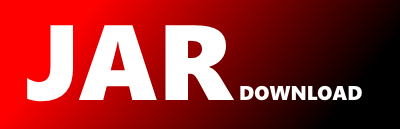
com.volcengine.service.im.ImTrait Maven / Gradle / Ivy
Show all versions of volc-sdk-java Show documentation
package com.volcengine.service.im;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.annotation.JSONField;
import com.volcengine.helper.Const;
import com.volcengine.model.ServiceInfo;
import com.volcengine.model.im.*;
import com.volcengine.model.response.RawResponse;
import com.volcengine.model.response.ResponseMetadata;
import com.volcengine.service.BaseServiceImpl;
import lombok.Data;
/**
* Im Trait
*/
public class ImTrait extends BaseServiceImpl {
protected ImTrait() {
super(ImConfig.serviceInfoMap.get(Const.REGION_CN_NORTH_1), ImConfig.apiInfoList);
}
protected ImTrait(ServiceInfo serviceInfo) {
super(serviceInfo, ImConfig.apiInfoList);
}
@Data
static public class ResponseModel {
@JSONField(name = "ResponseMetadata")
private ResponseMetadata responseMetadata;
}
private T parseRawResponse(RawResponse rawResponse, Class type) throws Exception {
Exception ex = (rawResponse.getException());
if (ex != null) {
throw ex;
}
byte[] data = rawResponse.getData();
if (data == null) {
throw new Exception("null response body got, rawResponse:" + JSON.toJSONString(rawResponse));
}
return JSON.parseObject(data, type);
}
/**
* getConversationMarks
* 会话标记查询
*
* 你可以调用GetConversationMarks接口,指定你所属的AppId、会话成员 ID 和标记类型进行会话标记。目前仅直播群支持
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetConversationMarksRes getConversationMarks(GetConversationMarksBody body) throws Exception {
RawResponse rawResponse = json("GetConversationMarks", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetConversationMarksRes.class);
}
/**
* markConversation
* 标记会话
*
* 你可以调用MarkConversation接口,指定你所属的AppId、会话成员 ID 和标记类型进行给会话标记。目前仅直播群支持
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public MarkConversationRes markConversation(MarkConversationBody body) throws Exception {
RawResponse rawResponse = json("MarkConversation", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, MarkConversationRes.class);
}
/**
* modifyParticipantReadIndex
* 修改会话成员已读位置
*
* 你可以使用此接口修改会话成员的已读位置。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ModifyParticipantReadIndexRes modifyParticipantReadIndex(ModifyParticipantReadIndexBody body) throws Exception {
RawResponse rawResponse = json("ModifyParticipantReadIndex", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ModifyParticipantReadIndexRes.class);
}
/**
* getMessagesReadReceipt
* 获取消息的已读回执详情
*
* 你可以调用GetMessagesReadReceipt接口,指定你所属的AppId、会话 Id 和消息Id获得消息的已读详情
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetMessagesReadReceiptRes getMessagesReadReceipt(GetMessagesReadReceiptBody body) throws Exception {
RawResponse rawResponse = json("GetMessagesReadReceipt", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetMessagesReadReceiptRes.class);
}
/**
* scanConversationParticipantList
* 分批扫描群聊中的成员详细信
*
* 你可以使用此接口分批扫描群中成员的详细信息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ScanConversationParticipantListRes scanConversationParticipantList(ScanConversationParticipantListBody body) throws Exception {
RawResponse rawResponse = json("ScanConversationParticipantList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ScanConversationParticipantListRes.class);
}
/**
* batchGetBlockParticipants
* 分批获得禁言/拉黑成员列表
*
* 你可以使用此接口分批在直播群中获得禁言或拉黑成员列表。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchGetBlockParticipantsRes batchGetBlockParticipants(BatchGetBlockParticipantsBody body) throws Exception {
RawResponse rawResponse = json("BatchGetBlockParticipants", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchGetBlockParticipantsRes.class);
}
/**
* isUserInConversation
* 判断用户是否在会话中
*
* 你可以使用此接口判断用户是否在会话中。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public IsUserInConversationRes isUserInConversation(IsUserInConversationBody body) throws Exception {
RawResponse rawResponse = json("IsUserInConversation", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, IsUserInConversationRes.class);
}
/**
* batchModifyConversationParticipant
* 批量修改群聊成员详情
*
* 你可以使用此接口批量对群成员的详细信息进行修改。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchModifyConversationParticipantRes batchModifyConversationParticipant(BatchModifyConversationParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchModifyConversationParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchModifyConversationParticipantRes.class);
}
/**
* batchDeleteConversationParticipant
* 批量删除群聊成员
*
* 你可以使用此接口批量删除群成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchDeleteConversationParticipantRes batchDeleteConversationParticipant(BatchDeleteConversationParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchDeleteConversationParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchDeleteConversationParticipantRes.class);
}
/**
* batchDeleteBlockParticipants
* 批量取消禁言/取消拉黑会话成员
*
* 你可以使用此接口在直播群中批量取消禁言或取消拉黑会话成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchDeleteBlockParticipantsRes batchDeleteBlockParticipants(BatchDeleteBlockParticipantsBody body) throws Exception {
RawResponse rawResponse = json("BatchDeleteBlockParticipants", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchDeleteBlockParticipantsRes.class);
}
/**
* batchGetConversationParticipant
* 批量查询会话成员详细信息
*
* 你可以使用此接口批量查询会话成员的详细信息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchGetConversationParticipantRes batchGetConversationParticipant(BatchGetConversationParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchGetConversationParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchGetConversationParticipantRes.class);
}
/**
* batchGetWhitelistParticipant
* 批量查询全员禁言下白名单成员
*
* 你可以调用BatchGetWhitelistParticipant接口,指定你所属的AppId、会话 ID、 查询起始位置和查询条数批量查询全员禁言下白名单成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchGetWhitelistParticipantRes batchGetWhitelistParticipant(BatchGetWhitelistParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchGetWhitelistParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchGetWhitelistParticipantRes.class);
}
/**
* batchAddWhitelistParticipant
* 批量添加全员禁言下白名单成员
*
* 你可以使用此接口批量添加全员禁言下可发言白名单成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchAddWhitelistParticipantRes batchAddWhitelistParticipant(BatchAddWhitelistParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchAddWhitelistParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchAddWhitelistParticipantRes.class);
}
/**
* batchAddManager
* 批量添加直播群管理员
*
* 你可以使用此接口向直播群中批量添加管理员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchAddManagerRes batchAddManager(BatchAddManagerBody body) throws Exception {
RawResponse rawResponse = json("BatchAddManager", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchAddManagerRes.class);
}
/**
* batchAddConversationParticipant
* 批量添加群聊成员
*
* 你可以使用此接口向群聊中批量添加群成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchAddConversationParticipantRes batchAddConversationParticipant(BatchAddConversationParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchAddConversationParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchAddConversationParticipantRes.class);
}
/**
* batchAddBlockParticipants
* 批量禁言/拉黑会话成员
*
* 你可以使用此接口在直播群中批量禁言或拉黑会话成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchAddBlockParticipantsRes batchAddBlockParticipants(BatchAddBlockParticipantsBody body) throws Exception {
RawResponse rawResponse = json("BatchAddBlockParticipants", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchAddBlockParticipantsRes.class);
}
/**
* batchRemoveWhitelistParticipant
* 批量移除全员禁言下白名单成员
*
* 你可以使用此接口批量移除全员禁言下白名单成员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchRemoveWhitelistParticipantRes batchRemoveWhitelistParticipant(BatchRemoveWhitelistParticipantBody body) throws Exception {
RawResponse rawResponse = json("BatchRemoveWhitelistParticipant", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchRemoveWhitelistParticipantRes.class);
}
/**
* batchRemoveManager
* 批量移除直播群管理员
*
* 你可以使用此接口批量移除直播群中的管理员。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchRemoveManagerRes batchRemoveManager(BatchRemoveManagerBody body) throws Exception {
RawResponse rawResponse = json("BatchRemoveManager", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchRemoveManagerRes.class);
}
/**
* batchUpdateLiveParticipants
* 更新直播群成员资料
*
* 你可以调用 BatchUpdateLiveParticipants 接口,指定你所属的AppId、会话 Id、 直播群成员 ID 来更新群成员资料。
*
*
*
* 目前仅直播群支持此接口
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchUpdateLiveParticipantsRes batchUpdateLiveParticipants(BatchUpdateLiveParticipantsBody body) throws Exception {
RawResponse rawResponse = json("BatchUpdateLiveParticipants", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchUpdateLiveParticipantsRes.class);
}
/**
* getParticipantReadIndex
* 查询会话成员已读位置
*
* 你可以使用此接口查询会话中所有成员的已读位置。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetParticipantReadIndexRes getParticipantReadIndex(GetParticipantReadIndexBody body) throws Exception {
RawResponse rawResponse = json("GetParticipantReadIndex", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetParticipantReadIndexRes.class);
}
/**
* getConversationUserCount
* 查询会话成员数量
*
* 你可以使用此接口查询会话成员数量。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetConversationUserCountRes getConversationUserCount(GetConversationUserCountBody body) throws Exception {
RawResponse rawResponse = json("GetConversationUserCount", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetConversationUserCountRes.class);
}
/**
* queryLiveParticipantStatus
* 查询直播群成员状态
*
* 你可以使用此接口查询群成员在直播群的状态。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public QueryLiveParticipantStatusRes queryLiveParticipantStatus(QueryLiveParticipantStatusBody body) throws Exception {
RawResponse rawResponse = json("QueryLiveParticipantStatus", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, QueryLiveParticipantStatusRes.class);
}
/**
* modifyConversation
* 修改会话信息
*
* 你可以使用此接口修改会话信息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ModifyConversationRes modifyConversation(ModifyConversationBody body) throws Exception {
RawResponse rawResponse = json("ModifyConversation", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ModifyConversationRes.class);
}
/**
* modifyConversationSetting
* 修改用户对会话的设置
*
* 你可以使用此接口查询会话成员对会话的设置
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ModifyConversationSettingRes modifyConversationSetting(ModifyConversationSettingBody body) throws Exception {
RawResponse rawResponse = json("ModifyConversationSetting", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ModifyConversationSettingRes.class);
}
/**
* createConversation
* 创建会话
*
* 你可以使用此接口创建会话。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public CreateConversationRes createConversation(CreateConversationBody body) throws Exception {
RawResponse rawResponse = json("CreateConversation", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, CreateConversationRes.class);
}
/**
* batchGetConversations
* 批量查询会话
*
* 你可以使用此接口批量查询会话。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchGetConversationsRes batchGetConversations(BatchGetConversationsBody body) throws Exception {
RawResponse rawResponse = json("BatchGetConversations", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchGetConversationsRes.class);
}
/**
* getConversationSetting
* 查询成员对会话的设置
*
* 你可以使用此接口查询会话成员对会话的设置。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetConversationSettingRes getConversationSetting(GetConversationSettingBody body) throws Exception {
RawResponse rawResponse = json("GetConversationSetting", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetConversationSettingRes.class);
}
/**
* getUserConversations
* 查询用户拥有会话
*
* 你可以使用此接口查询某个成员拥有的会话。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetUserConversationsRes getUserConversations(GetUserConversationsBody body) throws Exception {
RawResponse rawResponse = json("GetUserConversations", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetUserConversationsRes.class);
}
/**
* destroyConversation
* 解散群聊
*
* 你可以使用此接口解散群聊。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public DestroyConversationRes destroyConversation(DestroyConversationBody body) throws Exception {
RawResponse rawResponse = json("DestroyConversation", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, DestroyConversationRes.class);
}
/**
* modifyMessage
* 修改消息扩展字段
*
* 你可以使用此接口修改消息扩展字段。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ModifyMessageRes modifyMessage(ModifyMessageBody body) throws Exception {
RawResponse rawResponse = json("ModifyMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ModifyMessageRes.class);
}
/**
* getConversationMessages
* 分批查询会话中的消息
*
* 你可以使用此接口批量查询会话中的消息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetConversationMessagesRes getConversationMessages(GetConversationMessagesBody body) throws Exception {
RawResponse rawResponse = json("GetConversationMessages", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetConversationMessagesRes.class);
}
/**
* deleteConversationMessage
* 删除会话消息
*
* 你可以使用此接口删除会话中的指定消息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public DeleteConversationMessageRes deleteConversationMessage(DeleteConversationMessageBody body) throws Exception {
RawResponse rawResponse = json("DeleteConversationMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, DeleteConversationMessageRes.class);
}
/**
* deleteMessage
* 删除用户消息
*
* 你可以使用此接口删除用户消息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public DeleteMessageRes deleteMessage(DeleteMessageBody body) throws Exception {
RawResponse rawResponse = json("DeleteMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, DeleteMessageRes.class);
}
/**
* sendMessage
* 发送消息
*
* 你可以调用SendMessage接口,指定你所属的AppId和,发送者 UserId、会话 ID、消息类型和消息内容,进行消息发送。
*
*
*
* 用户需要在会话中才允许消息发送,且不会触发第三方回调。
*
*
*
* 发送消息前不会检查发送方是否禁言,也不会检查会话是否开启禁言。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public SendMessageRes sendMessage(SendMessageBody body) throws Exception {
RawResponse rawResponse = json("SendMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, SendMessageRes.class);
}
/**
* getMessages
* 批量查询消息
*
* 你可以使用此接口指定消息 ID 对消息进行批量查询。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetMessagesRes getMessages(GetMessagesBody body) throws Exception {
RawResponse rawResponse = json("GetMessages", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetMessagesRes.class);
}
/**
* recallMessage
* 撤回用户消息
*
* 你可以使用此接口撤回用户的消息。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public RecallMessageRes recallMessage(RecallMessageBody body) throws Exception {
RawResponse rawResponse = json("RecallMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, RecallMessageRes.class);
}
/**
* deleteFriend
* 删除好友
*
* 你可以使用此接口删除好友。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public DeleteFriendRes deleteFriend(DeleteFriendBody body) throws Exception {
RawResponse rawResponse = json("DeleteFriend", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, DeleteFriendRes.class);
}
/**
* updateFriend
* 更新好友
*
* 你可以调用 UpdateFriend 接口,指定你所属的 AppId、用户 ID 和 好友 ID 更新好友信息。 一次最多支持更新 10 个好友。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public UpdateFriendRes updateFriend(UpdateFriendBody body) throws Exception {
RawResponse rawResponse = json("UpdateFriend", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, UpdateFriendRes.class);
}
/**
* updateBlackList
* 更新黑名单
*
* 你可以调用 UpdateBlackList 接口,指定你所属的 AppId、用户 ID 和黑名单中用户的 ID,更新黑名单用户的扩展信息。 一次最多支持更新 10 个好友。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public UpdateBlackListRes updateBlackList(UpdateBlackListBody body) throws Exception {
RawResponse rawResponse = json("UpdateBlackList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, UpdateBlackListRes.class);
}
/**
* listFriend
* 查询好友列表
*
* 你可以使用此接口查询指定用户的好友列表。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public ListFriendRes listFriend(ListFriendBody body) throws Exception {
RawResponse rawResponse = json("ListFriend", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, ListFriendRes.class);
}
/**
* queryOnlineStatus
* 查询用户在线状态
*
* 你可以通过QueryOnlineStatus接口,指定 AppId 和 UserId,查询指定用户的在线状态。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public QueryOnlineStatusRes queryOnlineStatus(QueryOnlineStatusBody body) throws Exception {
RawResponse rawResponse = json("QueryOnlineStatus", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, QueryOnlineStatusRes.class);
}
/**
* getBlackList
* 查询黑名单
*
* 你可以使用此接口查询指定用户的黑名单列表。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetBlackListRes getBlackList(GetBlackListBody body) throws Exception {
RawResponse rawResponse = json("GetBlackList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetBlackListRes.class);
}
/**
* isFriend
* 校验好友关系
*
* 你可以使用此接口校验两个用户之间的好友关系。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public IsFriendRes isFriend(IsFriendBody body) throws Exception {
RawResponse rawResponse = json("IsFriend", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, IsFriendRes.class);
}
/**
* isInBlackList
* 校验用户是否在黑名单
*
* 你可以使用此接口查看某用户是否在黑名单中。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public IsInBlackListRes isInBlackList(IsInBlackListBody body) throws Exception {
RawResponse rawResponse = json("IsInBlackList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, IsInBlackListRes.class);
}
/**
* addFriend
* 添加好友
*
* 你可以使用此接口添加好友。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public AddFriendRes addFriend(AddFriendBody body) throws Exception {
RawResponse rawResponse = json("AddFriend", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, AddFriendRes.class);
}
/**
* addBlackList
* 添加黑名单
*
* 你可以调用 AddBlackList 接口,指定你所属的 AppId、用户 ID 和对方的用户 ID,将对方加入黑名单。一次最多支持添加 10 个用户至黑名单。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public AddBlackListRes addBlackList(AddBlackListBody body) throws Exception {
RawResponse rawResponse = json("AddBlackList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, AddBlackListRes.class);
}
/**
* getAppToken
* 生成Token
*
* 你可以指定你所属的AppId,UserId 和 Token过期时间来获得一个对应的Token (Token本质就是使用AppId,UserId和Token过期时间字段使用AppKey进行对称性加密)
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public GetAppTokenRes getAppToken(GetAppTokenBody body) throws Exception {
RawResponse rawResponse = json("GetAppToken", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, GetAppTokenRes.class);
}
/**
* removeBlackList
* 移出黑名单
*
* 你可以使用此接口将用户移出黑名单。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public RemoveBlackListRes removeBlackList(RemoveBlackListBody body) throws Exception {
RawResponse rawResponse = json("RemoveBlackList", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, RemoveBlackListRes.class);
}
/**
* userBroadcast
* 全员广播
*
* 你可以调用 UserBroadcast 接口,指定你所属的 AppId、发送方 UserId,标签,标签匹配关系对符合标签的用户进行全员广播。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public UserBroadcastRes userBroadcast(UserBroadcastBody body) throws Exception {
RawResponse rawResponse = json("UserBroadcast", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, UserBroadcastRes.class);
}
/**
* batchUpdateUserTags
* 更新用户标签
*
* 你可以调用 BatchUpdateUserTags 接口,指定你所属的 AppId、用户 ID、用户 Tag 对已注册的广播用户进行标签更新。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchUpdateUserTagsRes batchUpdateUserTags(BatchUpdateUserTagsBody body) throws Exception {
RawResponse rawResponse = json("BatchUpdateUserTags", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchUpdateUserTagsRes.class);
}
/**
* registerUsers
* 注册用户
*
* 你可以调用 RegisterUsers 接口,指定你所属的 AppId、用户 ID , 用户 Tag 进行注册。
*
* 进行注册后,IM会存储用户资料,同时注册的用户uid列表会在全院广播的时候使用。
*
* 当前注册广播用户仅用来进行全员广播,其他操作如发消息无需注册用户。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public RegisterUsersRes registerUsers(RegisterUsersBody body) throws Exception {
RawResponse rawResponse = json("RegisterUsers", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, RegisterUsersRes.class);
}
/**
* unRegisterUsers
* 注销用户
*
* 你可以调用 UnRegisterUsers 接口,指定你所属的 AppId、用户 ID,对已注册的广播用户进行注销。
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public UnRegisterUsersRes unRegisterUsers(UnRegisterUsersBody body) throws Exception {
RawResponse rawResponse = json("UnRegisterUsers", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, UnRegisterUsersRes.class);
}
/**
* batchGetUser
* 查询用户资料
*
* 你可以调用 BatchGetUser 接口,指定你所属的 AppId、用户 ID,对已注册的广播用户进行查询。
*
*
*
*
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchGetUserRes batchGetUser(BatchGetUserBody body) throws Exception {
RawResponse rawResponse = json("BatchGetUser", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchGetUserRes.class);
}
/**
* batchUpdateUser
* 更新用户资料
*
* 你可以调用 BatchUpdateUser 接口,指定你所属的 AppId、用户 ID,对已注册的用户更新资料。
*
*
*
*
*
* @param body body payload
* @return response data
* @throws Exception error during request
*/
public BatchUpdateUserRes batchUpdateUser(BatchUpdateUserBody body) throws Exception {
RawResponse rawResponse = json("BatchUpdateUser", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchUpdateUserRes.class);
}
public BatchSendMessageRes batchSendMessage(BatchSendMessageBody body) throws Exception {
RawResponse rawResponse = json("BatchSendMessage", null, JSON.toJSONString(body));
return parseRawResponse(rawResponse, BatchSendMessageRes.class);
}
}