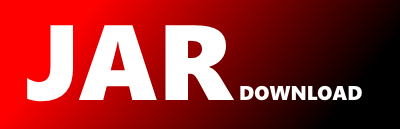
com.volcengine.cdn.model.ServiceInfoForDescribeCdnServiceOutput Maven / Gradle / Ivy
/*
* cdn
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.cdn.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* ServiceInfoForDescribeCdnServiceOutput
*/
public class ServiceInfoForDescribeCdnServiceOutput {
@SerializedName("BeginTime")
private String beginTime = null;
@SerializedName("BillingCode")
private String billingCode = null;
@SerializedName("BillingCycle")
private String billingCycle = null;
@SerializedName("BillingData")
private String billingData = null;
@SerializedName("BillingDesc")
private String billingDesc = null;
@SerializedName("CreateTime")
private String createTime = null;
@SerializedName("InstanceCategory")
private String instanceCategory = null;
@SerializedName("InstanceType")
private String instanceType = null;
@SerializedName("MetricType")
private String metricType = null;
@SerializedName("ServiceRegion")
private String serviceRegion = null;
@SerializedName("StartTime")
private String startTime = null;
@SerializedName("Status")
private String status = null;
public ServiceInfoForDescribeCdnServiceOutput beginTime(String beginTime) {
this.beginTime = beginTime;
return this;
}
/**
* Get beginTime
* @return beginTime
**/
@Schema(description = "")
public String getBeginTime() {
return beginTime;
}
public void setBeginTime(String beginTime) {
this.beginTime = beginTime;
}
public ServiceInfoForDescribeCdnServiceOutput billingCode(String billingCode) {
this.billingCode = billingCode;
return this;
}
/**
* Get billingCode
* @return billingCode
**/
@Schema(description = "")
public String getBillingCode() {
return billingCode;
}
public void setBillingCode(String billingCode) {
this.billingCode = billingCode;
}
public ServiceInfoForDescribeCdnServiceOutput billingCycle(String billingCycle) {
this.billingCycle = billingCycle;
return this;
}
/**
* Get billingCycle
* @return billingCycle
**/
@Schema(description = "")
public String getBillingCycle() {
return billingCycle;
}
public void setBillingCycle(String billingCycle) {
this.billingCycle = billingCycle;
}
public ServiceInfoForDescribeCdnServiceOutput billingData(String billingData) {
this.billingData = billingData;
return this;
}
/**
* Get billingData
* @return billingData
**/
@Schema(description = "")
public String getBillingData() {
return billingData;
}
public void setBillingData(String billingData) {
this.billingData = billingData;
}
public ServiceInfoForDescribeCdnServiceOutput billingDesc(String billingDesc) {
this.billingDesc = billingDesc;
return this;
}
/**
* Get billingDesc
* @return billingDesc
**/
@Schema(description = "")
public String getBillingDesc() {
return billingDesc;
}
public void setBillingDesc(String billingDesc) {
this.billingDesc = billingDesc;
}
public ServiceInfoForDescribeCdnServiceOutput createTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Get createTime
* @return createTime
**/
@Schema(description = "")
public String getCreateTime() {
return createTime;
}
public void setCreateTime(String createTime) {
this.createTime = createTime;
}
public ServiceInfoForDescribeCdnServiceOutput instanceCategory(String instanceCategory) {
this.instanceCategory = instanceCategory;
return this;
}
/**
* Get instanceCategory
* @return instanceCategory
**/
@Schema(description = "")
public String getInstanceCategory() {
return instanceCategory;
}
public void setInstanceCategory(String instanceCategory) {
this.instanceCategory = instanceCategory;
}
public ServiceInfoForDescribeCdnServiceOutput instanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* Get instanceType
* @return instanceType
**/
@Schema(description = "")
public String getInstanceType() {
return instanceType;
}
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
public ServiceInfoForDescribeCdnServiceOutput metricType(String metricType) {
this.metricType = metricType;
return this;
}
/**
* Get metricType
* @return metricType
**/
@Schema(description = "")
public String getMetricType() {
return metricType;
}
public void setMetricType(String metricType) {
this.metricType = metricType;
}
public ServiceInfoForDescribeCdnServiceOutput serviceRegion(String serviceRegion) {
this.serviceRegion = serviceRegion;
return this;
}
/**
* Get serviceRegion
* @return serviceRegion
**/
@Schema(description = "")
public String getServiceRegion() {
return serviceRegion;
}
public void setServiceRegion(String serviceRegion) {
this.serviceRegion = serviceRegion;
}
public ServiceInfoForDescribeCdnServiceOutput startTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Get startTime
* @return startTime
**/
@Schema(description = "")
public String getStartTime() {
return startTime;
}
public void setStartTime(String startTime) {
this.startTime = startTime;
}
public ServiceInfoForDescribeCdnServiceOutput status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@Schema(description = "")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ServiceInfoForDescribeCdnServiceOutput serviceInfoForDescribeCdnServiceOutput = (ServiceInfoForDescribeCdnServiceOutput) o;
return Objects.equals(this.beginTime, serviceInfoForDescribeCdnServiceOutput.beginTime) &&
Objects.equals(this.billingCode, serviceInfoForDescribeCdnServiceOutput.billingCode) &&
Objects.equals(this.billingCycle, serviceInfoForDescribeCdnServiceOutput.billingCycle) &&
Objects.equals(this.billingData, serviceInfoForDescribeCdnServiceOutput.billingData) &&
Objects.equals(this.billingDesc, serviceInfoForDescribeCdnServiceOutput.billingDesc) &&
Objects.equals(this.createTime, serviceInfoForDescribeCdnServiceOutput.createTime) &&
Objects.equals(this.instanceCategory, serviceInfoForDescribeCdnServiceOutput.instanceCategory) &&
Objects.equals(this.instanceType, serviceInfoForDescribeCdnServiceOutput.instanceType) &&
Objects.equals(this.metricType, serviceInfoForDescribeCdnServiceOutput.metricType) &&
Objects.equals(this.serviceRegion, serviceInfoForDescribeCdnServiceOutput.serviceRegion) &&
Objects.equals(this.startTime, serviceInfoForDescribeCdnServiceOutput.startTime) &&
Objects.equals(this.status, serviceInfoForDescribeCdnServiceOutput.status);
}
@Override
public int hashCode() {
return Objects.hash(beginTime, billingCode, billingCycle, billingData, billingDesc, createTime, instanceCategory, instanceType, metricType, serviceRegion, startTime, status);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ServiceInfoForDescribeCdnServiceOutput {\n");
sb.append(" beginTime: ").append(toIndentedString(beginTime)).append("\n");
sb.append(" billingCode: ").append(toIndentedString(billingCode)).append("\n");
sb.append(" billingCycle: ").append(toIndentedString(billingCycle)).append("\n");
sb.append(" billingData: ").append(toIndentedString(billingData)).append("\n");
sb.append(" billingDesc: ").append(toIndentedString(billingDesc)).append("\n");
sb.append(" createTime: ").append(toIndentedString(createTime)).append("\n");
sb.append(" instanceCategory: ").append(toIndentedString(instanceCategory)).append("\n");
sb.append(" instanceType: ").append(toIndentedString(instanceType)).append("\n");
sb.append(" metricType: ").append(toIndentedString(metricType)).append("\n");
sb.append(" serviceRegion: ").append(toIndentedString(serviceRegion)).append("\n");
sb.append(" startTime: ").append(toIndentedString(startTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy