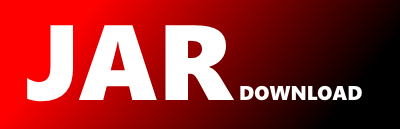
com.volcengine.billing.model.ListForListBillOverviewByProdOutput Maven / Gradle / Ivy
/*
* billing
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.billing.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* ListForListBillOverviewByProdOutput
*/
public class ListForListBillOverviewByProdOutput {
@SerializedName("BillCategoryParent")
private String billCategoryParent = null;
@SerializedName("BillPeriod")
private String billPeriod = null;
@SerializedName("BillingMode")
private String billingMode = null;
@SerializedName("BusinessMode")
private String businessMode = null;
@SerializedName("CouponAmount")
private String couponAmount = null;
@SerializedName("CreditCarriedAmount")
private String creditCarriedAmount = null;
@SerializedName("DiscountBillAmount")
private String discountBillAmount = null;
@SerializedName("OriginalBillAmount")
private String originalBillAmount = null;
@SerializedName("OwnerCustomerName")
private String ownerCustomerName = null;
@SerializedName("OwnerID")
private String ownerID = null;
@SerializedName("OwnerUserName")
private String ownerUserName = null;
@SerializedName("PaidAmount")
private String paidAmount = null;
@SerializedName("PayableAmount")
private String payableAmount = null;
@SerializedName("PayerCustomerName")
private String payerCustomerName = null;
@SerializedName("PayerID")
private String payerID = null;
@SerializedName("PayerUserName")
private String payerUserName = null;
@SerializedName("PreferentialBillAmount")
private String preferentialBillAmount = null;
@SerializedName("Product")
private String product = null;
@SerializedName("ProductZh")
private String productZh = null;
@SerializedName("RoundBillAmount")
private String roundBillAmount = null;
@SerializedName("SellerCustomerName")
private String sellerCustomerName = null;
@SerializedName("SellerID")
private String sellerID = null;
@SerializedName("SellerUserName")
private String sellerUserName = null;
@SerializedName("SettlementType")
private String settlementType = null;
@SerializedName("UnpaidAmount")
private String unpaidAmount = null;
public ListForListBillOverviewByProdOutput billCategoryParent(String billCategoryParent) {
this.billCategoryParent = billCategoryParent;
return this;
}
/**
* Get billCategoryParent
* @return billCategoryParent
**/
@Schema(description = "")
public String getBillCategoryParent() {
return billCategoryParent;
}
public void setBillCategoryParent(String billCategoryParent) {
this.billCategoryParent = billCategoryParent;
}
public ListForListBillOverviewByProdOutput billPeriod(String billPeriod) {
this.billPeriod = billPeriod;
return this;
}
/**
* Get billPeriod
* @return billPeriod
**/
@Schema(description = "")
public String getBillPeriod() {
return billPeriod;
}
public void setBillPeriod(String billPeriod) {
this.billPeriod = billPeriod;
}
public ListForListBillOverviewByProdOutput billingMode(String billingMode) {
this.billingMode = billingMode;
return this;
}
/**
* Get billingMode
* @return billingMode
**/
@Schema(description = "")
public String getBillingMode() {
return billingMode;
}
public void setBillingMode(String billingMode) {
this.billingMode = billingMode;
}
public ListForListBillOverviewByProdOutput businessMode(String businessMode) {
this.businessMode = businessMode;
return this;
}
/**
* Get businessMode
* @return businessMode
**/
@Schema(description = "")
public String getBusinessMode() {
return businessMode;
}
public void setBusinessMode(String businessMode) {
this.businessMode = businessMode;
}
public ListForListBillOverviewByProdOutput couponAmount(String couponAmount) {
this.couponAmount = couponAmount;
return this;
}
/**
* Get couponAmount
* @return couponAmount
**/
@Schema(description = "")
public String getCouponAmount() {
return couponAmount;
}
public void setCouponAmount(String couponAmount) {
this.couponAmount = couponAmount;
}
public ListForListBillOverviewByProdOutput creditCarriedAmount(String creditCarriedAmount) {
this.creditCarriedAmount = creditCarriedAmount;
return this;
}
/**
* Get creditCarriedAmount
* @return creditCarriedAmount
**/
@Schema(description = "")
public String getCreditCarriedAmount() {
return creditCarriedAmount;
}
public void setCreditCarriedAmount(String creditCarriedAmount) {
this.creditCarriedAmount = creditCarriedAmount;
}
public ListForListBillOverviewByProdOutput discountBillAmount(String discountBillAmount) {
this.discountBillAmount = discountBillAmount;
return this;
}
/**
* Get discountBillAmount
* @return discountBillAmount
**/
@Schema(description = "")
public String getDiscountBillAmount() {
return discountBillAmount;
}
public void setDiscountBillAmount(String discountBillAmount) {
this.discountBillAmount = discountBillAmount;
}
public ListForListBillOverviewByProdOutput originalBillAmount(String originalBillAmount) {
this.originalBillAmount = originalBillAmount;
return this;
}
/**
* Get originalBillAmount
* @return originalBillAmount
**/
@Schema(description = "")
public String getOriginalBillAmount() {
return originalBillAmount;
}
public void setOriginalBillAmount(String originalBillAmount) {
this.originalBillAmount = originalBillAmount;
}
public ListForListBillOverviewByProdOutput ownerCustomerName(String ownerCustomerName) {
this.ownerCustomerName = ownerCustomerName;
return this;
}
/**
* Get ownerCustomerName
* @return ownerCustomerName
**/
@Schema(description = "")
public String getOwnerCustomerName() {
return ownerCustomerName;
}
public void setOwnerCustomerName(String ownerCustomerName) {
this.ownerCustomerName = ownerCustomerName;
}
public ListForListBillOverviewByProdOutput ownerID(String ownerID) {
this.ownerID = ownerID;
return this;
}
/**
* Get ownerID
* @return ownerID
**/
@Schema(description = "")
public String getOwnerID() {
return ownerID;
}
public void setOwnerID(String ownerID) {
this.ownerID = ownerID;
}
public ListForListBillOverviewByProdOutput ownerUserName(String ownerUserName) {
this.ownerUserName = ownerUserName;
return this;
}
/**
* Get ownerUserName
* @return ownerUserName
**/
@Schema(description = "")
public String getOwnerUserName() {
return ownerUserName;
}
public void setOwnerUserName(String ownerUserName) {
this.ownerUserName = ownerUserName;
}
public ListForListBillOverviewByProdOutput paidAmount(String paidAmount) {
this.paidAmount = paidAmount;
return this;
}
/**
* Get paidAmount
* @return paidAmount
**/
@Schema(description = "")
public String getPaidAmount() {
return paidAmount;
}
public void setPaidAmount(String paidAmount) {
this.paidAmount = paidAmount;
}
public ListForListBillOverviewByProdOutput payableAmount(String payableAmount) {
this.payableAmount = payableAmount;
return this;
}
/**
* Get payableAmount
* @return payableAmount
**/
@Schema(description = "")
public String getPayableAmount() {
return payableAmount;
}
public void setPayableAmount(String payableAmount) {
this.payableAmount = payableAmount;
}
public ListForListBillOverviewByProdOutput payerCustomerName(String payerCustomerName) {
this.payerCustomerName = payerCustomerName;
return this;
}
/**
* Get payerCustomerName
* @return payerCustomerName
**/
@Schema(description = "")
public String getPayerCustomerName() {
return payerCustomerName;
}
public void setPayerCustomerName(String payerCustomerName) {
this.payerCustomerName = payerCustomerName;
}
public ListForListBillOverviewByProdOutput payerID(String payerID) {
this.payerID = payerID;
return this;
}
/**
* Get payerID
* @return payerID
**/
@Schema(description = "")
public String getPayerID() {
return payerID;
}
public void setPayerID(String payerID) {
this.payerID = payerID;
}
public ListForListBillOverviewByProdOutput payerUserName(String payerUserName) {
this.payerUserName = payerUserName;
return this;
}
/**
* Get payerUserName
* @return payerUserName
**/
@Schema(description = "")
public String getPayerUserName() {
return payerUserName;
}
public void setPayerUserName(String payerUserName) {
this.payerUserName = payerUserName;
}
public ListForListBillOverviewByProdOutput preferentialBillAmount(String preferentialBillAmount) {
this.preferentialBillAmount = preferentialBillAmount;
return this;
}
/**
* Get preferentialBillAmount
* @return preferentialBillAmount
**/
@Schema(description = "")
public String getPreferentialBillAmount() {
return preferentialBillAmount;
}
public void setPreferentialBillAmount(String preferentialBillAmount) {
this.preferentialBillAmount = preferentialBillAmount;
}
public ListForListBillOverviewByProdOutput product(String product) {
this.product = product;
return this;
}
/**
* Get product
* @return product
**/
@Schema(description = "")
public String getProduct() {
return product;
}
public void setProduct(String product) {
this.product = product;
}
public ListForListBillOverviewByProdOutput productZh(String productZh) {
this.productZh = productZh;
return this;
}
/**
* Get productZh
* @return productZh
**/
@Schema(description = "")
public String getProductZh() {
return productZh;
}
public void setProductZh(String productZh) {
this.productZh = productZh;
}
public ListForListBillOverviewByProdOutput roundBillAmount(String roundBillAmount) {
this.roundBillAmount = roundBillAmount;
return this;
}
/**
* Get roundBillAmount
* @return roundBillAmount
**/
@Schema(description = "")
public String getRoundBillAmount() {
return roundBillAmount;
}
public void setRoundBillAmount(String roundBillAmount) {
this.roundBillAmount = roundBillAmount;
}
public ListForListBillOverviewByProdOutput sellerCustomerName(String sellerCustomerName) {
this.sellerCustomerName = sellerCustomerName;
return this;
}
/**
* Get sellerCustomerName
* @return sellerCustomerName
**/
@Schema(description = "")
public String getSellerCustomerName() {
return sellerCustomerName;
}
public void setSellerCustomerName(String sellerCustomerName) {
this.sellerCustomerName = sellerCustomerName;
}
public ListForListBillOverviewByProdOutput sellerID(String sellerID) {
this.sellerID = sellerID;
return this;
}
/**
* Get sellerID
* @return sellerID
**/
@Schema(description = "")
public String getSellerID() {
return sellerID;
}
public void setSellerID(String sellerID) {
this.sellerID = sellerID;
}
public ListForListBillOverviewByProdOutput sellerUserName(String sellerUserName) {
this.sellerUserName = sellerUserName;
return this;
}
/**
* Get sellerUserName
* @return sellerUserName
**/
@Schema(description = "")
public String getSellerUserName() {
return sellerUserName;
}
public void setSellerUserName(String sellerUserName) {
this.sellerUserName = sellerUserName;
}
public ListForListBillOverviewByProdOutput settlementType(String settlementType) {
this.settlementType = settlementType;
return this;
}
/**
* Get settlementType
* @return settlementType
**/
@Schema(description = "")
public String getSettlementType() {
return settlementType;
}
public void setSettlementType(String settlementType) {
this.settlementType = settlementType;
}
public ListForListBillOverviewByProdOutput unpaidAmount(String unpaidAmount) {
this.unpaidAmount = unpaidAmount;
return this;
}
/**
* Get unpaidAmount
* @return unpaidAmount
**/
@Schema(description = "")
public String getUnpaidAmount() {
return unpaidAmount;
}
public void setUnpaidAmount(String unpaidAmount) {
this.unpaidAmount = unpaidAmount;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ListForListBillOverviewByProdOutput listForListBillOverviewByProdOutput = (ListForListBillOverviewByProdOutput) o;
return Objects.equals(this.billCategoryParent, listForListBillOverviewByProdOutput.billCategoryParent) &&
Objects.equals(this.billPeriod, listForListBillOverviewByProdOutput.billPeriod) &&
Objects.equals(this.billingMode, listForListBillOverviewByProdOutput.billingMode) &&
Objects.equals(this.businessMode, listForListBillOverviewByProdOutput.businessMode) &&
Objects.equals(this.couponAmount, listForListBillOverviewByProdOutput.couponAmount) &&
Objects.equals(this.creditCarriedAmount, listForListBillOverviewByProdOutput.creditCarriedAmount) &&
Objects.equals(this.discountBillAmount, listForListBillOverviewByProdOutput.discountBillAmount) &&
Objects.equals(this.originalBillAmount, listForListBillOverviewByProdOutput.originalBillAmount) &&
Objects.equals(this.ownerCustomerName, listForListBillOverviewByProdOutput.ownerCustomerName) &&
Objects.equals(this.ownerID, listForListBillOverviewByProdOutput.ownerID) &&
Objects.equals(this.ownerUserName, listForListBillOverviewByProdOutput.ownerUserName) &&
Objects.equals(this.paidAmount, listForListBillOverviewByProdOutput.paidAmount) &&
Objects.equals(this.payableAmount, listForListBillOverviewByProdOutput.payableAmount) &&
Objects.equals(this.payerCustomerName, listForListBillOverviewByProdOutput.payerCustomerName) &&
Objects.equals(this.payerID, listForListBillOverviewByProdOutput.payerID) &&
Objects.equals(this.payerUserName, listForListBillOverviewByProdOutput.payerUserName) &&
Objects.equals(this.preferentialBillAmount, listForListBillOverviewByProdOutput.preferentialBillAmount) &&
Objects.equals(this.product, listForListBillOverviewByProdOutput.product) &&
Objects.equals(this.productZh, listForListBillOverviewByProdOutput.productZh) &&
Objects.equals(this.roundBillAmount, listForListBillOverviewByProdOutput.roundBillAmount) &&
Objects.equals(this.sellerCustomerName, listForListBillOverviewByProdOutput.sellerCustomerName) &&
Objects.equals(this.sellerID, listForListBillOverviewByProdOutput.sellerID) &&
Objects.equals(this.sellerUserName, listForListBillOverviewByProdOutput.sellerUserName) &&
Objects.equals(this.settlementType, listForListBillOverviewByProdOutput.settlementType) &&
Objects.equals(this.unpaidAmount, listForListBillOverviewByProdOutput.unpaidAmount);
}
@Override
public int hashCode() {
return Objects.hash(billCategoryParent, billPeriod, billingMode, businessMode, couponAmount, creditCarriedAmount, discountBillAmount, originalBillAmount, ownerCustomerName, ownerID, ownerUserName, paidAmount, payableAmount, payerCustomerName, payerID, payerUserName, preferentialBillAmount, product, productZh, roundBillAmount, sellerCustomerName, sellerID, sellerUserName, settlementType, unpaidAmount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ListForListBillOverviewByProdOutput {\n");
sb.append(" billCategoryParent: ").append(toIndentedString(billCategoryParent)).append("\n");
sb.append(" billPeriod: ").append(toIndentedString(billPeriod)).append("\n");
sb.append(" billingMode: ").append(toIndentedString(billingMode)).append("\n");
sb.append(" businessMode: ").append(toIndentedString(businessMode)).append("\n");
sb.append(" couponAmount: ").append(toIndentedString(couponAmount)).append("\n");
sb.append(" creditCarriedAmount: ").append(toIndentedString(creditCarriedAmount)).append("\n");
sb.append(" discountBillAmount: ").append(toIndentedString(discountBillAmount)).append("\n");
sb.append(" originalBillAmount: ").append(toIndentedString(originalBillAmount)).append("\n");
sb.append(" ownerCustomerName: ").append(toIndentedString(ownerCustomerName)).append("\n");
sb.append(" ownerID: ").append(toIndentedString(ownerID)).append("\n");
sb.append(" ownerUserName: ").append(toIndentedString(ownerUserName)).append("\n");
sb.append(" paidAmount: ").append(toIndentedString(paidAmount)).append("\n");
sb.append(" payableAmount: ").append(toIndentedString(payableAmount)).append("\n");
sb.append(" payerCustomerName: ").append(toIndentedString(payerCustomerName)).append("\n");
sb.append(" payerID: ").append(toIndentedString(payerID)).append("\n");
sb.append(" payerUserName: ").append(toIndentedString(payerUserName)).append("\n");
sb.append(" preferentialBillAmount: ").append(toIndentedString(preferentialBillAmount)).append("\n");
sb.append(" product: ").append(toIndentedString(product)).append("\n");
sb.append(" productZh: ").append(toIndentedString(productZh)).append("\n");
sb.append(" roundBillAmount: ").append(toIndentedString(roundBillAmount)).append("\n");
sb.append(" sellerCustomerName: ").append(toIndentedString(sellerCustomerName)).append("\n");
sb.append(" sellerID: ").append(toIndentedString(sellerID)).append("\n");
sb.append(" sellerUserName: ").append(toIndentedString(sellerUserName)).append("\n");
sb.append(" settlementType: ").append(toIndentedString(settlementType)).append("\n");
sb.append(" unpaidAmount: ").append(toIndentedString(unpaidAmount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy