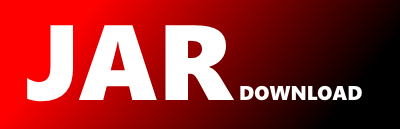
com.volcengine.service.vikingDB.Index Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of volc-sdk-java Show documentation
Show all versions of volc-sdk-java Show documentation
The VOLC Engine SDK for Java
package com.volcengine.service.vikingDB;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.hamcrest.core.IsInstanceOf;
import com.google.gson.internal.LinkedTreeMap;
import com.volcengine.model.stream.SearchAuthorResponse;
import com.volcengine.service.vikingDB.common.DataObject;
import com.volcengine.service.vikingDB.common.FetchDataParam;
import com.volcengine.service.vikingDB.common.SearchByIdParam;
import com.volcengine.service.vikingDB.common.SearchByTextParam;
import com.volcengine.service.vikingDB.common.SearchByVectorParam;
import com.volcengine.service.vikingDB.common.SearchParam;
import com.volcengine.service.vikingDB.common.VectorIndexParams;
import lombok.Data;
@Data
public class Index {
private String collectionName = null;
private String indexName = null;
private String description = "";
private VectorIndexParams vectorIndex = null;
private List scalarIndex = null;
private String stat = null;
private VikingDBService vikingDBService = null;
private Integer cpuQuota = 2;
private Object partitionBy = null;
private String primaryKey = null;
private String createTime=null;
private String updateTime=null;
private String updatePerson=null;
private Integer shardCount=null;
private HashMap indexCost=null;
public Index(){}
public Index(String collectionName, String indexName, VectorIndexParams vectorIndex,
List scalarIndex, String stat, VikingDBService vikingDBService){
this.collectionName = collectionName;
this.indexName = indexName;
this.vectorIndex = vectorIndex;
this.scalarIndex = scalarIndex;
this.stat = stat;
this.vikingDBService = vikingDBService;
}
public void setCpuQuota(Integer cpuQuota) {
this.cpuQuota = cpuQuota;
}
public void setCpuQuota(Long cpuQuota) {
this.cpuQuota = cpuQuota.intValue();
}
public void setShardCount(Integer shardCount) {
this.shardCount = shardCount;
}
public void setShardCount(Long shardCount) {
this.shardCount = shardCount.intValue();
}
public String requestPrimaryKey() throws Exception{
if(this.primaryKey != null) return this.primaryKey;
Collection collection = vikingDBService.getCollection(this.collectionName);
this.primaryKey = collection.getPrimaryKey();
return this.primaryKey;
}
public List search(SearchParam searchParam) throws Exception{
if(searchParam.getIsBuild() == 0){
VikingDBException vikingDBException = new VikingDBException(1000031, null, "Param dose not build");
throw vikingDBException.getErrorCodeException(1000031, null, "Param dose not build");
}
List res = new ArrayList<>();
if(searchParam.getVectorOrder() != null){
if(searchParam.getVectorOrder().getVector() != null){
SearchByVectorParam searchByVectorParam = new SearchByVectorParam()
.setFilter(searchParam.getFilter())
.setLimit(searchParam.getLimit())
.setOutputFields(searchParam.getOutputFields())
.setPartition(searchParam.getPartition())
.setVector(searchParam.getVectorOrder().getVector())
.setDenseWeight(searchParam.getDenseWeight())
.setSparseVectors(searchParam.getVectorOrder().getSparseVectors())
.build();
return searchByVector(searchByVectorParam);
} else if(searchParam.getVectorOrder().getId() != null){
SearchByIdParam searchByIdParam = new SearchByIdParam()
.setFilter(searchParam.getFilter())
.setLimit(searchParam.getLimit())
.setOutputFields(searchParam.getOutputFields())
.setPartition(searchParam.getPartition())
.setId(searchParam.getVectorOrder().getId())
.setDenseWeight(searchParam.getDenseWeight())
.build();
res = searchById(searchByIdParam);
}
} else if(searchParam.getScalarOrder() != null){
HashMap orderByScalar = new HashMap<>();
orderByScalar.put("order", searchParam.getScalarOrder().getOrder());
orderByScalar.put("field_name", searchParam.getScalarOrder().getFieldName());
HashMap search = new HashMap<>();
search.put("order_by_scalar", orderByScalar);
search.put("limit", searchParam.getLimit());
search.put("partition", searchParam.getPartition());
if(searchParam.getOutputFields() != null)
search.put("output_fields", searchParam.getOutputFields());
if(searchParam.getFilter() != null)
search.put("filter", searchParam.getFilter());
HashMap params = new HashMap<>();
params.put("collection_name", collectionName);
params.put("index_name", indexName);
params.put("search", search);
LinkedTreeMap resData = vikingDBService.doRequest("SearchIndex",null, params);
res = getDatas(resData, searchParam.getOutputFields());
} else {
HashMap search = new HashMap<>();
search.put("limit", searchParam.getLimit());
search.put("partition", searchParam.getPartition());
if(searchParam.getOutputFields() != null)
search.put("output_fields", searchParam.getOutputFields());
if(searchParam.getFilter() != null)
search.put("filter", searchParam.getFilter());
HashMap params = new HashMap<>();
params.put("collection_name", collectionName);
params.put("index_name", indexName);
params.put("search", search);
LinkedTreeMap resData = vikingDBService.doRequest("SearchIndex",null, params);
res = getDatas(resData, searchParam.getOutputFields());
}
return res;
}
public List searchById(SearchByIdParam searchByIdParam) throws Exception{
if(searchByIdParam.getIsBuild() == 0){
VikingDBException vikingDBException = new VikingDBException(1000031, null, "Param dose not build");
throw vikingDBException.getErrorCodeException(1000031, null, "Param dose not build");
}
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy