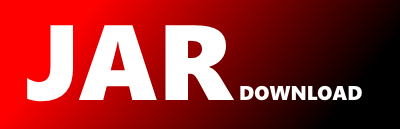
com.volcengine.cdn.model.AccessActionForUpdateCdnConfigInput Maven / Gradle / Ivy
/*
* cdn
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.cdn.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* AccessActionForUpdateCdnConfigInput
*/
public class AccessActionForUpdateCdnConfigInput {
@SerializedName("AllowEmpty")
private Boolean allowEmpty = null;
@SerializedName("ListRules")
private List listRules = null;
@SerializedName("RequestHeader")
private String requestHeader = null;
@SerializedName("RuleType")
private String ruleType = null;
public AccessActionForUpdateCdnConfigInput allowEmpty(Boolean allowEmpty) {
this.allowEmpty = allowEmpty;
return this;
}
/**
* Get allowEmpty
* @return allowEmpty
**/
@Schema(description = "")
public Boolean isAllowEmpty() {
return allowEmpty;
}
public void setAllowEmpty(Boolean allowEmpty) {
this.allowEmpty = allowEmpty;
}
public AccessActionForUpdateCdnConfigInput listRules(List listRules) {
this.listRules = listRules;
return this;
}
public AccessActionForUpdateCdnConfigInput addListRulesItem(String listRulesItem) {
if (this.listRules == null) {
this.listRules = new ArrayList();
}
this.listRules.add(listRulesItem);
return this;
}
/**
* Get listRules
* @return listRules
**/
@Schema(description = "")
public List getListRules() {
return listRules;
}
public void setListRules(List listRules) {
this.listRules = listRules;
}
public AccessActionForUpdateCdnConfigInput requestHeader(String requestHeader) {
this.requestHeader = requestHeader;
return this;
}
/**
* Get requestHeader
* @return requestHeader
**/
@Schema(description = "")
public String getRequestHeader() {
return requestHeader;
}
public void setRequestHeader(String requestHeader) {
this.requestHeader = requestHeader;
}
public AccessActionForUpdateCdnConfigInput ruleType(String ruleType) {
this.ruleType = ruleType;
return this;
}
/**
* Get ruleType
* @return ruleType
**/
@Schema(description = "")
public String getRuleType() {
return ruleType;
}
public void setRuleType(String ruleType) {
this.ruleType = ruleType;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccessActionForUpdateCdnConfigInput accessActionForUpdateCdnConfigInput = (AccessActionForUpdateCdnConfigInput) o;
return Objects.equals(this.allowEmpty, accessActionForUpdateCdnConfigInput.allowEmpty) &&
Objects.equals(this.listRules, accessActionForUpdateCdnConfigInput.listRules) &&
Objects.equals(this.requestHeader, accessActionForUpdateCdnConfigInput.requestHeader) &&
Objects.equals(this.ruleType, accessActionForUpdateCdnConfigInput.ruleType);
}
@Override
public int hashCode() {
return Objects.hash(allowEmpty, listRules, requestHeader, ruleType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AccessActionForUpdateCdnConfigInput {\n");
sb.append(" allowEmpty: ").append(toIndentedString(allowEmpty)).append("\n");
sb.append(" listRules: ").append(toIndentedString(listRules)).append("\n");
sb.append(" requestHeader: ").append(toIndentedString(requestHeader)).append("\n");
sb.append(" ruleType: ").append(toIndentedString(ruleType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy