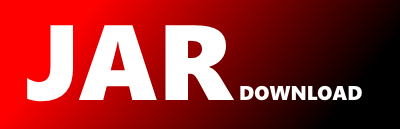
com.volcengine.cdn.model.BandwidthLimitActionForDescribeCdnConfigOutput Maven / Gradle / Ivy
/*
* cdn
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: common-version
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.volcengine.cdn.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.v3.oas.annotations.media.Schema;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.Valid;
/**
* BandwidthLimitActionForDescribeCdnConfigOutput
*/
public class BandwidthLimitActionForDescribeCdnConfigOutput {
@SerializedName("BandwidthThreshold")
private Long bandwidthThreshold = null;
@SerializedName("LimitType")
private String limitType = null;
@SerializedName("SpeedLimitRate")
private Long speedLimitRate = null;
@SerializedName("SpeedLimitRateMax")
private Long speedLimitRateMax = null;
public BandwidthLimitActionForDescribeCdnConfigOutput bandwidthThreshold(Long bandwidthThreshold) {
this.bandwidthThreshold = bandwidthThreshold;
return this;
}
/**
* Get bandwidthThreshold
* @return bandwidthThreshold
**/
@Schema(description = "")
public Long getBandwidthThreshold() {
return bandwidthThreshold;
}
public void setBandwidthThreshold(Long bandwidthThreshold) {
this.bandwidthThreshold = bandwidthThreshold;
}
public BandwidthLimitActionForDescribeCdnConfigOutput limitType(String limitType) {
this.limitType = limitType;
return this;
}
/**
* Get limitType
* @return limitType
**/
@Schema(description = "")
public String getLimitType() {
return limitType;
}
public void setLimitType(String limitType) {
this.limitType = limitType;
}
public BandwidthLimitActionForDescribeCdnConfigOutput speedLimitRate(Long speedLimitRate) {
this.speedLimitRate = speedLimitRate;
return this;
}
/**
* Get speedLimitRate
* @return speedLimitRate
**/
@Schema(description = "")
public Long getSpeedLimitRate() {
return speedLimitRate;
}
public void setSpeedLimitRate(Long speedLimitRate) {
this.speedLimitRate = speedLimitRate;
}
public BandwidthLimitActionForDescribeCdnConfigOutput speedLimitRateMax(Long speedLimitRateMax) {
this.speedLimitRateMax = speedLimitRateMax;
return this;
}
/**
* Get speedLimitRateMax
* @return speedLimitRateMax
**/
@Schema(description = "")
public Long getSpeedLimitRateMax() {
return speedLimitRateMax;
}
public void setSpeedLimitRateMax(Long speedLimitRateMax) {
this.speedLimitRateMax = speedLimitRateMax;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BandwidthLimitActionForDescribeCdnConfigOutput bandwidthLimitActionForDescribeCdnConfigOutput = (BandwidthLimitActionForDescribeCdnConfigOutput) o;
return Objects.equals(this.bandwidthThreshold, bandwidthLimitActionForDescribeCdnConfigOutput.bandwidthThreshold) &&
Objects.equals(this.limitType, bandwidthLimitActionForDescribeCdnConfigOutput.limitType) &&
Objects.equals(this.speedLimitRate, bandwidthLimitActionForDescribeCdnConfigOutput.speedLimitRate) &&
Objects.equals(this.speedLimitRateMax, bandwidthLimitActionForDescribeCdnConfigOutput.speedLimitRateMax);
}
@Override
public int hashCode() {
return Objects.hash(bandwidthThreshold, limitType, speedLimitRate, speedLimitRateMax);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BandwidthLimitActionForDescribeCdnConfigOutput {\n");
sb.append(" bandwidthThreshold: ").append(toIndentedString(bandwidthThreshold)).append("\n");
sb.append(" limitType: ").append(toIndentedString(limitType)).append("\n");
sb.append(" speedLimitRate: ").append(toIndentedString(speedLimitRate)).append("\n");
sb.append(" speedLimitRateMax: ").append(toIndentedString(speedLimitRateMax)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy