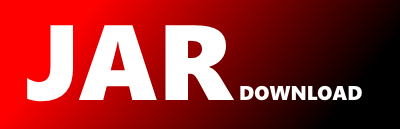
com.volcengine.service.imagex.v2.ImagexConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of volc-sdk-java Show documentation
Show all versions of volc-sdk-java Show documentation
The VOLC Engine SDK for Java
package com.volcengine.service.imagex.v2;
import com.volcengine.helper.Const;
import com.volcengine.model.ApiInfo;
import com.volcengine.model.Credentials;
import com.volcengine.model.ServiceInfo;
import org.apache.http.Header;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicHeader;
import org.apache.http.message.BasicNameValuePair;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
* Basic configuration for Imagex
*/
public class ImagexConfig {
/**
* Basic service configuration for Imagex
*/
public static Map serviceInfoMap = new HashMap() {
{
put("cn-north-1", new ServiceInfo(
new HashMap() {
{
put(Const.CONNECTION_TIMEOUT, 5000);
put(Const.SOCKET_TIMEOUT, 5000);
put(Const.Scheme, "https");
put(Const.Host, "imagex.volcengineapi.com");
put(Const.Header, new ArrayList() {
{
add(new BasicHeader("Accept", "application/json"));
}
});
put(Const.Credentials, new Credentials("cn-north-1", "ImageX"));
}
}
));
put("ap-singapore-1", new ServiceInfo(
new HashMap() {
{
put(Const.CONNECTION_TIMEOUT, 5000);
put(Const.SOCKET_TIMEOUT, 5000);
put(Const.Scheme, "https");
put(Const.Host, "imagex-ap-singapore-1.volcengineapi.com");
put(Const.Header, new ArrayList() {
{
add(new BasicHeader("Accept", "application/json"));
}
});
put(Const.Credentials, new Credentials("ap-singapore-1", "ImageX"));
}
}
));
put("us-east-1", new ServiceInfo(
new HashMap() {
{
put(Const.CONNECTION_TIMEOUT, 5000);
put(Const.SOCKET_TIMEOUT, 5000);
put(Const.Scheme, "https");
put(Const.Host, "imagex-us-east-1.volcengineapi.com");
put(Const.Header, new ArrayList() {
{
add(new BasicHeader("Accept", "application/json"));
}
});
put(Const.Credentials, new Credentials("us-east-1", "ImageX"));
}
}
));
}
};
/**
* Basic api configuration for Imagex
*/
public static Map apiInfoList = new HashMap() {
{
put("DelDomain", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DelDomain"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateRefer", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateRefer"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("UpdateHttps", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateHttps"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("UpdateResponseHeader", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateResponseHeader"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("SetDefaultDomain", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "SetDefaultDomain"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageVolcCdnAccessLog", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageVolcCdnAccessLog"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetResponseHeaderValidateKeys", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetResponseHeaderValidateKeys"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetDomainConfig", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetDomainConfig"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetServiceDomains", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetServiceDomains"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("CreateImageMigrateTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CreateImageMigrateTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DeleteImageMigrateTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DeleteImageMigrateTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("ExportFailedMigrateTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "ExportFailedMigrateTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageTaskStrategy", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageTaskStrategy"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("TerminateImageMigrateTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "TerminateImageMigrateTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetVendorBuckets", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetVendorBuckets"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageMigrateTasks", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageMigrateTasks"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("RerunImageMigrateTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "RerunImageMigrateTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSourceRequestBandwidth", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSourceRequestBandwidth"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSourceRequestTraffic", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSourceRequestTraffic"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSourceRequest", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSourceRequest"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXBucketRetrievalUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXBucketRetrievalUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSummary", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSummary"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXDomainTrafficData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXDomainTrafficData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXDomainBandwidthData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXDomainBandwidthData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXDomainBandwidthNinetyFiveData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXDomainBandwidthNinetyFiveData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXBucketUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXBucketUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXBillingRequestCntUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXBillingRequestCntUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXRequestCntUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXRequestCntUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXBaseOpUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXBaseOpUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCompressUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCompressUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXScreenshotUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXScreenshotUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXVideoClipDurationUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXVideoClipDurationUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXMultiCompressUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXMultiCompressUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXEdgeRequest", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXEdgeRequest"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXEdgeRequestBandwidth", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXEdgeRequestBandwidth"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXEdgeRequestTraffic", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXEdgeRequestTraffic"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXEdgeRequestRegions", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXEdgeRequestRegions"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXMirrorRequestHttpCodeByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXMirrorRequestHttpCodeByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXMirrorRequestHttpCodeOverview", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXMirrorRequestHttpCodeOverview"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXMirrorRequestTraffic", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXMirrorRequestTraffic"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXMirrorRequestBandwidth", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXMirrorRequestBandwidth"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXServerQPSUsage", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXServerQPSUsage"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXHitRateTrafficData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXHitRateTrafficData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXHitRateRequestData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXHitRateRequestData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCDNTopRequestData", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCDNTopRequestData"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXExceedResolutionRatioAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXExceedResolutionRatioAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXExceedFileSize", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXExceedFileSize"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXExceedCountByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXExceedCountByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXServiceQuality", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXServiceQuality"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageXQueryApps", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageXQueryApps"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageXQueryRegions", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageXQueryRegions"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageXQueryDims", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageXQueryDims"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageXQueryVals", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageXQueryVals"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadCountByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadCountByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadDuration", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadDuration"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadSuccessRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadSuccessRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadFileSize", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadFileSize"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadErrorCodeByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadErrorCodeByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadErrorCodeAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadErrorCodeAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadSpeed", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadSpeed"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXUploadSegmentSpeedByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXUploadSegmentSpeedByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnSuccessRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnSuccessRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnSuccessRateAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnSuccessRateAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnErrorCodeByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnErrorCodeByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnErrorCodeAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnErrorCodeAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnDurationDetailByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnDurationDetailByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnDurationAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnDurationAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnReuseRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnReuseRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnReuseRateAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnReuseRateAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXCdnProtocolRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXCdnProtocolRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientFailureRate", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientFailureRate"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientDecodeSuccessRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientDecodeSuccessRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientDecodeDurationByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientDecodeDurationByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientQueueDurationByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientQueueDurationByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientErrorCodeByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientErrorCodeByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientErrorCodeAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientErrorCodeAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientLoadDuration", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientLoadDuration"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientLoadDurationAll", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientLoadDurationAll"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientSdkVerByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientSdkVerByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientFileSize", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientFileSize"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientTopFileSize", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientTopFileSize"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientCountByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientCountByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientQualityRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientQualityRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientTopQualityURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientTopQualityURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientDemotionRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientDemotionRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientTopDemotionURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientTopDemotionURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXClientScoreByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXClientScoreByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleCountByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleCountByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleCacheHitRateByTime", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleCacheHitRateByTime"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleTopSizeURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleTopSizeURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleTopResolutionURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleTopResolutionURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleTopRamURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleTopRamURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DescribeImageXSensibleTopUnknownURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DescribeImageXSensibleTopUnknownURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageResourceStatus", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageResourceStatus"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageStorageFiles", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageStorageFiles"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("DeleteImageUploadFiles", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DeleteImageUploadFiles"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("UpdateImageUploadFiles", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageUploadFiles"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("CommitImageUpload", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CommitImageUpload"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("ApplyImageUpload", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "ApplyImageUpload"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetImageUploadFile", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageUploadFile"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageUploadFiles", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageUploadFiles"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetImageUpdateFiles", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageUpdateFiles"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("PreviewImageUploadFile", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "PreviewImageUploadFile"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageServiceSubscription", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageServiceSubscription"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageService", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageService"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetAllImageServices", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetAllImageServices"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("CreateImageCompressTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CreateImageCompressTask"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("FetchImageUrl", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "FetchImageUrl"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageStorageTTL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageStorageTTL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetCompressTaskInfo", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetCompressTaskInfo"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetUrlFetchTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetUrlFetchTask"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("GetResourceURL", new ApiInfo(
new HashMap() {
{
put(Const.Method, "GET");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetResourceURL"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("CreateImageFromUri", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CreateImageFromUri"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageFileKey", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageFileKey"));
add(new BasicNameValuePair("Version", "2018-08-01"));
}
});
}
}
));
put("CreateImageContentTask", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CreateImageContentTask"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageContentTaskDetail", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageContentTaskDetail"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("GetImageContentBlockList", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "GetImageContentBlockList"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("CreateImageTranscodeQueue", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "CreateImageTranscodeQueue"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("DeleteImageTranscodeQueue", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "DeleteImageTranscodeQueue"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageTranscodeQueue", new ApiInfo(
new HashMap() {
{
put(Const.Method, "POST");
put(Const.Path, "/");
put(Const.Query, new ArrayList() {
{
add(new BasicNameValuePair("Action", "UpdateImageTranscodeQueue"));
add(new BasicNameValuePair("Version", "2023-05-01"));
}
});
}
}
));
put("UpdateImageTranscodeQueueStatus", new ApiInfo(
new HashMap