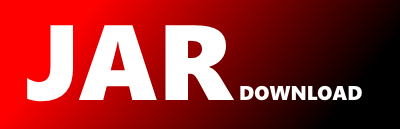
com.volcengine.service.vikingDB.Collection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of volc-sdk-java Show documentation
Show all versions of volc-sdk-java Show documentation
The VOLC Engine SDK for Java
package com.volcengine.service.vikingDB;
import com.google.gson.internal.LinkedTreeMap;
import com.volcengine.service.vikingDB.common.DataObject;
import com.volcengine.service.vikingDB.common.Field;
import com.volcengine.service.vikingDB.common.ID;
import lombok.Data;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Data
public class Collection {
private String collectionName;
private List fields;
private VikingDBService vikingDBService;
private String primaryKey;
private List indexes = null;
private String description="";
private HashMap stat=null;
private String createTime=null;
private String updateTime=null;
private String updatePerson=null;
public Collection(String collectionName, List fields, VikingDBService vikingDBService, String primaryKey){
this.collectionName = collectionName;
this.fields = fields;
this.vikingDBService = vikingDBService;
this.primaryKey = primaryKey;
}
public Collection(){}
public void upsertData(DataObject dataObject) throws Exception{
if(dataObject.getIsBuild() == 0){
VikingDBException vikingDBException = new VikingDBException(1000031, null, "Param dose not build");
throw vikingDBException.getErrorCodeException(1000031, null, "Param dose not build");
}
List> fieldList = new ArrayList<>();
fieldList.add(dataObject.getFields());
Integer ttl = null;
if(dataObject.getTTL() != null){
ttl = dataObject.getTTL();
}
HashMap params = new HashMap();
params.put("collection_name", collectionName);
params.put("fields", fieldList);
params.put("ttl", ttl);
// System.out.println(params);
vikingDBService.doRequest("UpsertData", null, params);
}
public void upsertData(List dataObjects) throws Exception{
List> fieldList = new ArrayList<>();
HashMap> record = new HashMap<>();
for(DataObject item: dataObjects){
if(item.getIsBuild() == 0){
VikingDBException vikingDBException = new VikingDBException(1000031, null, "Param dose not build");
throw vikingDBException.getErrorCodeException(1000031, null, "Param dose not build");
}
if(record.get(item.getTTL()) != null){
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy