 1;
*/
@java.lang.Override
public java.util.List getLogGroupsList() {
return logGroups_;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
@java.lang.Override
public java.util.List extends PutLogRequest.LogGroupOrBuilder>
getLogGroupsOrBuilderList() {
return logGroups_;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
@java.lang.Override
public int getLogGroupsCount() {
return logGroups_.size();
}
/**
* repeated .LogGroup LogGroups = 1;
*/
@java.lang.Override
public PutLogRequest.LogGroup getLogGroups(int index) {
return logGroups_.get(index);
}
/**
* repeated .LogGroup LogGroups = 1;
*/
@java.lang.Override
public PutLogRequest.LogGroupOrBuilder getLogGroupsOrBuilder(
int index) {
return logGroups_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < logGroups_.size(); i++) {
output.writeMessage(1, logGroups_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < logGroups_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, logGroups_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof PutLogRequest.LogGroupList)) {
return super.equals(obj);
}
PutLogRequest.LogGroupList other = (PutLogRequest.LogGroupList) obj;
if (!getLogGroupsList()
.equals(other.getLogGroupsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getLogGroupsCount() > 0) {
hash = (37 * hash) + LOGGROUPS_FIELD_NUMBER;
hash = (53 * hash) + getLogGroupsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static PutLogRequest.LogGroupList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroupList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroupList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroupList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroupList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static PutLogRequest.LogGroupList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static PutLogRequest.LogGroupList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroupList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogGroupList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroupList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static PutLogRequest.LogGroupList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static PutLogRequest.LogGroupList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(PutLogRequest.LogGroupList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code LogGroupList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:LogGroupList)
PutLogRequest.LogGroupListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return PutLogRequest.internal_static_pb_LogGroupList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return PutLogRequest.internal_static_pb_LogGroupList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
PutLogRequest.LogGroupList.class, PutLogRequest.LogGroupList.Builder.class);
}
// Construct using PutLogRequest.LogGroupList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogGroupsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (logGroupsBuilder_ == null) {
logGroups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logGroupsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return PutLogRequest.internal_static_pb_LogGroupList_descriptor;
}
@java.lang.Override
public PutLogRequest.LogGroupList getDefaultInstanceForType() {
return PutLogRequest.LogGroupList.getDefaultInstance();
}
@java.lang.Override
public PutLogRequest.LogGroupList build() {
PutLogRequest.LogGroupList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public PutLogRequest.LogGroupList buildPartial() {
PutLogRequest.LogGroupList result = new PutLogRequest.LogGroupList(this);
int from_bitField0_ = bitField0_;
if (logGroupsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
logGroups_ = java.util.Collections.unmodifiableList(logGroups_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logGroups_ = logGroups_;
} else {
result.logGroups_ = logGroupsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof PutLogRequest.LogGroupList) {
return mergeFrom((PutLogRequest.LogGroupList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(PutLogRequest.LogGroupList other) {
if (other == PutLogRequest.LogGroupList.getDefaultInstance()) return this;
if (logGroupsBuilder_ == null) {
if (!other.logGroups_.isEmpty()) {
if (logGroups_.isEmpty()) {
logGroups_ = other.logGroups_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogGroupsIsMutable();
logGroups_.addAll(other.logGroups_);
}
onChanged();
}
} else {
if (!other.logGroups_.isEmpty()) {
if (logGroupsBuilder_.isEmpty()) {
logGroupsBuilder_.dispose();
logGroupsBuilder_ = null;
logGroups_ = other.logGroups_;
bitField0_ = (bitField0_ & ~0x00000001);
logGroupsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogGroupsFieldBuilder() : null;
} else {
logGroupsBuilder_.addAllMessages(other.logGroups_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
PutLogRequest.LogGroupList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (PutLogRequest.LogGroupList) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List logGroups_ =
java.util.Collections.emptyList();
private void ensureLogGroupsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
logGroups_ = new java.util.ArrayList(logGroups_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogGroup, PutLogRequest.LogGroup.Builder, PutLogRequest.LogGroupOrBuilder> logGroupsBuilder_;
/**
* repeated .LogGroup LogGroups = 1;
*/
public java.util.List getLogGroupsList() {
if (logGroupsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logGroups_);
} else {
return logGroupsBuilder_.getMessageList();
}
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public int getLogGroupsCount() {
if (logGroupsBuilder_ == null) {
return logGroups_.size();
} else {
return logGroupsBuilder_.getCount();
}
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public PutLogRequest.LogGroup getLogGroups(int index) {
if (logGroupsBuilder_ == null) {
return logGroups_.get(index);
} else {
return logGroupsBuilder_.getMessage(index);
}
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder setLogGroups(
int index, PutLogRequest.LogGroup value) {
if (logGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogGroupsIsMutable();
logGroups_.set(index, value);
onChanged();
} else {
logGroupsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder setLogGroups(
int index, PutLogRequest.LogGroup.Builder builderForValue) {
if (logGroupsBuilder_ == null) {
ensureLogGroupsIsMutable();
logGroups_.set(index, builderForValue.build());
onChanged();
} else {
logGroupsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder addLogGroups(PutLogRequest.LogGroup value) {
if (logGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogGroupsIsMutable();
logGroups_.add(value);
onChanged();
} else {
logGroupsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder addLogGroups(
int index, PutLogRequest.LogGroup value) {
if (logGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogGroupsIsMutable();
logGroups_.add(index, value);
onChanged();
} else {
logGroupsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder addLogGroups(
PutLogRequest.LogGroup.Builder builderForValue) {
if (logGroupsBuilder_ == null) {
ensureLogGroupsIsMutable();
logGroups_.add(builderForValue.build());
onChanged();
} else {
logGroupsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder addLogGroups(
int index, PutLogRequest.LogGroup.Builder builderForValue) {
if (logGroupsBuilder_ == null) {
ensureLogGroupsIsMutable();
logGroups_.add(index, builderForValue.build());
onChanged();
} else {
logGroupsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder addAllLogGroups(
java.lang.Iterable extends PutLogRequest.LogGroup> values) {
if (logGroupsBuilder_ == null) {
ensureLogGroupsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logGroups_);
onChanged();
} else {
logGroupsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder clearLogGroups() {
if (logGroupsBuilder_ == null) {
logGroups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logGroupsBuilder_.clear();
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public Builder removeLogGroups(int index) {
if (logGroupsBuilder_ == null) {
ensureLogGroupsIsMutable();
logGroups_.remove(index);
onChanged();
} else {
logGroupsBuilder_.remove(index);
}
return this;
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public PutLogRequest.LogGroup.Builder getLogGroupsBuilder(
int index) {
return getLogGroupsFieldBuilder().getBuilder(index);
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public PutLogRequest.LogGroupOrBuilder getLogGroupsOrBuilder(
int index) {
if (logGroupsBuilder_ == null) {
return logGroups_.get(index); } else {
return logGroupsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public java.util.List extends PutLogRequest.LogGroupOrBuilder>
getLogGroupsOrBuilderList() {
if (logGroupsBuilder_ != null) {
return logGroupsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logGroups_);
}
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public PutLogRequest.LogGroup.Builder addLogGroupsBuilder() {
return getLogGroupsFieldBuilder().addBuilder(
PutLogRequest.LogGroup.getDefaultInstance());
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public PutLogRequest.LogGroup.Builder addLogGroupsBuilder(
int index) {
return getLogGroupsFieldBuilder().addBuilder(
index, PutLogRequest.LogGroup.getDefaultInstance());
}
/**
* repeated .LogGroup LogGroups = 1;
*/
public java.util.List
getLogGroupsBuilderList() {
return getLogGroupsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogGroup, PutLogRequest.LogGroup.Builder, PutLogRequest.LogGroupOrBuilder>
getLogGroupsFieldBuilder() {
if (logGroupsBuilder_ == null) {
logGroupsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
PutLogRequest.LogGroup, PutLogRequest.LogGroup.Builder, PutLogRequest.LogGroupOrBuilder>(
logGroups_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
logGroups_ = null;
}
return logGroupsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:LogGroupList)
}
// @@protoc_insertion_point(class_scope:LogGroupList)
private static final PutLogRequest.LogGroupList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PutLogRequest.LogGroupList();
}
public static PutLogRequest.LogGroupList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogGroupList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogGroupList(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public PutLogRequest.LogGroupList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_pb_LogContent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_pb_LogContent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_pb_Log_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_pb_Log_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_pb_LogTag_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_pb_LogTag_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_pb_LogGroup_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_pb_LogGroup_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_pb_LogGroupList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_pb_LogGroupList_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\023PutLogRequest.proto\022\002pb\"(\n\nLogContent\022" +
"\013\n\003Key\030\001 \001(\t\022\r\n\005Value\030\002 \001(\t\"5\n\003Log\022\014\n\004Ti" +
"me\030\001 \001(\003\022 \n\010Contents\030\002 \003(\0132\016.LogConte" +
"nt\"$\n\006LogTag\022\013\n\003Key\030\001 \001(\t\022\r\n\005Value\030\002 \001(\t" +
"\"u\n\010LogGroup\022\025\n\004Logs\030\001 \003(\0132\007.Log\022\016\n\006S" +
"ource\030\002 \001(\t\022\033\n\007LogTags\030\003 \003(\0132\n.LogTag" +
"\022\020\n\010FileName\030\004 \001(\t\022\023\n\013ContextFlow\030\005 \001(\t\"" +
"/\n\014LogGroupList\022\037\n\tLogGroups\030\001 \003(\0132\014." +
"LogGroupB\007Z\005./;pbb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_pb_LogContent_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_pb_LogContent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_pb_LogContent_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_pb_Log_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_pb_Log_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_pb_Log_descriptor,
new java.lang.String[] { "Time", "Contents", });
internal_static_pb_LogTag_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_pb_LogTag_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_pb_LogTag_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_pb_LogGroup_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_pb_LogGroup_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_pb_LogGroup_descriptor,
new java.lang.String[] { "Logs", "Source", "LogTags", "FileName", "ContextFlow", });
internal_static_pb_LogGroupList_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_pb_LogGroupList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_pb_LogGroupList_descriptor,
new java.lang.String[] { "LogGroups", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy